mn create-app example.micronaut.micronautguide --build=gradle --lang=java
Secure a Micronaut app with Cognito
Learn how to create Micronaut app and secure it with an Authorization Server provided by Cognito.
Authors: Sergio del Amo
Micronaut Version: 2.5.0
1. Getting Started
In this guide we are going to create a Micronaut app written in Java.
2. What you will need
To complete this guide, you will need the following:
-
Some time on your hands
-
A decent text editor or IDE
-
JDK 1.8 or greater installed with
JAVA_HOME
configured appropriately
3. Solution
We recommend that you follow the instructions in the next sections and create the app step by step. However, you can go right to the completed example.
-
Download and unzip the source
4. Writing the App
Create an app using the Micronaut Command Line Interface or with Micronaut Launch.
If you don’t specify the --build argument, Gradle is used as a build tool. If you don’t specify the --lang argument, Java is used as a language.
|
The previous command creates a Micronaut app with the default package example.micronaut
in a folder named micronautguide
.
If you are using Java or Kotlin and IntelliJ IDEA, make sure you have enabled annotation processing.
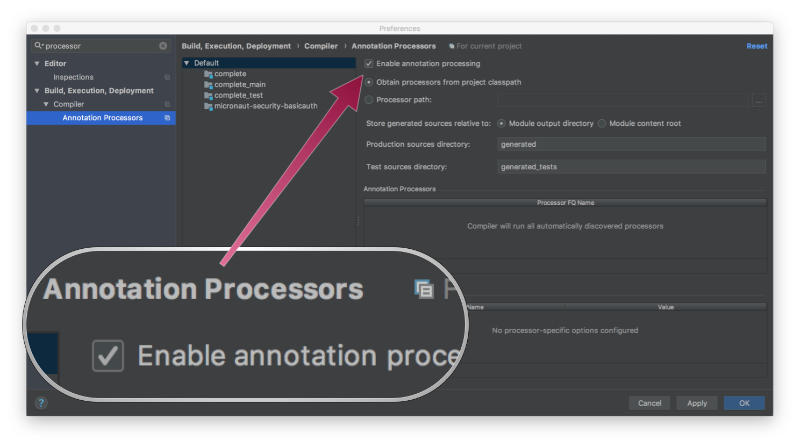
4.1. Views
Although Micronaut is primarily designed around message encoding / decoding, there are occasions where it is convenient to render a view on the server side.
To use Thymeleaf Java template engine to render views in a Micronaut application add the following dependency on your classpath.
implementation("io.micronaut.views:micronaut-views-thymeleaf")
4.2. OAuth 2.0
To provide authentication, sign in to your AWS account and go to AWS Cognito.
With the Amazon Cognito SDK, you just write a few lines of code to enable your users to sign-up and sign-in to your mobile and web apps.
Standards-based authentication
Amazon Cognito uses common identity management standards including OpenID Connect, OAuth 2.0, and SAML 2.0.
First, create an Amazon Cognito User Pool:
Amazon Cognito User Pools - A directory for all your users
You can quickly create your own directory to sign up and sign in users, and to store user profiles using Amazon Cognito User Pools. User Pools provide a user interface you can customize to match your app. User Pools also enable easy integration with social identity providers such as Facebook, Google, and Amazon, and enterprise identity providers such as Microsoft Active Directory through SAML.

