Micronaut JWT Authentication
Learn how to secure a Micronaut app using JWT (JSON Web Token) Authentication.
Authors: Sergio del Amo
Micronaut Version: 2.5.0
1. Getting Started
Micronaut ships with security capabilities based on Json Web Token (JWT). JWT is an IETF standard which defines a secure way to encapsulate arbitrary data that can be sent over unsecure URL’s.
In this guide you are going to create a Micronaut app and secure it with JWT.
The following sequence illustrates the authentication flow:
2. What you will need
To complete this guide, you will need the following:
-
Some time on your hands
-
A decent text editor or IDE
-
JDK 1.8 or greater installed with
JAVA_HOME
configured appropriately
3. Solution
We recommend that you follow the instructions in the next sections and create the app step by step. However, you can go right to the completed example.
-
Download and unzip the source
4. Writing the application
4.1. Create an App with the Command Line Interface
Create an app using the Micronaut Command Line Interface.
mn create-app example.micronaut.micronautguide --build=maven --lang=java
The previous command creates a micronaut app with the default package example.micronaut
in a folder named micronautguide
.
If you are using Java or Kotlin and IntelliJ IDEA, make sure you have enabled annotation processing.
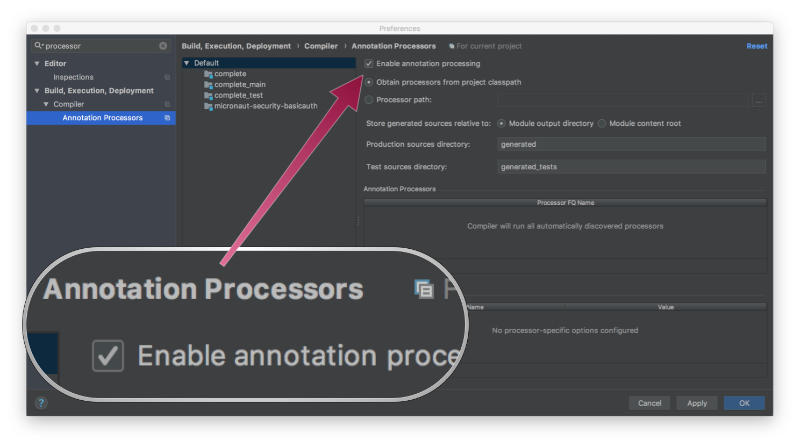
4.2. Security Dependency
Add Micronaut’s security-jwt
dependency.
<!-- Add the following to your annotationProcessorPaths element -->
<path>
<groupId>io.micronaut.security</groupId>
<artifactId>micronaut-security-annotations</artifactId>
</path>
<dependency>
<groupId>io.micronaut.security</groupId>
<artifactId>micronaut-security-jwt</artifactId>
<scope>compile</scope>
</dependency>
4.3. Configuration
Add the following to application.yml
:
micronaut:
security:
authentication: bearer (1)
token:
jwt:
signatures:
secret:
generator:
secret: '"${JWT_GENERATOR_SIGNATURE_SECRET:pleaseChangeThisSecretForANewOne}"' (2)
1 | Set authentication to bearer to receive a JSON response from the login endpoint. |
2 | Change this by your own secret and keep it safe (do not store this in your VCS). |
4.4. Authentication Provider
To keep this guide simple, create a naive AuthenticationProvider
to simulate user’s authentication.
