mn create-app example.micronaut.micronautguide --feature=aws-lambda --build=maven --lang=java
Deploy a Micronaut application to AWS Lambda Java 11 Runtime
Learn how to distribute a Micronaut application to AWS Lambda 11 Runtime
Authors: Sergio del Amo
Micronaut Version: 2.5.0
1. Getting Started
In this guide we are going to create a Micronaut app written in Java.
2. What you will need
To complete this guide, you will need the following:
-
Some time on your hands
-
A decent text editor or IDE
-
JDK 1.8 or greater installed with
JAVA_HOME
configured appropriately
3. Solution
We recommend that you follow the instructions in the next sections and create the app step by step. However, you can go right to the completed example.
-
Download and unzip the source
4. Writing the App
Create an app using the Micronaut Command Line Interface or with Micronaut Launch.
If you don’t specify the --build argument, Gradle is used as a build tool. If you don’t specify the --lang argument, Java is used as a language.
|
If you use Micronaut Launch, add aws-lambda
feature.
The previous command creates a Micronaut app with the default package example.micronaut
in a folder named micronautguide
.
If you are using Java or Kotlin and IntelliJ IDEA, make sure you have enabled annotation processing.
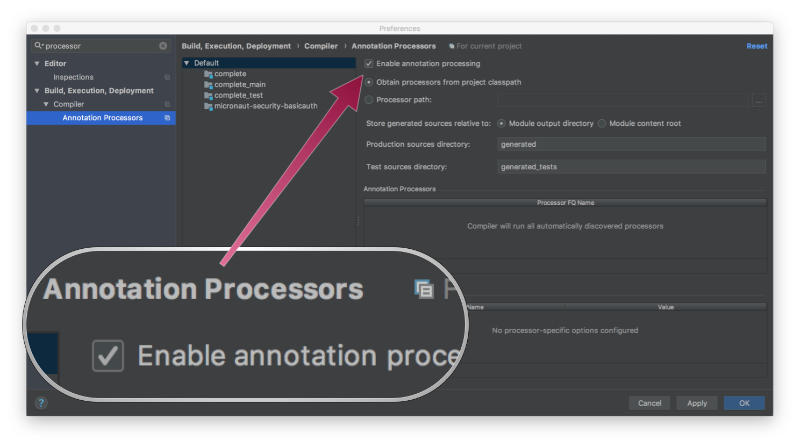
The generated application contains a BookController
. It responds to POST request to /
.
package example.micronaut;
import io.micronaut.http.annotation.Body;
import io.micronaut.http.annotation.Controller;
import io.micronaut.http.annotation.Post;
import javax.validation.Valid;
import java.util.UUID;
@Controller
public class BookController {
@Post
public BookSaved save(@Valid @Body Book book) {
BookSaved bookSaved = new BookSaved();
bookSaved.setName(book.getName());
bookSaved.setIsbn(UUID.randomUUID().toString());
return bookSaved;
}
}
-
The class is defined as a controller with the
@Controller
annotation mapped to the path / -
The
@Post
annotation is used to map HTTP request to/
to the thesave
method. -
Add the
@Valid
annotation to any method parameter’s object which requires validation.
The controller’s method parameter is a Book
object:
package example.micronaut;
import io.micronaut.core.annotation.NonNull;
import io.micronaut.core.annotation.Introspected;
import javax.validation.constraints.NotBlank;
@Introspected
public class Book {
@NonNull
@NotBlank
private String name;
public Book() {
}
@NonNull
public String getName() {
return name;
}
public void setName(@NonNull String name) {
this.name = name;
}
}
-
Annotate the class with
@Introspected
to generate the Bean Metainformation at compile time.