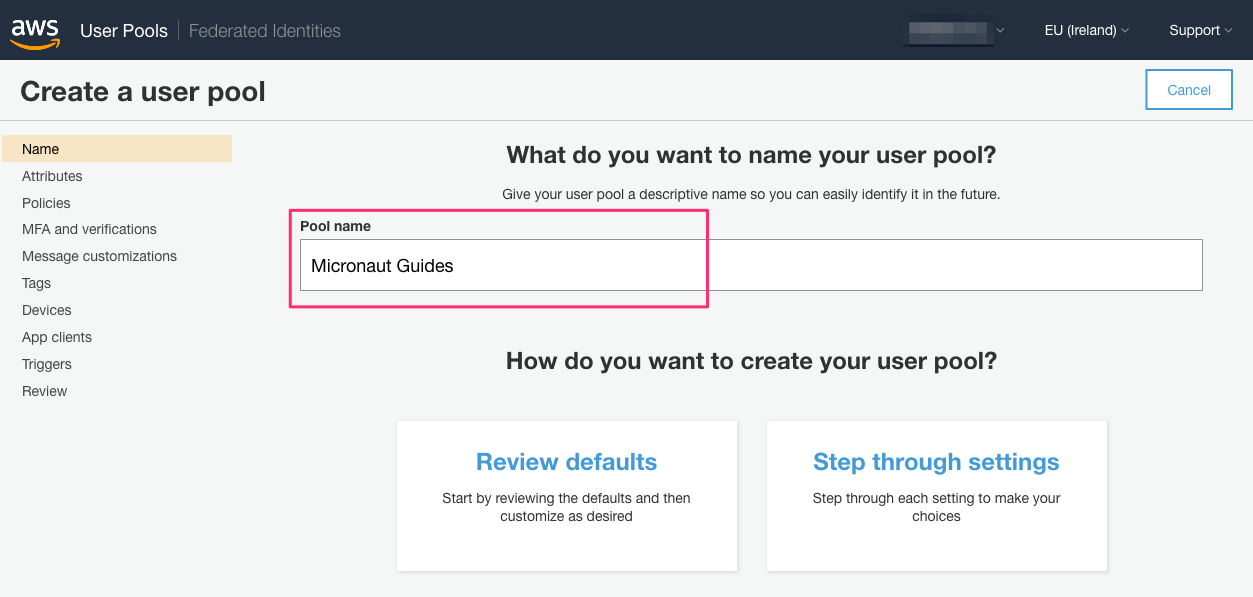
Amazon Cognito Pools are regional. Thus, annotate the region you are using.
While you setup the Amazon Cognito User Pool, you will need to save the pool id:
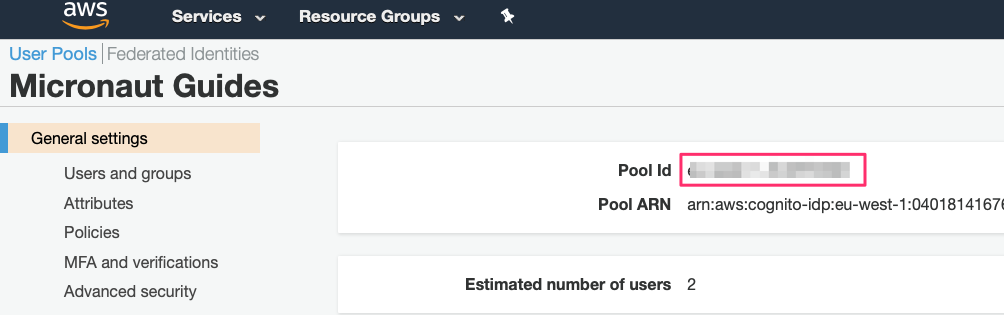
Configure the appropriate callback, make sure you allow Authorization code grant
OAuth Flow, and also the scopes openid
and email
are selected.