package example.micronaut;
import io.micronaut.core.annotation.Nullable;
import io.micronaut.http.HttpRequest;
import io.micronaut.security.authentication.AuthenticationException;
import io.micronaut.security.authentication.AuthenticationFailed;
import io.micronaut.security.authentication.AuthenticationProvider;
import io.micronaut.security.authentication.AuthenticationRequest;
import io.micronaut.security.authentication.AuthenticationResponse;
import io.micronaut.security.authentication.UserDetails;
import io.reactivex.BackpressureStrategy;
import io.reactivex.Flowable;
import org.reactivestreams.Publisher;
import javax.inject.Singleton;
import java.util.ArrayList;
@Singleton (1)
public class AuthenticationProviderUserPassword implements AuthenticationProvider { (2)
@Override
public Publisher<AuthenticationResponse> authenticate(@Nullable HttpRequest<?> httpRequest, AuthenticationRequest<?, ?> authenticationRequest) {
return Flowable.create(emitter -> {
if (authenticationRequest.getIdentity().equals("sherlock") &&
authenticationRequest.getSecret().equals("password")) {
emitter.onNext(new UserDetails((String) authenticationRequest.getIdentity(), new ArrayList<>()));
emitter.onComplete();
} else {
emitter.onError(new AuthenticationException(new AuthenticationFailed()));
}
}, BackpressureStrategy.ERROR);
}
}
1 | To register a Singleton in Micronaut’s application context, annotate your class with javax.inject.Singleton |
2 | A Micronaut’s Authentication Provider implements the interface io.micronaut.security.authentication.AuthenticationProvider |
4.5. Controller
Create a file named HomeController
which resolves the base URL /
:
package example.micronaut;
import io.micronaut.http.MediaType;
import io.micronaut.http.annotation.Controller;
import io.micronaut.http.annotation.Get;
import io.micronaut.http.annotation.Produces;
import io.micronaut.security.annotation.Secured;
import io.micronaut.security.rules.SecurityRule;
import java.security.Principal;
@Secured(SecurityRule.IS_AUTHENTICATED) (1)
@Controller (2)
public class HomeController {
@Produces(MediaType.TEXT_PLAIN)
@Get (3)
public String index(Principal principal) { (4)
return principal.getName();
}
}
1 | Annotate with io.micronaut.security.Secured to configure secured access. The isAuthenticated() expression will allow access only to authenticated users. |
2 | Annotate with io.micronaut.http.annotation.Controller to designate a class as a Micronaut’s controller. |
3 | You can specify the HTTP verb that a controller’s action responds to. To respond to a GET request, use the io.micronaut.http.annotation.Get annotation. |
4 | If a user is authenticated, Micronaut will bind the user object to an argument of type java.security.Principal (if present). |
5. Tests
Create a test to verify a user is able to login and access a secured endpoint.
package example.micronaut;
import com.nimbusds.jwt.JWTParser;
import com.nimbusds.jwt.SignedJWT;
import io.micronaut.http.HttpRequest;
import io.micronaut.http.HttpResponse;
import io.micronaut.http.HttpStatus;
import io.micronaut.http.MediaType;
import io.micronaut.http.client.HttpClient;
import io.micronaut.http.client.annotation.Client;
import io.micronaut.http.client.exceptions.HttpClientResponseException;
import io.micronaut.security.authentication.UsernamePasswordCredentials;
import io.micronaut.security.token.jwt.render.BearerAccessRefreshToken;
import io.micronaut.test.extensions.junit5.annotation.MicronautTest;
import org.junit.jupiter.api.Assertions;
import org.junit.jupiter.api.Test;
import org.junit.jupiter.api.function.Executable;
import javax.inject.Inject;
import java.text.ParseException;
import static org.junit.jupiter.api.Assertions.assertEquals;
import static org.junit.jupiter.api.Assertions.assertNotNull;
import static org.junit.jupiter.api.Assertions.assertThrows;
import static org.junit.jupiter.api.Assertions.assertTrue;
@MicronautTest (1)
class JwtAuthenticationTest {
@Inject
@Client("/")
HttpClient client; (2)
@Test
void accessingASecuredUrlWithoutAuthenticatingReturnsUnauthorized() {
HttpClientResponseException e = assertThrows(HttpClientResponseException.class, () -> {
client.toBlocking().exchange(HttpRequest.GET("/").accept(MediaType.TEXT_PLAIN)); (3)
});
assertEquals(e.getStatus(), HttpStatus.UNAUTHORIZED); (3)
}
@Test
void uponSuccessfulAuthenticationAJsonWebTokenIsIssuedToTheUser() throws ParseException {
UsernamePasswordCredentials creds = new UsernamePasswordCredentials("sherlock", "password");
HttpRequest request = HttpRequest.POST("/login", creds); (4)
HttpResponse<BearerAccessRefreshToken> rsp = client.toBlocking().exchange(request, BearerAccessRefreshToken.class); (5)
assertEquals(HttpStatus.OK, rsp.getStatus());
BearerAccessRefreshToken bearerAccessRefreshToken = rsp.body();
assertEquals("sherlock", bearerAccessRefreshToken.getUsername());
assertNotNull(bearerAccessRefreshToken.getAccessToken());
assertTrue(JWTParser.parse(bearerAccessRefreshToken.getAccessToken()) instanceof SignedJWT);
String accessToken = bearerAccessRefreshToken.getAccessToken();
HttpRequest requestWithAuthorization = HttpRequest.GET("/")
.accept(MediaType.TEXT_PLAIN)
.bearerAuth(accessToken); (6)
HttpResponse<String> response = client.toBlocking().exchange(requestWithAuthorization, String.class);
assertEquals(HttpStatus.OK, rsp.getStatus());
assertEquals("sherlock", response.body()); (7)
}
}
1 | Annotate the class with @MicronautTest to let Micronaut starts the embedded server and inject the beans. More info: https://micronaut-projects.github.io/micronaut-test/latest/guide/index.html. |
2 | Inject the HttpClient bean in the application context. |
3 | When you include the security dependencies, security is considered enabled and every endpoint is secured by default. |
4 | To login, do a POST request to /login with your credentials as a JSON payload in the body of the request. |
5 | Micronaut makes it easy to bind JSON responses into Java objects. |
6 | Micronaut supports RFC 6750 Bearer Token specification out-of-the-box. We supply the JWT token in the Authorization HTTP Header. |
7 | Use .body() to retrieve the parsed payload. |
5.1. Use Micronaut’s HTTP Client and JWT
If you want to access a secured endpoint, you can also use Micronaut’s HTTP Client and supply the JWT token in the Authorization header.