It returns a BookSaved
object:
package example.micronaut;
import io.micronaut.core.annotation.NonNull;
import io.micronaut.core.annotation.Introspected;
import javax.validation.constraints.NotBlank;
@Introspected
public class BookSaved {
@NonNull
@NotBlank
private String name;
@NonNull
@NotBlank
private String isbn;
public BookSaved() {
}
@NonNull
public String getName() {
return name;
}
public void setName(@NonNull String name) {
this.name = name;
}
@NonNull
public String getIsbn() {
return isbn;
}
public void setIsbn(@NonNull String isbn) {
this.isbn = isbn;
}
}
-
Annotate the class with
@Introspected
to generate the Bean Metainformation at compile time.
The generated tests illustrates how the code works when the lambda gets invoked:
package example.micronaut;
import com.amazonaws.serverless.exceptions.ContainerInitializationException;
import com.amazonaws.serverless.proxy.internal.testutils.AwsProxyRequestBuilder;
import com.amazonaws.serverless.proxy.internal.testutils.MockLambdaContext;
import com.amazonaws.serverless.proxy.model.AwsProxyRequest;
import com.amazonaws.serverless.proxy.model.AwsProxyResponse;
import com.amazonaws.services.lambda.runtime.Context;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.ObjectMapper;
import io.micronaut.http.HttpHeaders;
import io.micronaut.http.HttpMethod;
import io.micronaut.http.HttpStatus;
import io.micronaut.http.MediaType;
import org.junit.jupiter.api.AfterAll;
import org.junit.jupiter.api.Assertions;
import org.junit.jupiter.api.BeforeAll;
import org.junit.jupiter.api.Test;
import io.micronaut.function.aws.proxy.MicronautLambdaHandler;
public class BookControllerTest {
private static MicronautLambdaHandler handler;
private static Context lambdaContext = new MockLambdaContext();
private static ObjectMapper objectMapper;
@BeforeAll
public static void setupSpec() {
try {
handler = new MicronautLambdaHandler();
objectMapper = handler.getApplicationContext().getBean(ObjectMapper.class);
} catch (ContainerInitializationException e) {
e.printStackTrace();
}
}
@AfterAll
public static void cleanupSpec() {
handler.getApplicationContext().close();
}
@Test
void testSaveBook() throws JsonProcessingException {
Book book = new Book();
book.setName("Building Microservices");
String json = objectMapper.writeValueAsString(book);
AwsProxyRequest request = new AwsProxyRequestBuilder("/", HttpMethod.POST.toString())
.header(HttpHeaders.CONTENT_TYPE, MediaType.APPLICATION_JSON)
.body(json)
.build();
AwsProxyResponse response = handler.handleRequest(request, lambdaContext);
Assertions.assertEquals(HttpStatus.OK.getCode(), response.getStatusCode());
BookSaved bookSaved = objectMapper.readValue(response.getBody(), BookSaved.class);
Assertions.assertEquals(bookSaved.getName(), book.getName());
Assertions.assertNotNull(bookSaved.getIsbn());
}
}
-
When you instantiate the Handler, the application context starts.
-
Remember to close your application context when you end your test. You can use your handler to obtain it.
-
You don’t invoke the controller directly. Instead, your handler receives a AWS Proxy Request event which it is routed transparently to your controller.
5. Testing the Application
To run the tests:
$ ./mvnw test
6. Lambda
Create a Lambda Function. As a runtime, select Java 11 (Correto).