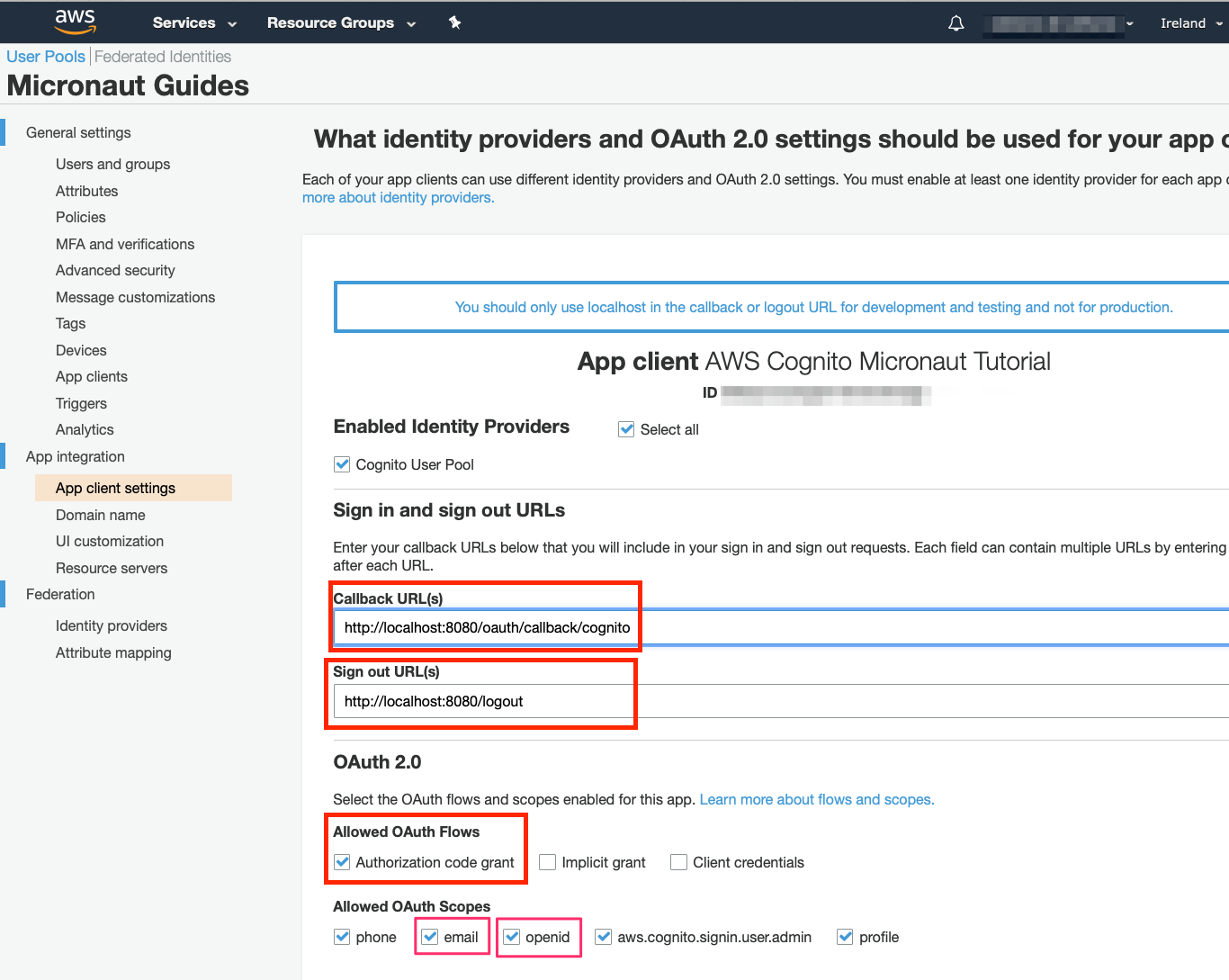
Save the client id and client secret:
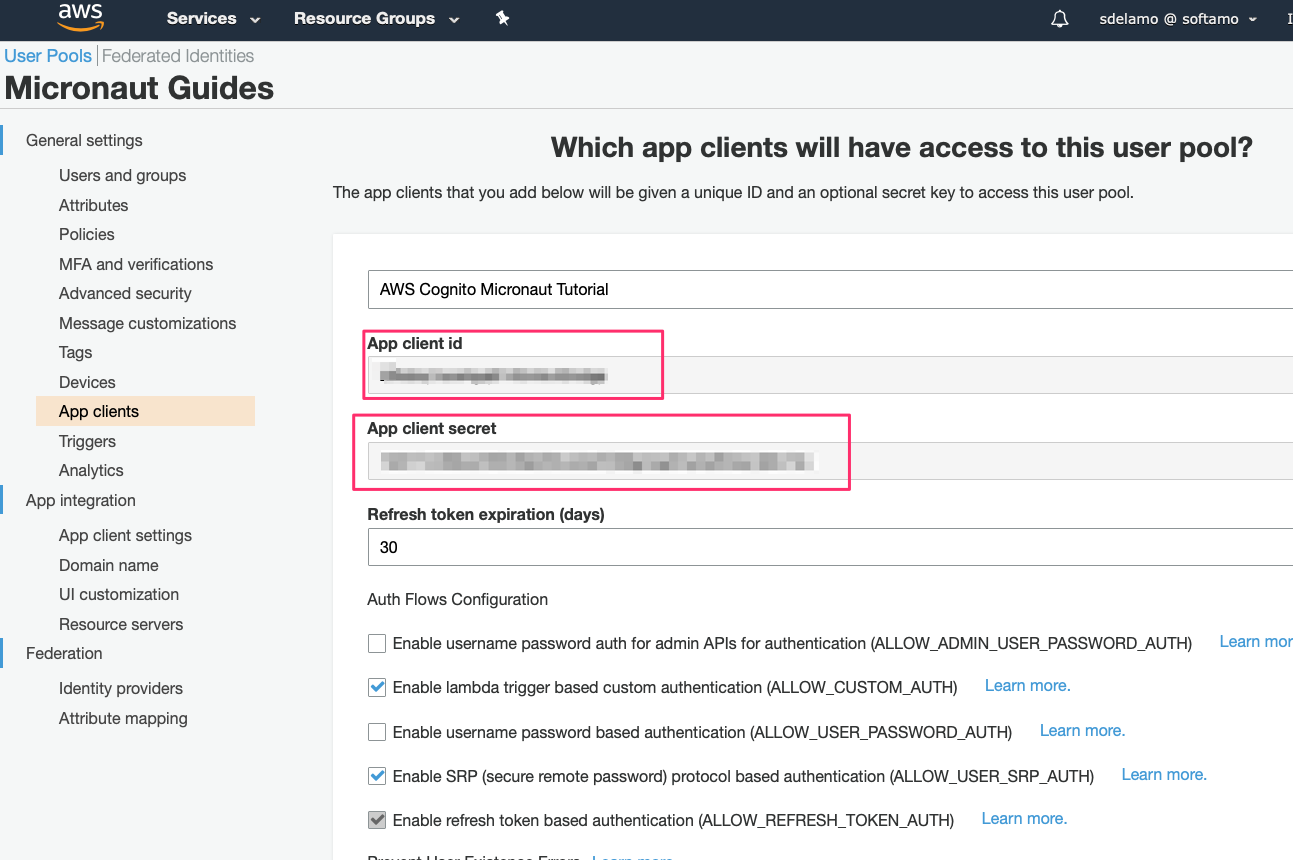
To use OAuth 2.0 integration, add the next dependency:
implementation("io.micronaut.security:micronaut-security-oauth2")
Add also JWT Micronaut’s JWT support dependencies:
implementation("io.micronaut.security:micronaut-security-jwt")
Add the following Oauth2 Configuration:
security:
authentication: idtoken (1)
oauth2:
clients:
cognito: (2)
client-id: '${OAUTH_CLIENT_ID:xxx}' (3)
client-secret: '${OAUTH_CLIENT_SECRET:yyy}' (4)
openid:
issuer: 'https://cognito-idp.${COGNITO_REGION:us-east-1}.amazonaws.com/${COGNITO_POOL_ID:12345}/' (5)
endpoints:
logout:
get-allowed: true (6)
1 | Set micronaut.security.authentication as idtoken. The idtoken provided by Cognito when the OAuth 2.0 Authorization code flow ends will be saved in a cookie. The id token is a signed JWT. For every request, Micronaut extracts the JWT from the Cookie and validates the JWT signature with the remote Json Web Key Set exposed by Cognito. JWKS is exposed by the jws-uri entry of Cognito .well-known/openid-configuration |
2 | The provider identifier should match the last part of the url you entered as a redirect url /oauth/callback/cognito |
3 | Client ID. See previous screenshot. |
4 | Client Secret. See previous screenshot. |
5 | issuer url. It allows micronaut to discover the configuration of the OpenID Connect server. Note: we will use the pool id and region mentioned previously. |
6 | Accept GET request to the /logout endpoint. |
The previous configuration uses several placeholders. You will need to setup OAUTH_CLIENT_ID
, OAUTH_CLIENT_SECRET
, COGNITO_REGION
and COGNITO_POOL_ID
environment variables.