First create a @Client
with a method home
which accepts an Authorization
HTTP Header.
package example.micronaut;
import io.micronaut.http.MediaType;
import io.micronaut.http.annotation.Body;
import io.micronaut.http.annotation.Consumes;
import io.micronaut.http.annotation.Get;
import io.micronaut.http.annotation.Header;
import io.micronaut.http.annotation.Post;
import io.micronaut.http.client.annotation.Client;
import io.micronaut.security.authentication.UsernamePasswordCredentials;
import io.micronaut.security.token.jwt.render.BearerAccessRefreshToken;
@Client("/")
public interface AppClient {
@Post("/login")
BearerAccessRefreshToken login(@Body UsernamePasswordCredentials credentials);
@Consumes(MediaType.TEXT_PLAIN)
@Get("/")
String home(@Header("authorization") String authorization);
}
Create a test which uses the previous @Client
package example.micronaut;
import com.nimbusds.jwt.JWTParser;
import com.nimbusds.jwt.SignedJWT;
import io.micronaut.security.authentication.UsernamePasswordCredentials;
import io.micronaut.security.token.jwt.render.BearerAccessRefreshToken;
import io.micronaut.test.extensions.junit5.annotation.MicronautTest;
import org.junit.jupiter.api.Test;
import javax.inject.Inject;
import java.text.ParseException;
import static org.junit.jupiter.api.Assertions.assertEquals;
import static org.junit.jupiter.api.Assertions.assertNotNull;
import static org.junit.jupiter.api.Assertions.assertTrue;
@MicronautTest
class DeclarativeHttpClientWithJwtTest {
@Inject
AppClient appClient; (1)
@Test
void verifyJwtAuthenticationWorksWithDeclarativeClient() throws ParseException {
UsernamePasswordCredentials creds = new UsernamePasswordCredentials("sherlock", "password");
BearerAccessRefreshToken loginRsp = appClient.login(creds); (2)
assertNotNull(loginRsp);
assertNotNull(loginRsp.getAccessToken());
assertTrue(JWTParser.parse(loginRsp.getAccessToken()) instanceof SignedJWT);
String msg = appClient.home("Bearer " + loginRsp.getAccessToken()); (3)
assertEquals("sherlock", msg);
}
}
1 | Inject AppClient bean from application context. |
2 | To login, do a POST request to /login with your credentials as a JSON payload in the body of the request. |
3 | Supply the JWT to the HTTP Authorization header value to the @Client method. |
6. Issuing a Refresh Token
Access tokens expire. You can control the expiration with micronaut.security.token.jwt.generator.access-token-expiration
.
In addition to the access token, you can configure your login endpoint to also return a refresh token. You can use the
refresh token to obtain a new access token.
First, add the following configuration:
micronaut:
security:
token:
jwt:
generator:
refresh-token:
secret: '"${JWT_GENERATOR_SIGNATURE_SECRET:pleaseChangeThisSecretForANewOne}"' (1)
1 | To generate a refresh token your app must have beans of type:
RefreshTokenGenerator,
RefreshTokenValidator.
RefreshTokenPersistence.
We will deal with the latter in the next section. For the generator and validator, Micronaut Security ships with
SignedRefreshTokenGenerator.
