Eureka and Micronaut - Microservices service discovery
Use Netflix Eureka service discovery to expose your Micronaut apps.
Authors: Sergio del Amo
Micronaut Version: 2.5.0
1. Getting started
In this guide, we are going to create three microservices and register them with Netflix Eureka service discovery. You will discover how Micronaut eases Eureka integration.
2. What you will need
To complete this guide, you will need the following:
-
Some time on your hands
-
A decent text editor or IDE
-
JDK 1.8 or greater installed with
JAVA_HOME
configured appropriately
3. Solution
We recommend that you follow the instructions in the next sections and create the app step by step. However, you can go right to the completed example.
-
Download and unzip the source
4. Writing the App
Let’s describe the microservices:
-
bookcatalogue
- It returns a list of books. It uses a domain consisting of a book name and isbn. -
bookinventory
- It exposes an endpoint to check whether a book has sufficient stock to fulfil an order. It uses a domain consisting of a stock level and isbn. -
bookrecommendation
- It consumes previous services and exposes and endpoint which recommends book names which are in stock.
Initially we are going to hardcode the addresses where the different services are in the bookcatalogue
service.
As shown in the previous image, the bookcatalogue
hardcodes references to its collaborators.
In the second part of this tutorial we are going to use a discovery service.
The services register when they start up:
When a service wants to do a request to other service, it uses the discovery service to retrieve the address.
If you are using Java or Kotlin and IntelliJ IDEA, make sure you have enabled annotation processing.
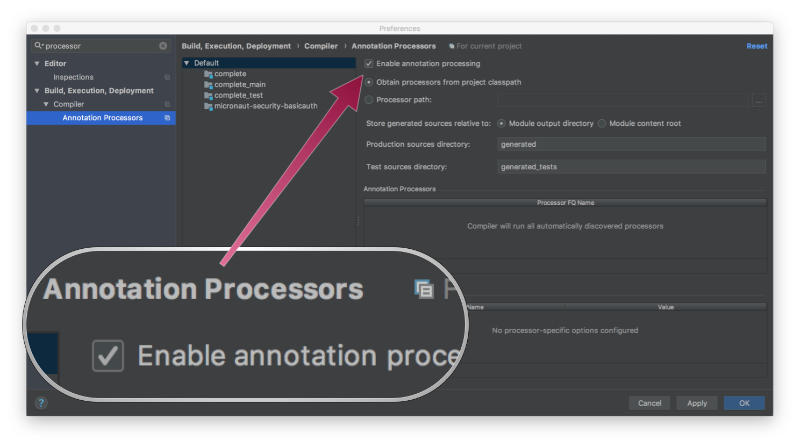
4.1. Catalogue Microservice
Create the bookcatalogue
microservice:
mn create-app example.micronaut.bookcatalogue --build=maven --lang=kotlin
The previous command creates a folder named bookcatalogue
and a Micronaut app inside it.
package example.micronaut
import io.micronaut.http.annotation.Controller
import io.micronaut.http.annotation.Get
@Controller("/books") (1)
class BooksController {
@Get (2)
internal fun index(): List<Book> {
return listOf(Book("1491950358", "Building Microservices"),
Book("1680502395", "Release It!"),
Book("0321601912", "Continuous Delivery:"))
}
}
1 | The class is defined as a controller with the @Controller annotation mapped to the path /books |
2 | The @Get annotation is used to map the index method to /books requests that use an HTTP GET. |
The previous controller responds a Flowable<Book>
. Create the Book
POJO:
package example.micronaut
data class Book(var isbn: String, val name: String)
Write a test:
package example.micronaut
import io.micronaut.core.type.Argument
import io.micronaut.http.HttpRequest
import io.micronaut.http.client.HttpClient
import io.micronaut.http.client.annotation.Client
import io.micronaut.test.extensions.junit5.annotation.MicronautTest
import org.junit.jupiter.api.Assertions
import org.junit.jupiter.api.Test
import javax.inject.Inject
@MicronautTest (1)
class BooksControllerTest {
@Inject
@field:Client("/")
lateinit var client: HttpClient (2)
@Test
fun testRetrieveBooks() {
val request: HttpRequest<*> = HttpRequest.GET<Any>("/books") (3)
val books: List<*> = client.toBlocking().retrieve(request, Argument.listOf(Book::class.java)) (4)
Assertions.assertEquals(3, books.size)
Assertions.assertTrue(books.contains(Book("1491950358", "Building Microservices")))
Assertions.assertTrue(books.contains(Book("1680502395", "Release It!")))
