mn create-app example.micronaut.micronautguide --build=maven --lang=java
Access a database with JPA and Hibernate
Learn how to access a database with JPA and Hibernate using Micronaut.
Authors: Iván López, Sergio del Amo
Micronaut Version: 2.5.0
1. Getting Started
In this guide we are going to create a Micronaut app written in Java.
In this guide, we are going to write a Micronaut application that exposes some REST endpoints and store data in a database using JPA and Hibernate.
2. What you will need
To complete this guide, you will need the following:
-
Some time on your hands
-
A decent text editor or IDE
-
JDK 1.8 or greater installed with
JAVA_HOME
configured appropriately
3. Solution
We recommend that you follow the instructions in the next sections and create the app step by step. However, you can go right to the completed example.
-
Download and unzip the source
4. Writing the App
Create an app using the Micronaut Command Line Interface or with Micronaut Launch.
If you don’t specify the --build argument, Gradle is used as a build tool. If you don’t specify the --lang argument, Java is used as a language.
|
The previous command creates a Micronaut app with the default package example.micronaut
in a folder named micronautguide
.
If you are using Java or Kotlin and IntelliJ IDEA, make sure you have enabled annotation processing.
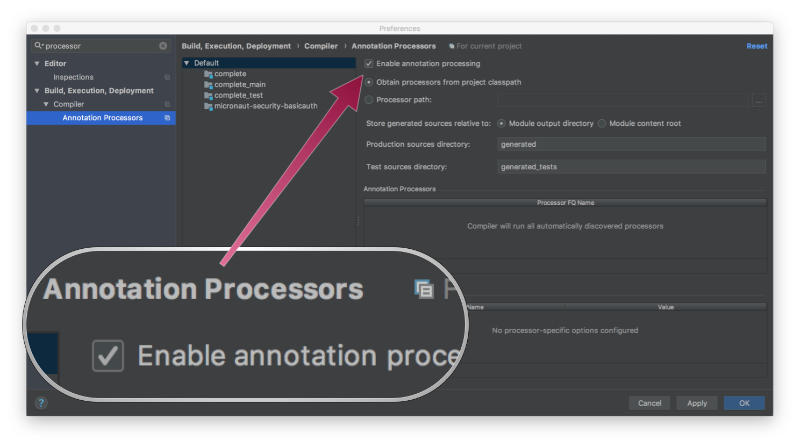
4.1. Data Source configuration
Add the following dependencies:
<dependency> (1)
<groupId>io.micronaut.sql</groupId>
<artifactId>micronaut-hibernate-jpa</artifactId>
<scope>compile</scope>
</dependency>
<dependency> (2)
<groupId>io.micronaut.sql</groupId>
<artifactId>micronaut-jdbc-hikari</artifactId>
<scope>compile</scope>
</dependency>
<dependency> (3)
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<scope>runtime</scope>
</dependency>
1 | Configures Hibernate/JPA EntityManagerFactory beans. |
2 | Configures SQL DataSource instances using Hikari Connection Pool. |
3 | Add dependency to in-memory H2 Database. |
Define the data source in src/main/resources/application.yml
.
datasources:
default:
url: ${JDBC_URL:`jdbc:h2:mem:default;DB_CLOSE_DELAY=-1;DB_CLOSE_ON_EXIT=FALSE`}
username: ${JDBC_USER:sa}
password: ${JDBC_PASSWORD:""}
driverClassName: ${JDBC_DRIVER:org.h2.Driver}
This way of defining the datasource properties means that we can externalize the configuration, for example for
production environment, and also provide a default value for development. If the environment variables are not defined
Micronaut will use the default values. Also keep in mind that it is necessary to escape the : in the connection url using back ticks ` .
|
4.2. JPA configuration
Add the next snippet to src/main/resources/application.yml
to configure JPA:
jpa:
default:
properties:
hibernate:
hbm2ddl:
auto: update
show_sql: true
4.3. Domain
Create the domain entities:
package example.micronaut.domain;
import com.fasterxml.jackson.annotation.JsonIgnore;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
import javax.persistence.OneToMany;
import javax.persistence.Table;
import javax.validation.constraints.NotNull;
import java.util.HashSet;
import java.util.Set;
@Entity
@Table(name = "genre")
public class Genre {
public Genre() {}
public Genre(@NotNull String name) {
this.name = name;
}
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
@NotNull
@Column(name = "name", nullable = false, unique = true)
private String name;
@JsonIgnore
@OneToMany(mappedBy = "genre")
private Set<Book> books = new HashSet<>();
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Set<Book> getBooks() {
return books;
}
public void setBooks(Set<Book> books) {
this.books = books;
}
@Override
public String toString() {
return "Genre{" +
"id=" + id +
", name='" + name + '\'' +
'}';
}
}
The previous domain has a OneToMany
relationship with the domain Book
.
