Session based authentication
Learn how to secure a Micronaut app using Session based authentication.
Authors: Sergio del Amo
Micronaut Version: 2.5.0
1. Getting Started
In this guide you are going to write a Micronaut app with session based authentication.
The following sequence illustrates the authentication flow:
2. What you will need
To complete this guide, you will need the following:
-
Some time on your hands
-
A decent text editor or IDE
-
JDK 1.8 or greater installed with
JAVA_HOME
configured appropriately
3. Solution
We recommend that you follow the instructions in the next sections and create the app step by step. However, you can go right to the completed example.
-
Download and unzip the source
4. Writing the Application
Create an app using the Micronaut Command Line Interface.
mn create-app example.micronaut.micronautguide --test=spock --lang=java
The previous command creates a micronaut app with the default package example.micronaut
in a folder named micronautguide
.
If you are using Java or Kotlin and IntelliJ IDEA, make sure you have enabled annotation processing.
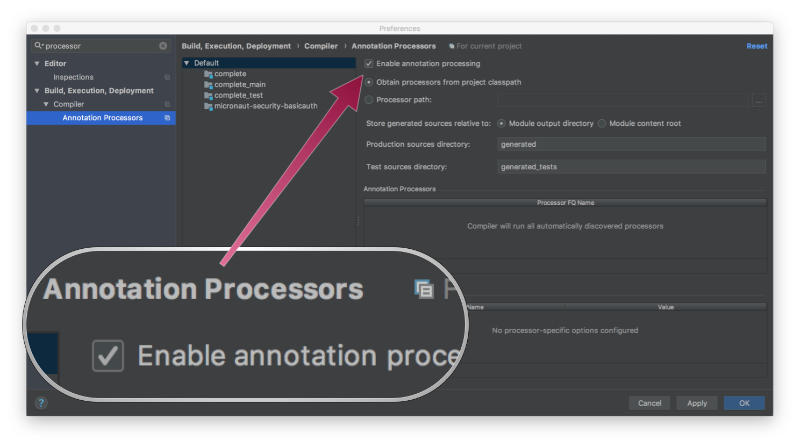
4.1. Security Dependency
Add Micronaut’s security dependency to your build file.
Add Micronaut’s JWT security dependency.
<!-- Add the following to your annotationProcessorPaths element -->
<path>
<groupId>io.micronaut.security</groupId>
<artifactId>micronaut-security-annotations</artifactId>
</path>
<dependency>
<groupId>io.micronaut.security</groupId>
<artifactId>micronaut-security-session</artifactId>
<scope>compile</scope>
</dependency>
4.2. Configuration
Create the next configuration file:
micronaut:
security:
authentication: session (1)
redirect:
login-success: / (2)
login-failure: /login/authFailed (3)
1 | Set micronaut.security.authentication to session . It sets the necessary beans for login and logout using session based authentication. |
2 | After the user logs in, redirect them to the Home page. |
3 | If the login fails, redirect them to /login/authFailed |
4.3. Authentication Provider
To keep this guide simple, create a naive AuthenticationProvider
to simulate user’s authentication.
package example.micronaut;
import io.micronaut.core.annotation.Nullable;
import io.micronaut.http.HttpRequest;
import io.micronaut.security.authentication.AuthenticationException;
import io.micronaut.security.authentication.AuthenticationFailed;
import io.micronaut.security.authentication.AuthenticationProvider;
import io.micronaut.security.authentication.AuthenticationRequest;
import io.micronaut.security.authentication.AuthenticationResponse;
import io.micronaut.security.authentication.UserDetails;
import io.reactivex.BackpressureStrategy;
import io.reactivex.Flowable;
import org.reactivestreams.Publisher;
import javax.inject.Singleton;
import java.util.ArrayList;
@Singleton (1)
public class AuthenticationProviderUserPassword implements AuthenticationProvider { (2)
@Override
public Publisher<AuthenticationResponse> authenticate(@Nullable HttpRequest<?> httpRequest, AuthenticationRequest<?, ?> authenticationRequest) {
return Flowable.create(emitter -> {
if (authenticationRequest.getIdentity().equals("sherlock") &&
authenticationRequest.getSecret().equals("password")) {
UserDetails userDetails = new UserDetails((String) authenticationRequest.getIdentity(), new ArrayList<>());
emitter.onNext(userDetails);
emitter.onComplete();
} else {
emitter.onError(new AuthenticationException(new AuthenticationFailed()));
}
}, BackpressureStrategy.ERROR);
}
}
1 | To register a Singleton in Micronaut’s application context annotate your class with javax.inject.Singleton |
2 | A Micronaut’s Authentication Provider implements the interface io.micronaut.security.authentication.AuthenticationProvider |
4.4. Apache Velocity
By default, Micronaut’s controllers produce JSON. Usually, you consume those endpoints with a mobile phone application, or a Javascript front end (Angular, React, Vue.js …). However, to keep this guide simple we are going to produce HTML in our controllers.