}
}
1 | Annotate the class with @MicronautTest to let Micronaut starts the embedded server and inject the beans. More info: https://micronaut-projects.github.io/micronaut-test/latest/guide/index.html. |
2 | Inject the HttpClient bean in the application context. |
3 | It is easy to create HTTP requests with a fluid API. |
4 | Parse easily JSON into Java objects. |
Edit application.yml
micronaut:
application:
name: bookcatalogue (1)
server:
port: 8081 (2)
1 | Configure the application name. The app name will be use by the discovery service. |
2 | Configure the app to listen at port 8081 |
Create a file named application-test.yml
which is used in the test environment:
micronaut:
server:
port: -1 (1)
1 | Start the micronaut microservice at a random port when running the tests. |
Run the unit test:
bookcatalogue $ ./mvnw test
4.2. Inventory Microservice
Create the bookinventory
microservice:
mn create-app example.micronaut.bookinventory --build=maven --lang=kotlin
The previous command creates a folder named bookinventory
and a Micronaut app inside it.
Create a Controller:
package example.micronaut
import io.micronaut.http.MediaType
import io.micronaut.http.annotation.Controller
import io.micronaut.http.annotation.Get
import io.micronaut.http.annotation.Produces
import java.util.Optional
import javax.validation.constraints.NotBlank
@Controller("/books") (1)
open class BooksController {
@Produces(MediaType.TEXT_PLAIN) (2)
@Get("/stock/{isbn}") (3)
open fun stock(@NotBlank isbn: String): Boolean? {
return bookInventoryByIsbn(isbn).map { (_, stock) -> stock > 0 }.orElse(null)
}
private fun bookInventoryByIsbn(isbn: String): Optional<BookInventory> {
if (isbn == "1491950358") {
return Optional.of(BookInventory(isbn, 4))
} else if (isbn == "1680502395") {
return Optional.of(BookInventory(isbn, 0))
}
return Optional.empty()
}
}
1 | The class is defined as a controller with the @Controller annotation mapped to the path /books |
2 | By default a Micronaut’s response uses application/json as Content-Type . We are returning a String not a JSON object. Because of that, we set it to text/plain . |
3 | The @Get annotation is used to map the index method to /books/stock/{isbn} requests that use an HTTP GET. |
The previous controller uses a POJO:
package example.micronaut
data class BookInventory(var isbn: String, val stock: Int)
Write a test:
package example.micronaut
import io.micronaut.http.HttpRequest
import io.micronaut.http.HttpStatus
import io.micronaut.http.client.RxHttpClient
import io.micronaut.http.client.annotation.Client
import io.micronaut.http.client.exceptions.HttpClientResponseException
import io.micronaut.test.extensions.junit5.annotation.MicronautTest
import org.junit.jupiter.api.Assertions
import org.junit.jupiter.api.Test
import javax.inject.Inject
@MicronautTest
class BooksControllerTest {
@Inject
@field:Client("/")
lateinit var rxHttpClient: RxHttpClient
@Test
fun testBooksController() {
val rsp = rxHttpClient.toBlocking().exchange(HttpRequest.GET<Any>("/books/stock/1491950358"), Boolean::class.java)
Assertions.assertEquals(rsp.status(), HttpStatus.OK)
Assertions.assertTrue(rsp.body()!!)
}
@Test
fun testBooksControllerWithNonExistingIsbn() {
val thrown = Assertions.assertThrows(HttpClientResponseException::class.java) { rxHttpClient.toBlocking().exchange(HttpRequest.GET<Any>("/books/stock/XXXXX"), Boolean::class.java) }
Assertions.assertEquals(
HttpStatus.NOT_FOUND,
thrown.response.status
)
}
}
Edit application.yml
micronaut:
application:
name: bookinventory (1)
server:
port: 8082 (2)
1 | Configure the application name. The app name will be used later in the tutorial. |
2 | Configure the app to listen at port 8082 |
Create a file named application-test.yml
which is used in the test environment:
micronaut:
server:
port: -1 (1)
1 | Start the micronaut microservice at a random port when running the tests. |
Run the unit test:
bookinventory $ ./mvnw test
4.3. Recommendation Microservice
Create the bookrecommendation
microservice:
mn create-app example.micronaut.bookrecommendation --build=maven --lang=kotlin
The previous command creates a folder named bookrecommendation
and a Micronaut app inside it.