package example.micronaut.domain;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
import javax.persistence.ManyToOne;
import javax.persistence.Table;
import javax.validation.constraints.NotNull;
@Entity
@Table(name = "book")
public class Book {
public Book() {}
public Book(@NotNull String isbn, @NotNull String name, Genre genre) {
this.isbn = isbn;
this.name = name;
this.genre = genre;
}
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
@NotNull
@Column(name = "name", nullable = false)
private String name;
@NotNull
@Column(name = "isbn", nullable = false)
private String isbn;
@ManyToOne
private Genre genre;
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getIsbn() {
return isbn;
}
public void setIsbn(String isbn) {
this.isbn = isbn;
}
public Genre getGenre() {
return genre;
}
public void setGenre(Genre genre) {
this.genre = genre;
}
@Override
public String toString() {
return "Book{" +
"id=" + id +
", name='" + name + '\'' +
", isbn='" + isbn + '\'' +
", genre=" + genre +
'}';
}
}
4.4. Application Configuration
Create an interface to encapsulate the application configuration settings:
package example.micronaut;
import javax.validation.constraints.NotNull;
public interface ApplicationConfiguration {
@NotNull Integer getMax();
}
In Micronaut, like Spring Boot and Grails, you can create type safe configuration by creating classes that are annotated with @ConfigurationProperties.
Create a ApplicationConfigurationProperties
class:
package example.micronaut;
import io.micronaut.context.annotation.ConfigurationProperties;
import io.micronaut.core.annotation.NonNull;
@ConfigurationProperties("application") (1)
public class ApplicationConfigurationProperties implements ApplicationConfiguration {
protected final Integer DEFAULT_MAX = 10;
@NonNull
private Integer max = DEFAULT_MAX;
@Override
@NonNull
public Integer getMax() {
return max;
}
public void setMax(@NonNull Integer max) {
if(max != null) {
this.max = max;
}
}
}
1 | @ConfigurationProperties` annotation takes a configuration prefix. |
You can override max
if you add to your src/main/resources/application.yml
:
application:
max: 50
4.5. Repository Access
To mark the transaction demarcations, we use the Java EE 7 javax.transaction.Transactional annotation.
To use it, you have to include the micronaut-data-processor
dependency in your annotation processor configuration:
<!-- Add the following to your annotationProcessorPaths element -->
<path>
<groupId>io.micronaut.data</groupId>
<artifactId>micronaut-data-hibernate-jpa</artifactId>
</path>
Next, create an interface to define the operations to access the database:
package example.micronaut;
import example.micronaut.domain.Genre;
import javax.validation.constraints.NotBlank;
import javax.validation.constraints.NotNull;
import java.util.List;
import java.util.Optional;
public interface GenreRepository {
Optional<Genre> findById(@NotNull Long id);
Genre save(@NotBlank String name);
Genre saveWithException(@NotBlank String name);
void deleteById(@NotNull Long id);
List<Genre> findAll(@NotNull SortingAndOrderArguments args);
int update(@NotNull Long id, @NotBlank String name);
}
The implementation:
package example.micronaut;
import example.micronaut.domain.Genre;
import javax.inject.Singleton;
import javax.persistence.EntityManager;
import javax.persistence.PersistenceException;
import javax.persistence.TypedQuery;
import javax.transaction.Transactional;
import io.micronaut.transaction.annotation.ReadOnly;
import javax.validation.constraints.NotBlank;
import javax.validation.constraints.NotNull;
import java.util.Arrays;
import java.util.List;
import java.util.Optional;
@Singleton (1)
public class GenreRepositoryImpl implements GenreRepository {
private final EntityManager entityManager; (2)
private final ApplicationConfiguration applicationConfiguration;
private final static List<String> VALID_PROPERTY_NAMES = Arrays.asList("id", "name");
public GenreRepositoryImpl(EntityManager entityManager,
ApplicationConfiguration applicationConfiguration) { (2)
this.entityManager = entityManager;
this.applicationConfiguration = applicationConfiguration;
}
@Override
@ReadOnly (3)
public Optional<Genre> findById(@NotNull Long id) {
return Optional.ofNullable(entityManager.find(Genre.class, id));
}
@Override
@Transactional (4)
public Genre save(@NotBlank String name) {
Genre genre = new Genre(name);
entityManager.persist(genre);
return genre;
}
@Override
@Transactional (4)
public void deleteById(@NotNull Long id) {
findById(id).ifPresent(entityManager::remove);
}
@ReadOnly (3)
public List<Genre> findAll(@NotNull SortingAndOrderArguments args) {
String qlString = "SELECT g FROM Genre as g";
if (args.getOrder().isPresent() && args.getSort().isPresent() && VALID_PROPERTY_NAMES.contains(args.getSort().get())) {
qlString += " ORDER BY g." + args.getSort().get() + " " + args.getOrder().get().toLowerCase();
}
TypedQuery<Genre> query = entityManager.createQuery(qlString, Genre.class);
query.setMaxResults(args.getMax().orElseGet(applicationConfiguration::getMax));
args.getOffset().ifPresent(query::setFirstResult);
return query.getResultList();
}
@Override
@Transactional (4)
public int update(@NotNull Long id, @NotBlank String name) {
return entityManager.createQuery("UPDATE Genre g SET name = :name where id = :id")
.setParameter("name", name)
.setParameter("id", id)
.executeUpdate();
}
@Override (4)
@Transactional (4)
public Genre saveWithException(@NotBlank String name) {
save(name);
throw new PersistenceException();
}
}
1 | Use javax.inject.Singleton to designate a class as a singleton. |
2 | Inject easily an EntityManager . |
3 | All database access needs to be wrapped inside a transaction. As the method
only reads data from the database, annotate it with @ReadOnly . |
4 | This method modifies the database, thus it is annoated with @Transactional . |
4.6. Controller
Micronaut’s validation is built on the standard framework – JSR 380, also known as Bean Validation 2.0.