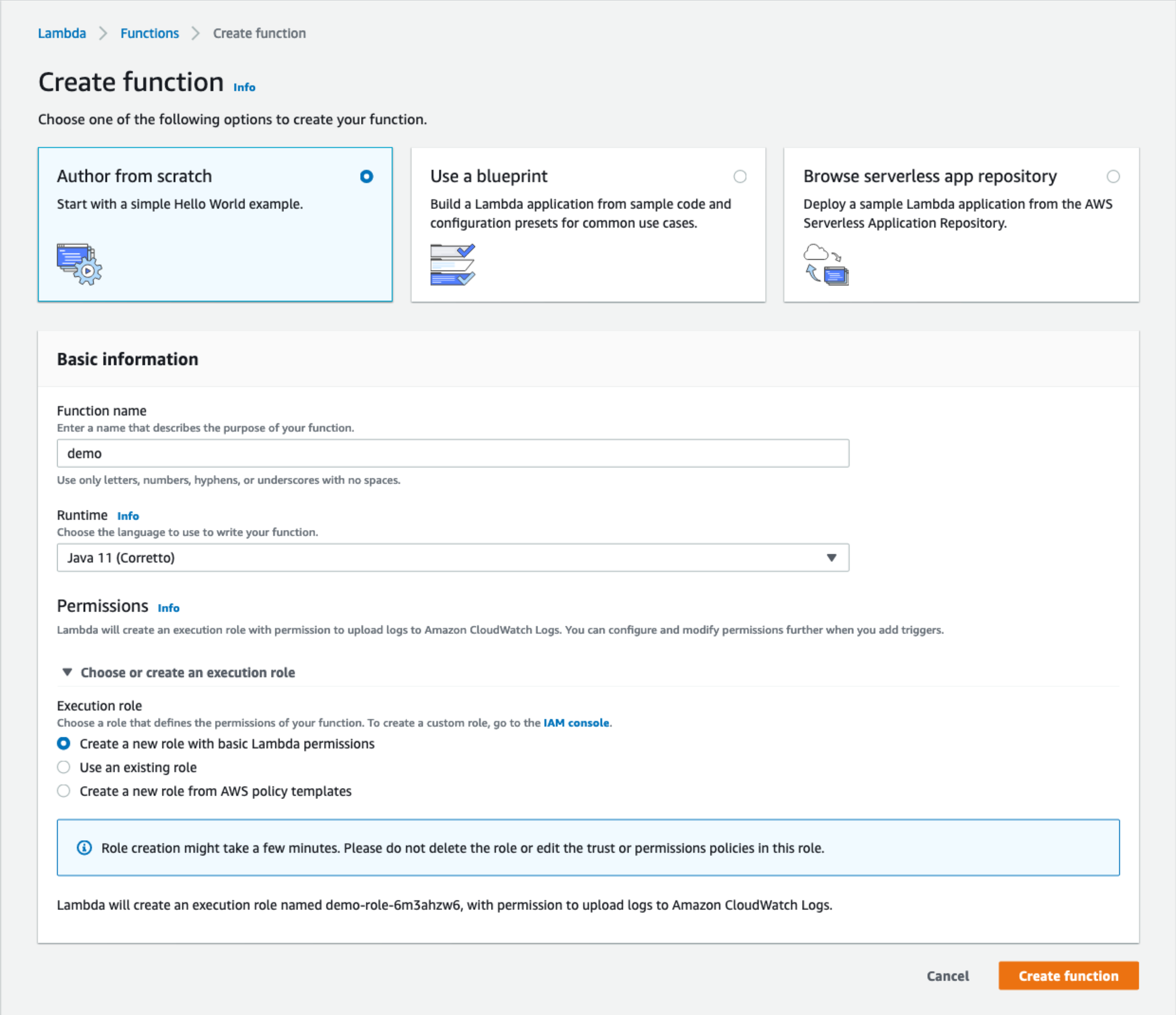
6.1. Upload Code
Create an executable jar including all dependencies:
$ ./mvnw package
Upload it:

6.2. Handler
As Handler, set:
io.micronaut.function.aws.proxy.MicronautLambdaHandler