Create an interface to map operations with bookcatalogue
, and a Micronaut Declarative HTTP Client to consume it.
package example.micronaut
import io.reactivex.Flowable
interface BookCatalogueOperations {
fun findAll(): Flowable<Book>
}
package example.micronaut
import io.micronaut.http.annotation.Get
import io.micronaut.http.client.annotation.Client
import io.micronaut.retry.annotation.Recoverable
import io.reactivex.Flowable
@Client("http://localhost:8081") (1)
@Recoverable(api = BookCatalogueOperations::class)
interface BookCatalogueClient : BookCatalogueOperations {
@Get("/books")
override fun findAll(): Flowable<Book>
}
1 | Use @Client to use declarative HTTP Clients |
The client returns a POJO. Create it in the bookrecommendation
:
package example.micronaut
data class Book(var isbn: String, val name: String)
Create an interface to map operations with bookinventory
, and a Micronaut Declarative HTTP Client to consume it.
package example.micronaut
import io.reactivex.Maybe
import javax.validation.constraints.NotBlank
interface BookInventoryOperations {
fun stock(@NotBlank isbn: String): Maybe<Boolean>
}
package example.micronaut
import io.micronaut.http.annotation.Get
import io.micronaut.http.client.annotation.Client
import io.micronaut.retry.annotation.Recoverable
import io.reactivex.Flowable
@Client("http://localhost:8081") (1)
@Recoverable(api = BookCatalogueOperations::class)
interface BookCatalogueClient : BookCatalogueOperations {
@Get("/books")
override fun findAll(): Flowable<Book>
}
1 | Use @Client to use declarative HTTP Clients |
Create a Controller which injects both clients.
package example.micronaut
import io.micronaut.http.annotation.Controller
import io.micronaut.http.annotation.Get
import io.reactivex.Flowable
@Controller("/books") (1)
class BookController(private val bookCatalogueOperations: BookCatalogueOperations,
private val bookInventoryOperations: BookInventoryOperations) { (2)
@Get (3)
fun index(): Flowable<BookRecommendation> {
return bookCatalogueOperations.findAll()
.flatMapMaybe { b ->
bookInventoryOperations.stock(b.isbn)
.filter { hasStock -> hasStock }
.map { _ -> b }
}.map { (_, name) -> BookRecommendation(name) }
}
}
1 | The class is defined as a controller with the @Controller annotation mapped to the path /books |
2 | Constructor injection |
3 | The @Get annotation is used to map the index method to /books requests that use an HTTP GET. |
The previous controller returns a Flowable<BookRecommendation>
. Create the BookRecommendation
POJO:
package example.micronaut
data class BookRecommendation(val name: String)
BookCatalogueClient
and BookInventoryClient
will fail to consume the bookcatalogue
and bookinventory
during the tests phase.
Using the @Fallback annotation you can declare a fallback implementation of a client that will be picked up and used once all possible retries have been exhausted
Create @Fallback
alternatives in the test
classpath.
package example.micronaut
import io.micronaut.context.annotation.Requires
import io.micronaut.context.env.Environment
import io.micronaut.retry.annotation.Fallback
import io.reactivex.Maybe
import javax.inject.Singleton
import javax.validation.constraints.NotBlank
@Requires(env = arrayOf(Environment.TEST)) (1)
@Fallback
@Singleton
open class BookInventoryClientStub : BookInventoryOperations {
override fun stock(@NotBlank isbn: String): Maybe<Boolean> {
if (isbn == "1491950358") {
return Maybe.just(java.lang.Boolean.TRUE) (2)
} else if (isbn == "1680502395") {
return Maybe.just(java.lang.Boolean.FALSE) (3)
}
return Maybe.empty() (4)
}
}
1 | Make this fallback class to be effective only when the micronaut environment TEST is active |
2 | Here we arbitrarily decided that if everything else fails, that book’s stock would be true |
3 | Similarly, we decided that other book’s stock method would be false |
4 | Finally, any other book will have their stock method return an empty value |
package example.micronaut
import io.micronaut.context.annotation.Requires
import io.micronaut.context.env.Environment
import io.micronaut.retry.annotation.Fallback
import io.reactivex.Flowable
import javax.inject.Singleton
@Requires(env = arrayOf(Environment.TEST))
@Fallback
@Singleton
class BookCatalogueClientStub : BookCatalogueOperations {
override fun findAll(): Flowable<Book> {
val buildingMicroservices = Book("1491950358", "Building Microservices")
val releaseIt = Book("1680502395", "Release It!")