It creates and verifies a JWS (JSON web signature) encoded object whose payload is a UUID with a hash-based message authentication
code (HMAC). You need to provide secret to use SignedRefreshTokenGenerator which implements both RefreshTokenGenerator and RefreshTokenValidator . |
Create a test to verify the login endpoint responds both access and refresh token:
package example.micronaut;
import com.nimbusds.jwt.JWTParser;
import com.nimbusds.jwt.SignedJWT;
import io.micronaut.http.HttpRequest;
import io.micronaut.http.client.RxHttpClient;
import io.micronaut.http.client.annotation.Client;
import io.micronaut.security.authentication.UsernamePasswordCredentials;
import io.micronaut.security.token.jwt.render.BearerAccessRefreshToken;
import io.micronaut.test.extensions.junit5.annotation.MicronautTest;
import org.junit.jupiter.api.Test;
import javax.inject.Inject;
import java.text.ParseException;
import static org.junit.jupiter.api.Assertions.assertEquals;
import static org.junit.jupiter.api.Assertions.assertNotNull;
import static org.junit.jupiter.api.Assertions.assertTrue;
@MicronautTest
public class LoginIncludesRefreshTokenTest {
@Inject
@Client("/")
RxHttpClient client;
@Test
void uponSuccessfulAuthenticationUserGetsAccessTokenAndRefreshToken() throws ParseException {
UsernamePasswordCredentials creds = new UsernamePasswordCredentials("sherlock", "password");
HttpRequest request = HttpRequest.POST("/login", creds);
BearerAccessRefreshToken rsp = client.toBlocking().retrieve(request, BearerAccessRefreshToken.class);
assertEquals("sherlock", rsp.getUsername());
assertNotNull(rsp.getAccessToken());
assertNotNull(rsp.getRefreshToken()); (1)
assertTrue(JWTParser.parse(rsp.getAccessToken()) instanceof SignedJWT);
}
}
1 | A refresh token is returned. |
6.1. Save Refresh Token
We may want to save refresh token issued by the application. For example, to be revoked a user’s refresh tokens. So that a particular user can not obtain a new access token, and thus access the application’s endpoints.
Persist the refresh tokens with help of Micronaut Data.
Micronaut Data is a database access toolkit that uses Ahead of Time (AoT) compilation to pre-compute queries for repository interfaces that are then executed by a thin, lightweight runtime layer.
In particular, use Micronaut JDBC
Micronaut Data JDBC is an implementation that pre-computes native SQL queries (given a particular database dialect) and provides a repository implementation that is a simple data mapper between a JDBC ResultSet and an object.
Add the following dependencies:
<!-- Add the following to your annotationProcessorPaths element -->
<path> (1)
<groupId>io.micronaut</groupId>
<artifactId>micronaut-data-processor</artifactId>
</path>
<dependency> (2)
<groupId>io.micronaut.data</groupId>
<artifactId>micronaut-data-jdbc</artifactId>
<scope>compile</scope>
</dependency>
<dependency> (3)
<groupId>io.micronaut.sql</groupId>
<artifactId>micronaut-jdbc-hikari</artifactId>
<scope>compile</scope>
</dependency>
<dependency> (4)
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<scope>runtime</scope>
</dependency>
1 | Micronaut data is a build time tool. You need to add the build time annotation processor |
2 | Add the Micronaut Data JDBC dependency |
3 | Add a Hikari connection pool |
4 | Add a JDBC driver. Add H2 driver |
Create an entity to save the issued Refresh Tokens.
package example.micronaut;
import io.micronaut.core.annotation.NonNull;
import io.micronaut.data.annotation.DateCreated;
import io.micronaut.data.annotation.GeneratedValue;
import io.micronaut.data.annotation.Id;
import io.micronaut.data.annotation.MappedEntity;
import javax.validation.constraints.NotBlank;
import javax.validation.constraints.NotNull;
import java.time.Instant;
@MappedEntity (1)
public class RefreshTokenEntity {
@Id (2)
@GeneratedValue (3)
@NonNull
private Long id;
@NonNull
@NotBlank
private String username;
@NonNull
@NotBlank
private String refreshToken;
@NonNull
@NotNull
private Boolean revoked;
@DateCreated (4)
@NonNull
@NotNull
private Instant dateCreated;
public RefreshTokenEntity() {
}
// getters and setters...