In order to do that, we use Apache Velocity.
Velocity is a Java-based template engine. It permits anyone to use a simple yet powerful template language to reference objects defined in Java code.
Add a dependency to Micronaut’s Server Side View Rendering Module and to Velocity:
<dependency>
<groupId>io.micronaut.views</groupId>
<artifactId>micronaut-views-velocity</artifactId>
<scope>compile</scope>
</dependency>
Create two velocity templates in src/main/resources/views
:
<!DOCTYPE html>
<html>
<head>
<title>Home</title>
</head>
<body>
#if( $loggedIn )
<h1>username: <span>$username</span></h1>
#else
<h1>You are not logged in</h1>
#end
#if( $loggedIn )
<form action="logout" method="POST">
<input type="submit" value="Logout"/>
</form>
#else
<p><a href="/login/auth">Login</a></p>
#end
</body>
</html>
<!DOCTYPE html>
<html>
<head>
#if( $errors )
<title>Login Failed</title>
#else
<title>Login</title>
#end
</head>
<body>
<form action="/login" method="POST">
<ol>
<li>
<label for="username">Username</label>
<input type="text" name="username" id="username"/>
</li>
<li>
<label for="password">Password</label>
<input type="password" name="password" id="password"/>
</li>
<li>
<input type="submit" value="Login"/>
</li>
#if( $errors )
<li id="errors">
<span style="color: red;">Login Failed</span>
</li>
#end
</ol>
</form>
</body>
</html>
4.5. Controllers
Create a file named HomeController
which resolves the base URL /
:
package example.micronaut;
import io.micronaut.core.annotation.Nullable;
import io.micronaut.http.MediaType;
import io.micronaut.http.annotation.Controller;
import io.micronaut.http.annotation.Get;
import io.micronaut.http.annotation.Produces;
import io.micronaut.security.annotation.Secured;
import io.micronaut.security.rules.SecurityRule;
import io.micronaut.views.View;
import java.security.Principal;
import java.util.HashMap;
import java.util.Map;
@Secured(SecurityRule.IS_ANONYMOUS) (1)
@Controller (2)
public class HomeController {
@Produces(MediaType.TEXT_HTML)
@Get (3)
@View("home") (4)
Map<String, Object> index(@Nullable Principal principal) { (5)
Map<String, Object> data = new HashMap<>();
data.put("loggedIn", principal != null);
if (principal != null) {
data.put("username", principal.getName());
}
return data;
}
}
1 | Annotate with io.micronaut.security.Secured to configure security access. Use isAnonymous() expression to allow access to authenticated and unauthenticated users. |
2 | Annotate with io.micronaut.http.annotation.Controller to designate a class as a Micronaut’s controller. |
3 | You can specify the HTTP verb for which a controller’s action responds to. To respond to a GET request, use io.micronaut.http.annotation.Get |
4 | Use View annotation to specify which template would you like to render the response against. |
5 | If you are authenticated, you can use the java.security.Principal as a parameter type. For parameters which maybe null, use io.micronaut.core.annotation.Nullable . |
5. Login Form
Next, create LoginAuthController
which renders the login form.
package example.micronaut;
import io.micronaut.http.MediaType;
import io.micronaut.http.annotation.Controller;
import io.micronaut.http.annotation.Get;
import io.micronaut.http.annotation.Produces;
import io.micronaut.security.annotation.Secured;
import io.micronaut.security.rules.SecurityRule;
import io.micronaut.views.View;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
@Secured(SecurityRule.IS_ANONYMOUS) (1)
@Controller("/login") (2)
public class LoginAuthController {
@Produces(MediaType.TEXT_HTML)
@Get("/auth") (3)
@View("auth") (4)
public Map<String, Object> auth() {
return new HashMap<>();
}
@Produces(MediaType.TEXT_HTML)
@Get("/authFailed") (5)
@View("auth") (4)
public Map<String, Object> authFailed() {
return Collections.singletonMap("errors", true);
}
}
1 | Annotate with io.micronaut.security.Secured to configure security access. Use isAnonymous() expression for anonymous access. |
2 | Annotate with io.micronaut.http.annotation.Controller to designate a class as a Micronaut’s controller. |
3 | Responds to GET requests at /login/auth |
4 | Use View annotation to specify which template would you like to render the response against. |
5 | Responds to GET requests at /login/authFailed |
6. Tests
We also use Geb, a browser automation solution.