export OAUTH_CLIENT_ID=XXXXXXXXXX export OAUTH_CLIENT_SECRET=YYYYYYYYYY export COGNITO_REGION=eu-west-1 export COGNITO_POOL_ID=eu-west-XXCCCAZZZ
Although undocumented in the Amazon Cognito User Pools Auth API Reference, Cognito provides an openid-configuration endpoint which is used by Micronaut to configure your app.
https://cognito-idp.{region}.amazonaws.com/{userPoolId}/.well-known/openid-configuration
We want to use an Authorization Code grant type flow which it is described in the following diagram:
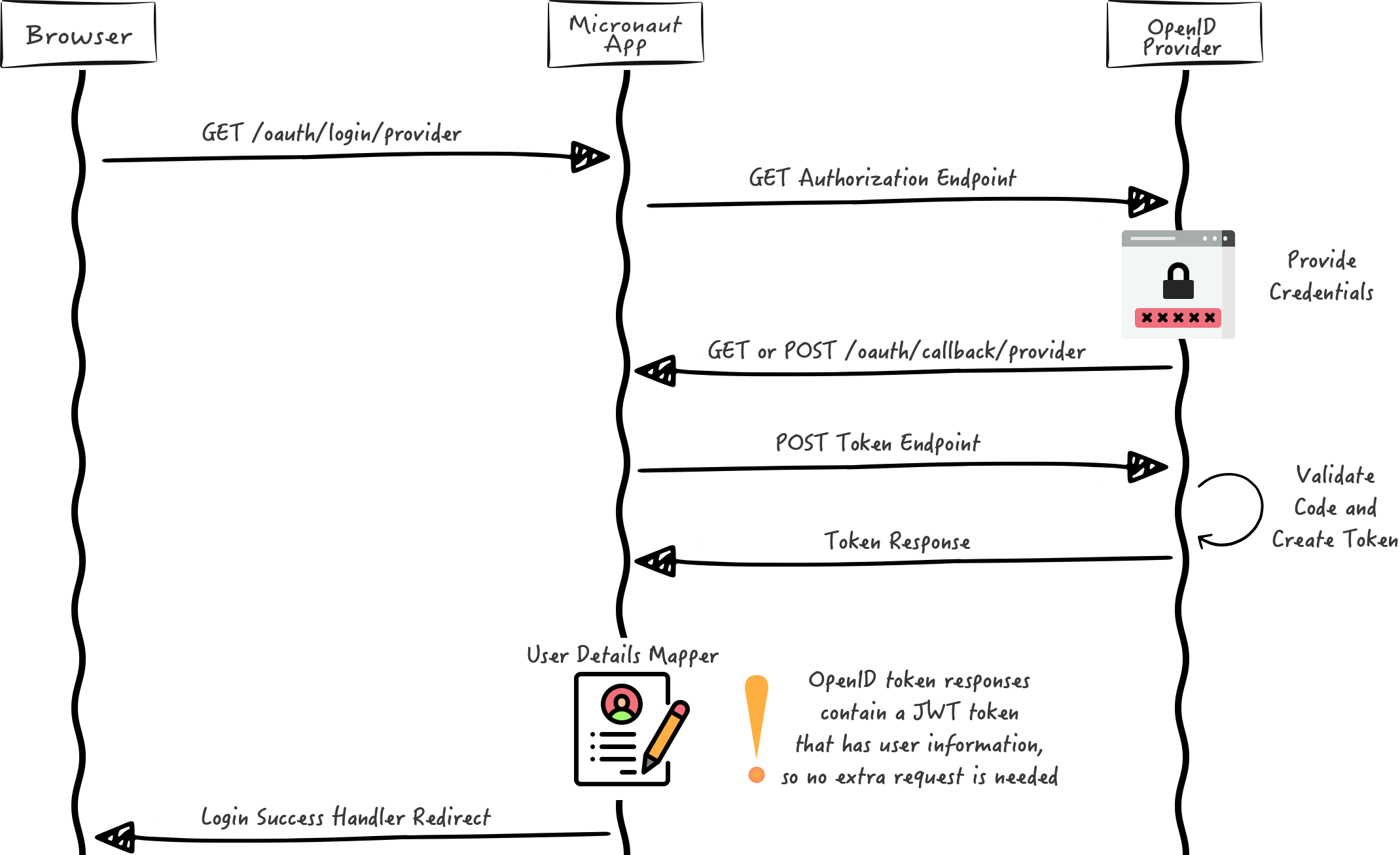
4.3. Home
Create a controller to handle the requests to /
. You are going to display the email of the authenticated person if any. Annotate the controller endpoint with @View
since we are going to use a Thymeleaf template.