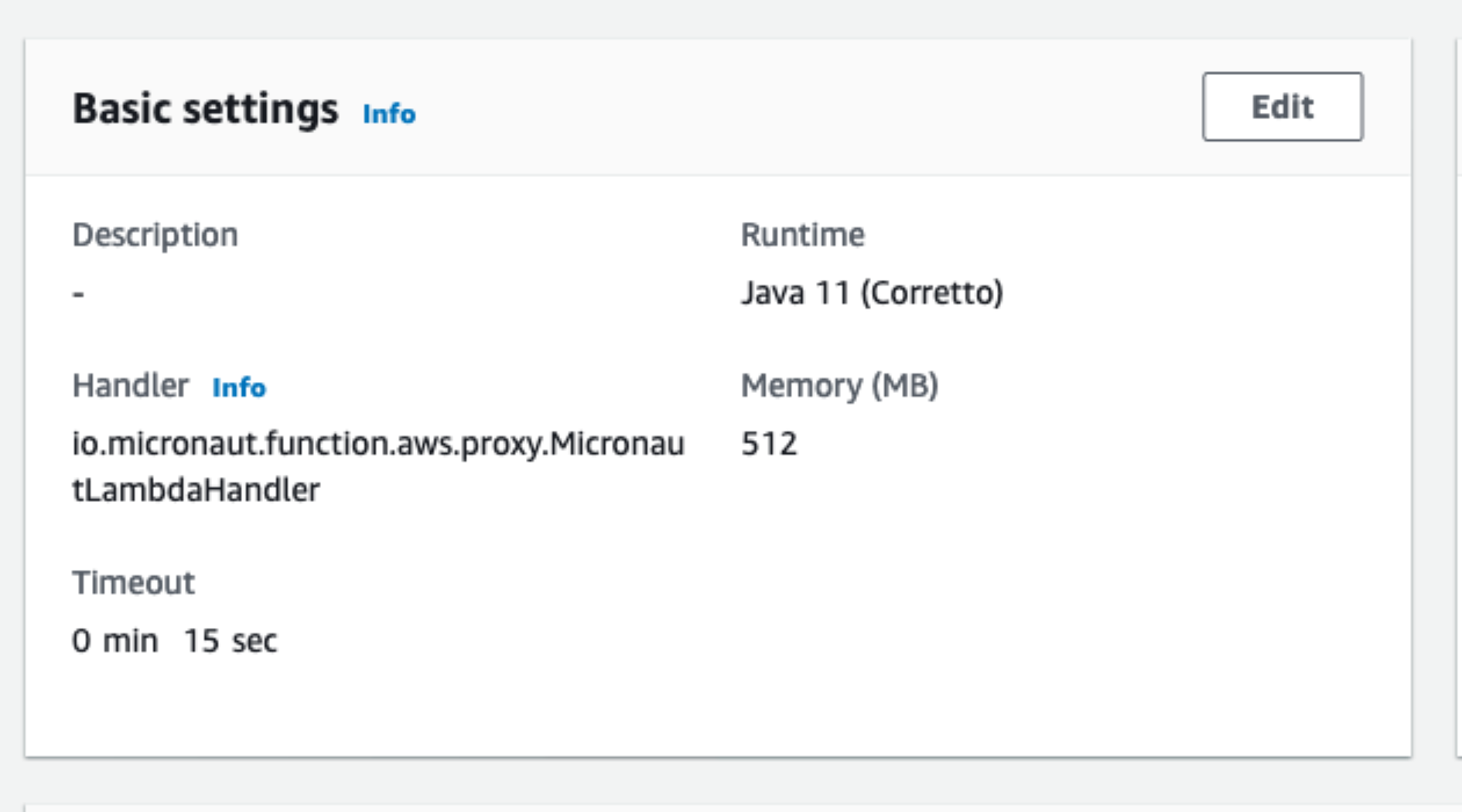
6.3. Test
You can test it easily. As Event Template
use apigateway-aws-proxy
to get you started:
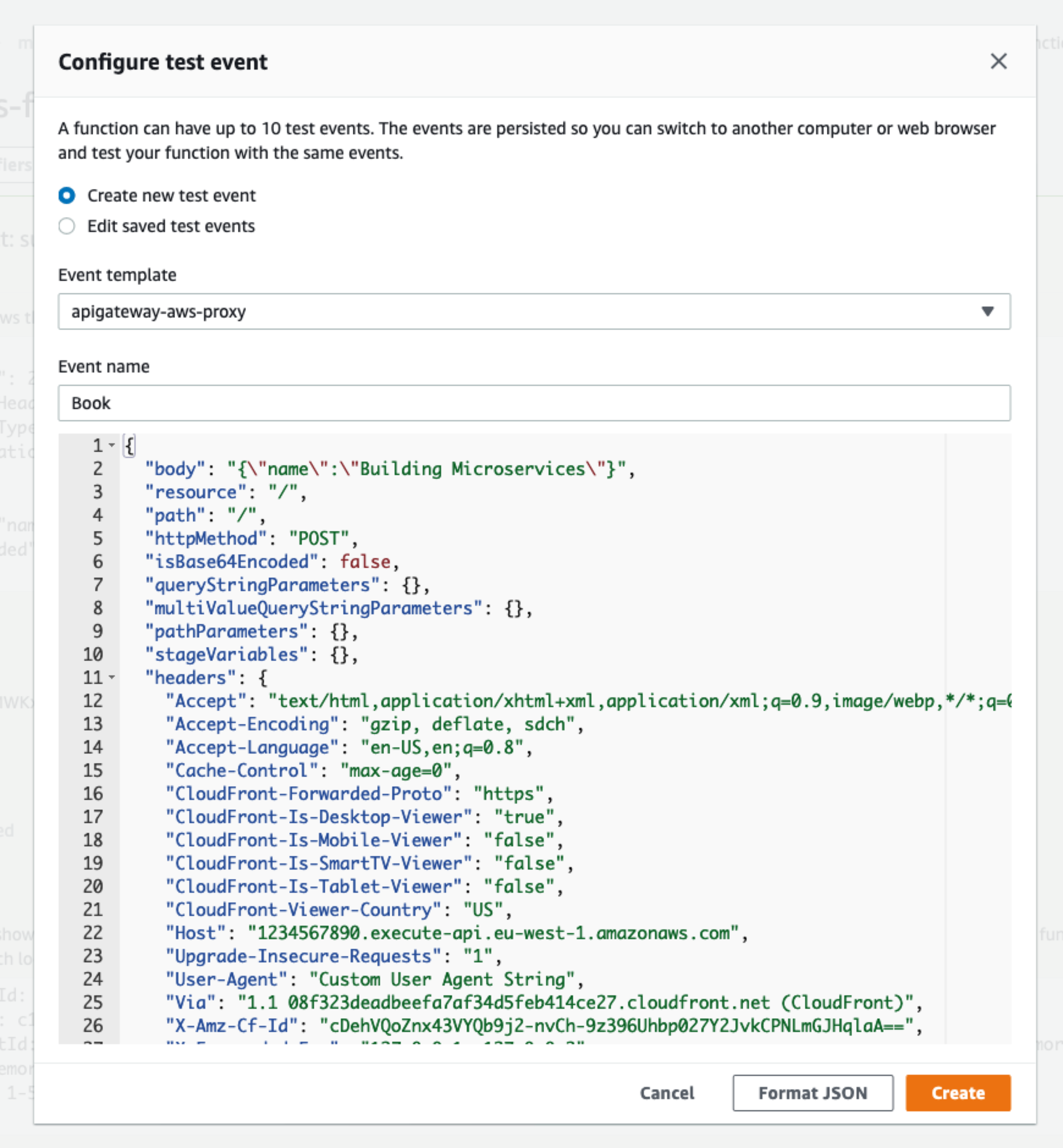
{
"body": "{\"name\": \"Building Microservices\"}",
"resource": "/",
"path": "/",
"httpMethod": "POST",
"isBase64Encoded": false,
"queryStringParameters": {},
"multiValueQueryStringParameters": {},
"pathParameters": {},
"stageVariables": {},
...