return Flowable.just(buildingMicroservices, releaseIt)
}
}
Write a test:
package example.micronaut
import io.micronaut.http.HttpRequest
import io.micronaut.http.client.RxStreamingHttpClient
import io.micronaut.http.client.annotation.Client
import io.micronaut.test.extensions.junit5.annotation.MicronautTest
import org.junit.jupiter.api.Assertions
import org.junit.jupiter.api.Test
import javax.inject.Inject
@MicronautTest
class BookControllerTest {
@Inject
@field:Client("/")
lateinit var client: RxStreamingHttpClient
@Test
fun testRetrieveBooks() {
val books = client.jsonStream(HttpRequest.GET<Any>("/books"), BookRecommendation::class.java)
Assertions.assertEquals(books.toList().blockingGet().size, 1)
Assertions.assertEquals(books.toList().blockingGet()[0].name, "Building Microservices")
}
}
Edit application.yml
micronaut:
application:
name: bookrecommendation (1)
server:
port: 8080 (2)
1 | Configure the application name. The app name will be used later in the tutorial. |
2 | Configure the app to listen at port 8080 |
Create a file named application-test.yml
which is used in the test environment:
micronaut:
server:
port: -1 (1)
1 | Start the micronaut microservice at a random port when running the tests. |
Run the unit test:
bookinventory $ ./mvnw test
4.4. Running the app
Run bookcatalogue
microservice:
To run the application execute ./mvnw mn:run
.
...
14:28:34.034 [main] INFO io.micronaut.runtime.Micronaut - Startup completed in 499ms. Server Running: http://localhost:8081
Run bookinventory
microservice:
To run the application execute ./mvnw mn:run
.
...
14:31:13.104 [main] INFO io.micronaut.runtime.Micronaut - Startup completed in 506ms. Server Running: http://localhost:8082
Run bookrecommendation
microservice:
To run the application execute ./mvnw mn:run
.
...
14:31:57.389 [main] INFO io.micronaut.runtime.Micronaut - Startup completed in 523ms. Server Running: http://localhost:8080
You can run a cURL command to test the whole application:
$ curl http://localhost:8080/books
[{"name":"Building Microservices"}]
5. Eureka and Micronaut
Eureka is a REST (Representational State Transfer) based service that is primarily used in the AWS cloud for locating services for the purpose of load balancing and failover of middle-tier servers.
5.1. Eureka Server
Spring-Cloud-Netflix provides a very neat way to bootstrap Eureka. To bring up Eureka server using Spring-Cloud-Netflix:
-
Clone the sample Eureka server application.
-
Run this project as a Spring Boot app (e.g. import into IDE and run main method, or use
mvn spring-boot:run
or./gradlew bootRun
). It will start up on port 8761 and serve the Eureka API from/eureka
.
5.2. Book Catalogue
Add discovery-client
dependency.
<dependency>
<groupId>io.micronaut.discovery</groupId>
<artifactId>micronaut-discovery-client</artifactId>
<scope>compile</scope>
</dependency>
Append to bookcatalogue
service application.yml
the following snippet:
eureka:
client:
registration:
enabled: true
defaultZone: "${EUREKA_HOST:localhost}:${EUREKA_PORT:8761}"
Previous configuration registers a Micronaut app with Eureka with minimal configuration. Discover a more complete list of Configuration options at EurekaConfiguration.
Disable Eureka registration in tests:
eureka:
client:
registration:
enabled: false
5.3. Book Inventory
Add discovery-client
dependency.