package example.micronaut;
import io.micronaut.http.annotation.Controller;
import io.micronaut.http.annotation.Get;
import io.micronaut.security.annotation.Secured;
import io.micronaut.security.rules.SecurityRule;
import io.micronaut.views.View;
import java.util.HashMap;
import java.util.Map;
@Controller (1)
public class HomeController {
@Secured(SecurityRule.IS_ANONYMOUS) (2)
@View("home") (3)
@Get (4)
public Map<String, Object> index() {
return new HashMap<>();
}
}
1 | The class is defined as a controller with the @Controller annotation mapped to the path / . |
2 | Annotate with io.micronaut.security.Secured to configure secured access. The SecurityRule.IS_ANONYMOUS expression will allow access without authentication. |
3 | Use View annotation to specify which template would you like to render the response against. |
4 | The @Get annotation is used to map the index method to GET / requests. |
Create a thymeleaf template:
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Home</title>
</head>
<body>
<h1>Micronaut - Cognito example</h1>
<h2 th:if="${security}">username: <span th:text="${security.attributes.get('email')}"></span></h2>
<h2 th:unless="${security}">username: Anonymous</h2>
<nav>
<ul>
<li th:unless="${security}"><a href="/oauth/login/cognito">Enter</a></li>
<li th:if="${security}"><a href="/oauth/logout">Logout</a></li>
</ul>
</nav>
</body>
</html>
Also, note that we return an empty model in the controller. However, we are accessing security
in the thymeleaf template.
-
The SecurityViewModelProcessor injects into the model a
security
map with the authenticated user. See User in a view documentation.
5. Running the Application