}
1 | Specifies the entity is mapped to the database |
2 | Specifies the ID of an entity |
3 | Specifies that the property value is generated by the database and not included in inserts |
4 | Allows assigning a data created value (such as a java.time.Instant ) prior to an insert |
Create a CrudRepository
to include methods to peform Create, Read, Updated and Delete operations with the RefreshTokenEntity
.
package example.micronaut;
import io.micronaut.core.annotation.NonNull;
import io.micronaut.data.jdbc.annotation.JdbcRepository;
import io.micronaut.data.model.query.builder.sql.Dialect;
import io.micronaut.data.repository.CrudRepository;
import javax.transaction.Transactional;
import javax.validation.constraints.NotBlank;
import javax.validation.constraints.NotNull;
import java.util.Optional;
@JdbcRepository(dialect = Dialect.H2) (1)
public interface RefreshTokenRepository extends CrudRepository<RefreshTokenEntity, Long> { (2)
@Transactional
RefreshTokenEntity save(@NonNull @NotBlank String username,
@NonNull @NotBlank String refreshToken,
@NonNull @NotNull Boolean revoked); (3)
Optional<RefreshTokenEntity> findByRefreshToken(@NonNull @NotBlank String refreshToken); (4)
long updateByUsername(@NonNull @NotBlank String username,
@NonNull @NotNull Boolean revoked); (5)
}
1 | The interface is annotated with @JdbcRepository and specifies a dialect of H2 used to generate queries |
2 | The CrudRepository interface take 2 generic arguments, the entity type (in this case RefreshTokenEntity ) and the ID type (in this case Long ) |
3 | When a new refresh token is issued we will use this method to persist it |
4 | Before issuing a new access token, we will use this method to see if the supplied refresh token exists |
5 | We can revoke the refresh tokens of a particular user with this method |
6.2. Refresh Controller
To enable the Refresh Controller, you have to enable it via configuration and provide an implementation of RefreshTokenPersistence.
To enable the refresh controller you need to create a bean of type RefreshTokenPersistence which leverages the Micronaut Data repository we coded in the previous section:
package example.micronaut;
import io.micronaut.runtime.event.annotation.EventListener;
import io.micronaut.security.authentication.UserDetails;
import io.micronaut.security.token.event.RefreshTokenGeneratedEvent;
import io.micronaut.security.token.refresh.RefreshTokenPersistence;
import io.micronaut.security.errors.OauthErrorResponseException;
import io.micronaut.security.errors.IssuingAnAccessTokenErrorCode;
import io.reactivex.BackpressureStrategy;
import io.reactivex.Flowable;
import org.reactivestreams.Publisher;
import javax.inject.Singleton;
import java.util.ArrayList;
import java.util.Optional;
@Singleton (1)
public class CustomRefreshTokenPersistence implements RefreshTokenPersistence {
private final RefreshTokenRepository refreshTokenRepository;
public CustomRefreshTokenPersistence(RefreshTokenRepository refreshTokenRepository) { (2)
this.refreshTokenRepository = refreshTokenRepository;
}
@Override
@EventListener (3)
public void persistToken(RefreshTokenGeneratedEvent event) {
if (event != null &&
event.getRefreshToken() != null &&
event.getUserDetails() != null &&
event.getUserDetails().getUsername() != null) {
String payload = event.getRefreshToken();
refreshTokenRepository.save(event.getUserDetails() .getUsername(), payload, Boolean.FALSE); (4)
}
}
@Override
public Publisher<UserDetails> getUserDetails(String refreshToken) {
return Flowable.create(emitter -> {
Optional<RefreshTokenEntity> tokenOpt = refreshTokenRepository.findByRefreshToken(refreshToken);
if (tokenOpt.isPresent()) {
RefreshTokenEntity token = tokenOpt.get();
if (token.getRevoked()) {
emitter.onError(new OauthErrorResponseException(IssuingAnAccessTokenErrorCode.INVALID_GRANT, "refresh token revoked", null)); (5)
} else {
emitter.onNext(new UserDetails(token.getUsername(), new ArrayList<>())); (6)
emitter.onComplete();
}
} else {
emitter.onError(new OauthErrorResponseException(IssuingAnAccessTokenErrorCode.INVALID_GRANT, "refresh token not found", null)); (7)
}
}, BackpressureStrategy.ERROR);
}
}
1 | To register a Singleton in Micronaut’s application context annotate your class with javax.inject.Singleton |
2 | Constructor injection of RefreshTokenRepository . |
3 | When a new refresh token is issued, the app emits an event of type RefreshTokenGeneratedEvent. We listen to it and save it in the database. |
4 | The event contains both the refresh token and the user details associated to the token. |
5 | Throw an exception if the token is revoked. |
6 | Return the user details associated to the refresh token. E.g. username, roles, attributes… |
7 | Throw an exception if the token is not found. |
6.3. Test Refresh Token
6.3.1. Test Refresh Token Validation
Refresh tokens issued by SignedRefreshTokenGenerator, the default implementation of RefreshTokenGenerator, are signed.