<dependency>
<groupId>io.micronaut.discovery</groupId>
<artifactId>micronaut-discovery-client</artifactId>
<scope>compile</scope>
</dependency>
Also, append to bookinventory
.application.yml
the following snippet:
eureka:
client:
registration:
enabled: true
defaultZone: "${EUREKA_HOST:localhost}:${EUREKA_PORT:8761}"
Disable Eureka registration in tests:
eureka:
client:
registration:
enabled: false
5.4. Book Recommendation
Add discovery-client
dependency.
<dependency>
<groupId>io.micronaut.discovery</groupId>
<artifactId>micronaut-discovery-client</artifactId>
<scope>compile</scope>
</dependency>
Also, append to bookrecommendation
.application.yml
the following snippet:
eureka:
client:
registration:
enabled: true
defaultZone: "${EUREKA_HOST:localhost}:${EUREKA_PORT:8761}"
Modify BookInventoryClient
and BookCatalogueClient
to use the service id instead of a harcoded ip.
package example.micronaut
import io.micronaut.http.annotation.Get
import io.micronaut.http.client.annotation.Client
import io.micronaut.retry.annotation.Recoverable
import io.reactivex.Flowable
@Client(id = "bookcatalogue") (1)
@Recoverable(api = BookCatalogueOperations::class)
interface BookCatalogueClient : BookCatalogueOperations {
@Get("/books")
override fun findAll(): Flowable<Book>
}
1 | Use the configuration value micronaut.application.name used in bookcatalogue as service id . |
package example.micronaut
import io.micronaut.http.MediaType
import io.micronaut.http.annotation.Consumes
import io.micronaut.http.annotation.Get
import io.micronaut.http.client.annotation.Client
import io.micronaut.retry.annotation.Recoverable
import io.reactivex.Maybe
import javax.validation.constraints.NotBlank
@Client(id = "bookinventory") (1)
@Recoverable(api = BookInventoryOperations::class)
interface BookInventoryClient : BookInventoryOperations {
@Consumes(MediaType.TEXT_PLAIN)
@Get("/books/stock/{isbn}")
override fun stock(@NotBlank isbn: String): Maybe<Boolean>
}
1 | Use the configuration value micronaut.application.name used in bookinventory as service id . |
Disable Eureka registration in tests:
eureka:
client:
registration:
enabled: false
5.5. Running the App
Run bookcatalogue
microservice:
To run the application execute ./mvnw mn:run
.
...
14:28:34.034 [main] INFO io.micronaut.runtime.Micronaut - Startup completed in 499ms. Server Running: http://localhost:8081
Run bookinventory
microservice:
To run the application execute ./mvnw mn:run
.
...
14:31:13.104 [main] INFO io.micronaut.runtime.Micronaut - Startup completed in 506ms. Server Running: http://localhost:8082
Run bookrecommendation
microservice:
To run the application execute ./mvnw mn:run
.
...
14:31:57.389 [main] INFO io.micronaut.runtime.Micronaut - Startup completed in 523ms. Server Running: http://localhost:8080
You can run a cURL command to test the whole application:
$ curl http://localhost:8080/books
[{"name":"Building Microservices"}]
Open http://localhost:8761 in your browser.