To use Geb, add these dependencies:
<dependency>
<groupId>org.gebish</groupId>
<artifactId>geb-spock:4.0</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>htmlunit-driver:2.47.1</artifactId>
<scope>test</scope>
</dependency>
Geb uses the Page concept pattern - The Page Object Pattern gives us a common sense way to model content in a reusable and maintainable way.
Create three pages:
package example.micronaut
import geb.Page
class HomePage extends Page {
static url = '/'
static at = { title == 'Home' }
static content = {
loginLink { $('a', text: 'Login') }
logoutButton { $('input', type: 'submit', value: 'Logout') }
usernameElement(required: false) { $('h1 span', 0) }
}
String username() {
if ( usernameElement.empty ) {
return null
}
usernameElement.text()
}
void login() {
loginLink.click()
}
void logout() {
logoutButton.click()
}
}
package example.micronaut
import geb.Page
class LoginPage extends Page {
static url = '/login/auth'
static at = { title.contains 'Login' }
static content = {
usernameInput { $('#username') }
passwordInput { $('#password') }
submitInput { $('input', type: 'submit') }
errorsLi(required: false) { $('li#errors') }
}
boolean hasErrors() {
!errorsLi.empty
}
void login(String username, String password) {
usernameInput = username
passwordInput = password
submitInput.click()
}
}
package example.micronaut
import geb.Page
class LoginFailedPage extends Page {
static at = { title == 'Login Failed' }
static url = '/login/authFailed'
}
Create a test which verifies the user authentication flow.
@MicronautTest (1)
class SessionAuthenticationSpec extends GebSpec {
@Inject
EmbeddedServer embeddedServer (2)
def "verify session based authentication works"() {
given:
browser.baseUrl = "http://localhost:${embeddedServer.port}"
when:
to HomePage
then:
at HomePage
when:
HomePage homePage = browser.page HomePage
then: 'As we are not logged in, there is no username'
homePage.username() == null
when: 'click the login link'
homePage.login()
then:
at LoginPage
when: 'fill the login form, with invalid credentials'
LoginPage loginPage = browser.page LoginPage
loginPage.login('foo', 'foo')
then: 'the user is still in the login form'
at LoginPage
and: 'and error is displayed'
loginPage.hasErrors()
when: 'fill the form with wrong credentials'
loginPage.login('sherlock', 'foo')
then: 'we get redirected to the home page'
at LoginFailedPage
when: 'fill the form with valid credentials'
loginPage.login('sherlock', 'password')
then: 'we get redirected to the home page'
at HomePage
when:
homePage = browser.page HomePage
then: 'the username is populated'
homePage.username() == 'sherlock'
when: 'click the logout button'
homePage.logout()
then: 'we are in the home page'
at HomePage
when:
homePage = browser.page HomePage
then: 'but we are no longer logged in'
homePage.username() == null
}
}
1 | Annotate the class with @MicronautTest to let Micronaut starts the embedded server and inject the beans. More info: https://micronaut-projects.github.io/micronaut-test/latest/guide/index.html. |
2 | Inject the EmbeddedServer bean. |
7. Testing the Application
To run the tests:
$ ./mvnw test
8. Running the Application
To run the application use the ./mvnw mn:run
command which will start the application on port 8080.
9. Generate a Micronaut app’s Native Image with GraalVM
We are going to use GraalVM, the polyglot embeddable virtual machine, to generate a Native image of our Micronaut application.
Native images compiled with GraalVM ahead-of-time improve the startup time and reduce the memory footprint of JVM-based applications.
Use of GraalVM’s native-image tool is only supported in Java or Kotlin projects. Groovy relies heavily on
reflection which is only partially supported by GraalVM.
|
9.1. Native Image generation
The easiest way to install GraalVM is to use SDKMan.io.
# For Java 8
$ sdk install java 21.1.0.r8-grl
# For Java 11
$ sdk install java 21.1.0.r11-grl
You need to install the native-image
component which is not installed by default.
$ gu install native-image
To generate a native image using Maven run:
$ ./mvnw package -Dpackaging=native-image
The native image will be created in target/application
and can be run with ./target/application
.
10. Next steps
Explore more features with Micronaut Guides.
11. Help with Micronaut
Object Computing, Inc. (OCI) sponsored the creation of this Guide. A variety of consulting and support services are available.