SignedRefreshTokenGenerator
implements both RefreshTokenGenerator
and RefreshTokenValidator.
The bean of type RefreshTokenValidator
is used by the Refresh Controller
to ensure the refresh token supplied is valid.
Create a test for this:
package example.micronaut;
import io.micronaut.core.type.Argument;
import io.micronaut.http.HttpRequest;
import io.micronaut.http.HttpStatus;
import io.micronaut.http.client.RxHttpClient;
import io.micronaut.http.client.annotation.Client;
import io.micronaut.http.client.exceptions.HttpClientResponseException;
import io.micronaut.security.token.jwt.endpoints.TokenRefreshRequest;
import io.micronaut.security.token.jwt.render.BearerAccessRefreshToken;
import io.micronaut.test.extensions.junit5.annotation.MicronautTest;
import org.junit.jupiter.api.Assertions;
import org.junit.jupiter.api.Test;
import javax.inject.Inject;
import java.util.Map;
import java.util.Optional;
import static org.junit.jupiter.api.Assertions.assertEquals;
@MicronautTest
class UnsignedRefreshTokenTest {
@Inject
@Client("/")
RxHttpClient client;
@Test
void accessingSecuredURLWithoutAuthenticatingReturnsUnauthorized() {
String unsignedRefreshedToken = "foo"; (1)
Argument<BearerAccessRefreshToken> bodyArgument = Argument.of(BearerAccessRefreshToken.class);
Argument<Map> errorArgument = Argument.of(Map.class);
HttpClientResponseException e = Assertions.assertThrows(HttpClientResponseException.class, () -> {
client.toBlocking().exchange(
HttpRequest.POST("/oauth/access_token", new TokenRefreshRequest(unsignedRefreshedToken)), bodyArgument, errorArgument
);
});
assertEquals(HttpStatus.BAD_REQUEST, e.getStatus());
Optional<Map> mapOptional = e.getResponse().getBody(Map.class);
Assertions.assertTrue(mapOptional.isPresent());
Map m = mapOptional.get();
assertEquals("invalid_grant", m.get("error"));
assertEquals("Refresh token is invalid", m.get("error_description"));
}
}
1 | Use an unsigned token |
6.3.2. Test Refresh Token Not Found
Create a test which verifies a that sending a valid refresh token but which was not persisted returns HTTP Status 400.
package example.micronaut;
import io.micronaut.core.type.Argument;
import io.micronaut.http.HttpRequest;
import io.micronaut.http.HttpStatus;
import io.micronaut.http.client.RxHttpClient;
import io.micronaut.http.client.annotation.Client;
import io.micronaut.http.client.exceptions.HttpClientResponseException;
import io.micronaut.security.authentication.UserDetails;
import io.micronaut.security.token.generator.RefreshTokenGenerator;
import io.micronaut.security.token.jwt.endpoints.TokenRefreshRequest;
import io.micronaut.security.token.jwt.render.BearerAccessRefreshToken;
import io.micronaut.test.extensions.junit5.annotation.MicronautTest;
import org.junit.jupiter.api.Test;
import javax.inject.Inject;
import java.util.Collections;
import java.util.Map;
import java.util.Optional;
import static org.junit.jupiter.api.Assertions.assertEquals;
import static org.junit.jupiter.api.Assertions.assertThrows;
import static org.junit.jupiter.api.Assertions.assertTrue;
@MicronautTest
class RefreshTokenNotFoundTest {
@Inject
@Client("/")
RxHttpClient client;
@Inject
RefreshTokenGenerator refreshTokenGenerator;
@Test
void accessingSecuredURLWithoutAuthenticatingReturnsUnauthorized() {
UserDetails user = new UserDetails("sherlock", Collections.emptyList());
String refreshToken = refreshTokenGenerator.createKey(user);
Optional<String> refreshTokenOptional = refreshTokenGenerator.generate(user, refreshToken);
assertTrue(refreshTokenOptional.isPresent());
String signedRefreshToken = refreshTokenOptional.get(); (1)
Argument<BearerAccessRefreshToken> bodyArgument = Argument.of(BearerAccessRefreshToken.class);
Argument<Map> errorArgument = Argument.of(Map.class);
HttpRequest req = HttpRequest.POST("/oauth/access_token", new TokenRefreshRequest(signedRefreshToken));
HttpClientResponseException e = assertThrows(HttpClientResponseException.class, () -> {
client.toBlocking().exchange(req, bodyArgument, errorArgument);
});
assertEquals(HttpStatus.BAD_REQUEST, e.getStatus());
Optional<Map> mapOptional = e.getResponse().getBody(Map.class);
assertTrue(mapOptional.isPresent());
Map m = mapOptional.get();
assertEquals("invalid_grant", m.get("error"));
assertEquals("refresh token not found", m.get("error_description"));
}
}
1 | Supply a signed token which was never saved. |
6.3.3. Test Refresh Token Revocation
Generate a valid refresh token, save it but flag it as revoked. Expect a 400.