You will see the services registered in Eureka:
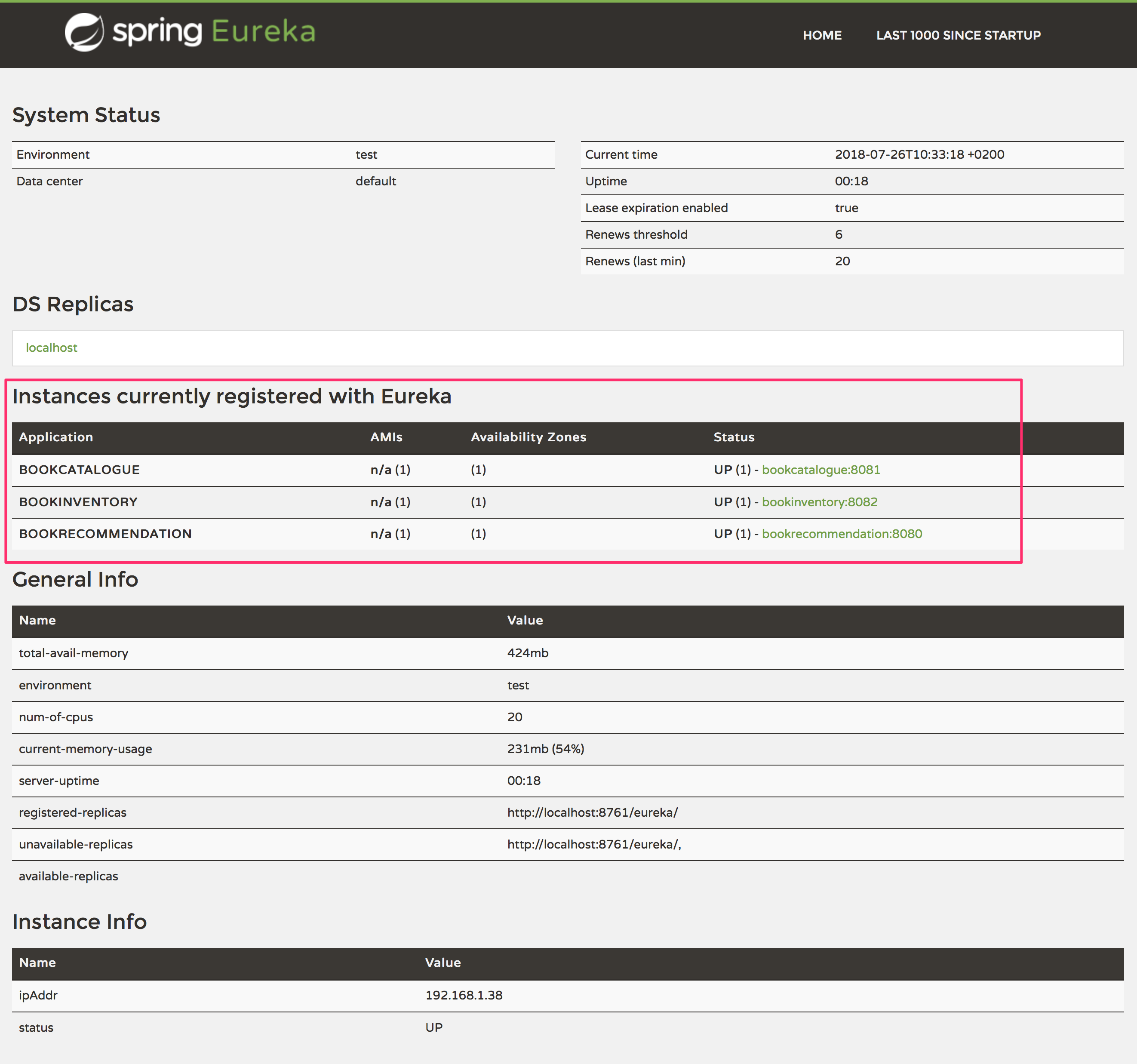
You can run a cURL command to test the whole application:
$ curl http://localhost:8080/books
[{"name":"Building Microservices"}]
6. Generate a Micronaut app’s Native Image with GraalVM
We are going to use GraalVM, the polyglot embeddable virtual machine, to generate a Native image of our Micronaut application.
Native images compiled with GraalVM ahead-of-time improve the startup time and reduce the memory footprint of JVM-based applications.
Use of GraalVM’s native-image tool is only supported in Java or Kotlin projects. Groovy relies heavily on
reflection which is only partially supported by GraalVM.
|
6.1. Native Image generation
The easiest way to install GraalVM is to use SDKMan.io.
# For Java 8
$ sdk install java 21.1.0.r8-grl
# For Java 11
$ sdk install java 21.1.0.r11-grl
You need to install the native-image
component which is not installed by default.
$ gu install native-image
To generate a native image using Maven run:
$ ./mvnw package -Dpackaging=native-image
The native image will be created in target/application
and can be run with ./target/application
.
Start the native images for the three microservices and run the same curl
request as before to check that everything works with GraalVM.
7. Next steps
Read more about Eureka Support inside Micronaut.
8. Help with Micronaut
Object Computing, Inc. (OCI) sponsored the creation of this Guide. A variety of consulting and support services are available.