}
You should see a 200 response:
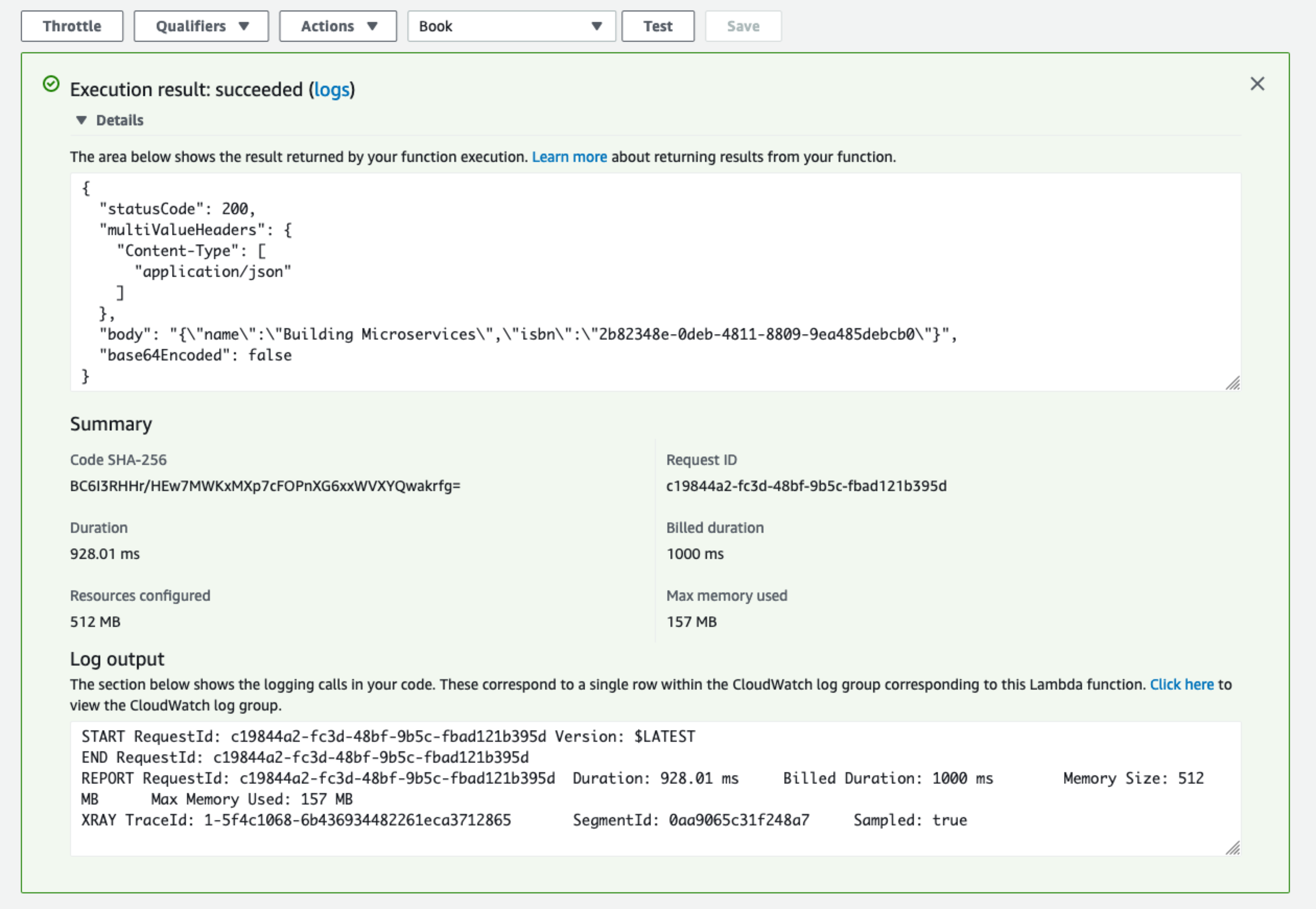
7. Next steps
Explore more features with Micronaut Guides.
Read more about:
8. Help with Micronaut
Object Computing, Inc. (OCI) sponsored the creation of this Guide. A variety of consulting and support services are available.