package example.micronaut;
import io.micronaut.context.ApplicationContext;
import io.micronaut.core.type.Argument;
import io.micronaut.http.HttpRequest;
import io.micronaut.http.HttpStatus;
import io.micronaut.http.client.HttpClient;
import io.micronaut.http.client.exceptions.HttpClientResponseException;
import io.micronaut.runtime.server.EmbeddedServer;
import io.micronaut.security.authentication.UserDetails;
import io.micronaut.security.token.generator.RefreshTokenGenerator;
import io.micronaut.security.token.jwt.endpoints.TokenRefreshRequest;
import io.micronaut.security.token.jwt.render.BearerAccessRefreshToken;
import org.junit.jupiter.api.Test;
import java.util.Collections;
import java.util.Map;
import java.util.Optional;
import static org.junit.jupiter.api.Assertions.assertEquals;
import static org.junit.jupiter.api.Assertions.assertThrows;
import static org.junit.jupiter.api.Assertions.assertTrue;
class RefreshTokenRevokedTest {
EmbeddedServer embeddedServer = ApplicationContext.run(EmbeddedServer.class, Collections.emptyMap());
HttpClient client = embeddedServer.getApplicationContext().createBean(HttpClient.class, embeddedServer.getURL());
RefreshTokenGenerator refreshTokenGenerator = embeddedServer.getApplicationContext().getBean(RefreshTokenGenerator.class);
RefreshTokenRepository refreshTokenRepository = embeddedServer.getApplicationContext().getBean(RefreshTokenRepository.class);
@Test
void accessingSecuredURLWithoutAuthenticatingReturnsUnauthorized() {
UserDetails user = new UserDetails("sherlock", Collections.emptyList());
String refreshToken = refreshTokenGenerator.createKey(user);
Optional<String> refreshTokenOptional = refreshTokenGenerator.generate(user, refreshToken);
assertTrue(refreshTokenOptional.isPresent());
long oldTokenCount = refreshTokenRepository.count();
String signedRefreshToken = refreshTokenOptional.get();
refreshTokenRepository.save(user.getUsername(), refreshToken, Boolean.TRUE); (1)
assertEquals(oldTokenCount + 1, refreshTokenRepository.count());
Argument<BearerAccessRefreshToken> bodyArgument = Argument.of(BearerAccessRefreshToken.class);
Argument<Map> errorArgument = Argument.of(Map.class);
HttpClientResponseException e = assertThrows(HttpClientResponseException.class, () -> {
client.toBlocking().exchange(
HttpRequest.POST("/oauth/access_token", new TokenRefreshRequest(signedRefreshToken)), bodyArgument, errorArgument
);
});
assertEquals(HttpStatus.BAD_REQUEST, e.getStatus());
Optional<Map> mapOptional = e.getResponse().getBody(Map.class);
assertTrue(mapOptional.isPresent());
Map m = mapOptional.get();
assertEquals("invalid_grant", m.get("error"));
assertEquals("refresh token revoked", m.get("error_description"));
refreshTokenRepository.deleteAll();
}
}
1 | Save the token but flag it as revoked |
6.3.4. Test Access Token Refresh
Login, obtain both access token and refresh token, with the refresh token obtain a different access token:
package example.micronaut;
import io.micronaut.http.HttpRequest;
import io.micronaut.http.client.RxHttpClient;
import io.micronaut.http.client.annotation.Client;
import io.micronaut.security.authentication.UsernamePasswordCredentials;
import io.micronaut.security.token.jwt.endpoints.TokenRefreshRequest;
import io.micronaut.security.token.jwt.render.AccessRefreshToken;
import io.micronaut.security.token.jwt.render.BearerAccessRefreshToken;
import io.micronaut.test.extensions.junit5.annotation.MicronautTest;
import org.junit.jupiter.api.Test;
import javax.inject.Inject;
import static org.junit.jupiter.api.Assertions.assertEquals;