To run the application use the ./gradlew run
command which will start the application on port 8080.
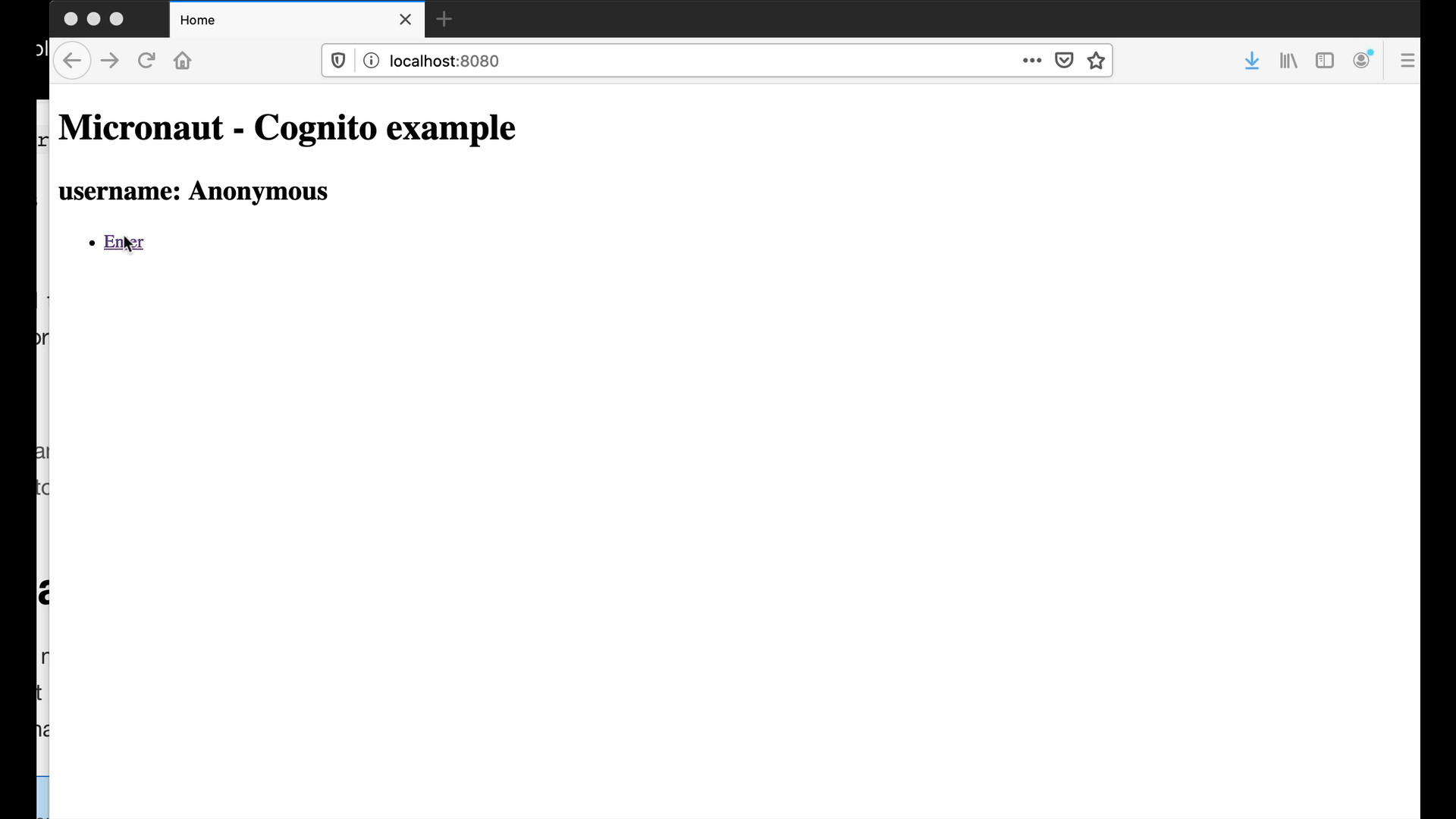
6. Generate a Micronaut app’s Native Image with GraalVM
We are going to use GraalVM, the polyglot embeddable virtual machine, to generate a Native image of our Micronaut application.
Native images compiled with GraalVM ahead-of-time improve the startup time and reduce the memory footprint of JVM-based applications.
Use of GraalVM’s native-image tool is only supported in Java or Kotlin projects. Groovy relies heavily on
reflection which is only partially supported by GraalVM.
|
6.1. Native Image generation
The easiest way to install GraalVM is to use SDKMan.io.
# For Java 8
$ sdk install java 21.1.0.r8-grl
# For Java 11
$ sdk install java 21.1.0.r11-grl
You need to install the native-image
component which is not installed by default.
$ gu install native-image
To generate a native image using Gradle run:
$ ./gradlew nativeImage
The native image will be created in build/native-image/application
and can be run with ./build/native-image/application
It is also possible to customize the name of the native image or pass additional parameters to GraalVM:
nativeImage {
args('--verbose')
imageName('mn-graalvm-application') (1)
}
1 | The native image name will now be mn-graalvm-application |
After you execute the native image, navigate to localhost:8080 and authenticate with Cognito.
7. Next steps
Read Micronaut OAuth 2.0 documentation to learn more.
8. Help with Micronaut
Object Computing, Inc. (OCI) sponsored the creation of this Guide. A variety of consulting and support services are available.