import static org.junit.jupiter.api.Assertions.assertNotEquals;
import static org.junit.jupiter.api.Assertions.assertNotNull;
@MicronautTest(rollback = false)
class OauthAccessTokenTest {
@Inject
@Client("/")
RxHttpClient client;
@Inject
RefreshTokenRepository refreshTokenRepository;
@Test
void verifyJWTAccessTokenRefreshWorks() throws InterruptedException {
String username = "sherlock";
UsernamePasswordCredentials creds = new UsernamePasswordCredentials(username, "password");
HttpRequest request = HttpRequest.POST("/login", creds);
long oldTokenCount = refreshTokenRepository.count();
BearerAccessRefreshToken rsp = client.toBlocking().retrieve(request, BearerAccessRefreshToken.class);
Thread.sleep(3_000);
assertEquals(oldTokenCount + 1, refreshTokenRepository.count());
assertNotNull(rsp.getAccessToken());
assertNotNull(rsp.getRefreshToken());
Thread.sleep(1_000); // sleep for one second to give time for the issued at `iat` Claim to change
AccessRefreshToken refreshResponse = client.toBlocking().retrieve(HttpRequest.POST("/oauth/access_token",
new TokenRefreshRequest(rsp.getRefreshToken())), AccessRefreshToken.class); (1)
assertNotNull(refreshResponse.getAccessToken());
assertNotEquals(rsp.getAccessToken(), refreshResponse.getAccessToken()); (2)
refreshTokenRepository.deleteAll();
}
}
1 | Make a POST request to /oauth/access_token with the refresh token in the JSON payload to get a new access token |
2 | A different access token is retrieved. |
7. Testing the Application
To run the tests:
$ ./mvnw test
8. Running the Application
To run the application use the ./mvnw mn:run
command which will start the application on port 8080.
Send a request to the login endpoint:
$ curl -X "POST" "http://localhost:8080/login" -H 'Content-Type: application/json' -d $'{"username": "sherlock","password": "password"}'
{"username":"sherlock","access_token":"eyJhbGciOiJIUzI1NiJ9.eyJzdWIiOiJzaGVybG9jayIsIm5iZiI6MTYxNDc2NDEzNywicm9sZXMiOltdLCJpc3MiOiJjb21wbGV0ZSIsImV4cCI6MTYxNDc2NzczNywiaWF0IjoxNjE0NzY0MTM3fQ.cn8bOjlccFqeUQA7x7MnfacMNPjSVAtWP65z1c8eaJc","refresh_token":"eyJhbGciOiJIUzI1NiJ9.NDI1ZjAxZTktYTRmYS00MmU5LTllYjctOWU2ZTNhNTI5YmQ1.RUc2iCfZdPQdwg2U0Nw_LLzZQIIDp5_Is2UWeHVZT7E","token_type":"Bearer","expires_in":3600}
9. Generate a Micronaut app’s Native Image with GraalVM
We are going to use GraalVM, the polyglot embeddable virtual machine, to generate a Native image of our Micronaut application.
Native images compiled with GraalVM ahead-of-time improve the startup time and reduce the memory footprint of JVM-based applications.
Use of GraalVM’s native-image tool is only supported in Java or Kotlin projects. Groovy relies heavily on
reflection which is only partially supported by GraalVM.
|
9.1. Native Image generation
The easiest way to install GraalVM is to use SDKMan.io.
# For Java 8
$ sdk install java 21.1.0.r8-grl
# For Java 11
$ sdk install java 21.1.0.r11-grl
You need to install the native-image
component which is not installed by default.
$ gu install native-image
To generate a native image using Maven run:
$ ./mvnw package -Dpackaging=native-image
The native image will be created in target/application
and can be run with ./target/application
.
Send the same curl
request as before to test that the native image application works.
10. Next steps
Learn more about JWT Authentication in the official documentation.
11. Help with Micronaut
Object Computing, Inc. (OCI) sponsored the creation of this Guide. A variety of consulting and support services are available.