Hibernate Validator is a reference implementation of the validation API. Starting with Micronaut 1.2, Micronaut
has built-in support for validation of beans that
are annotated with javax.validation
annotations.
The necessary dependencies are included by default when creating a new application, so you don’t need to add anything else.
Create two classes to encapsulate Save and Update operations:
package example.micronaut;
import io.micronaut.core.annotation.Introspected;
import javax.validation.constraints.NotBlank;
@Introspected (1)
public class GenreSaveCommand {
@NotBlank
private String name;
public GenreSaveCommand() {
}
public GenreSaveCommand(String name) {
this.name = name;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
1 | Annotate the class with @Introspected to generate the Bean Metainformation at compile time. |
package example.micronaut;
import io.micronaut.core.annotation.Introspected;
import javax.validation.constraints.NotBlank;
import javax.validation.constraints.NotNull;
@Introspected
public class GenreUpdateCommand {
@NotNull
private Long id;
@NotBlank
private String name;
public GenreUpdateCommand() {
}
public GenreUpdateCommand(Long id, String name) {
this.id = id;
this.name = name;
}
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
Create a POJO to encapsulate Sorting and Pagination:
package example.micronaut;
import io.micronaut.core.annotation.Introspected;
import io.micronaut.core.annotation.Nullable;
import javax.validation.constraints.Pattern;
import javax.validation.constraints.Positive;
import javax.validation.constraints.PositiveOrZero;
import java.util.Optional;
@Introspected
public class SortingAndOrderArguments {
@Nullable
@PositiveOrZero (1)
private Integer offset;
@Nullable
@Positive (1)
private Integer max;
@Nullable
@Pattern(regexp = "id|name") (1)
private String sort;
@Pattern(regexp = "asc|ASC|desc|DESC") (1)
@Nullable
private String order;
public SortingAndOrderArguments() {
}
public Optional<Integer> getOffset() {
return Optional.ofNullable(offset);
}
public void setOffset(@Nullable Integer offset) {
this.offset = offset;
}
public Optional<Integer> getMax() {
return Optional.ofNullable(max);
}
public void setMax(@Nullable Integer max) {
this.max = max;
}
public Optional<String> getSort() {
return Optional.ofNullable(sort);
}
public void setSort(@Nullable String sort) {
this.sort = sort;
}
public Optional<String> getOrder() {
return Optional.ofNullable(order);
}
public void setOrder(@Nullable String order) {
this.order = order;
}
}
1 | Use javax.validation.constraints Constraints to ensure the incoming data matches your expectations. |
Create GenreController
, a controller which exposes a resource with the common CRUD operations:
package example.micronaut;
import example.micronaut.domain.Genre;
import io.micronaut.http.HttpHeaders;
import io.micronaut.http.HttpResponse;
import io.micronaut.http.annotation.Body;
import io.micronaut.http.annotation.Controller;
import io.micronaut.http.annotation.Delete;
import io.micronaut.http.annotation.Get;
import io.micronaut.http.annotation.Post;
import io.micronaut.http.annotation.Put;
import io.micronaut.scheduling.TaskExecutors;
import io.micronaut.scheduling.annotation.ExecuteOn;
import javax.persistence.PersistenceException;
import javax.validation.Valid;
import java.net.URI;
import java.util.List;
@ExecuteOn(TaskExecutors.IO) (1)
@Controller("/genres") (2)
public class GenreController {
protected final GenreRepository genreRepository;
public GenreController(GenreRepository genreRepository) { (3)
this.genreRepository = genreRepository;
}
@Get("/{id}") (4)
public Genre show(Long id) {
return genreRepository
.findById(id)
.orElse(null); (5)
}
@Put (6)
public HttpResponse update(@Body @Valid GenreUpdateCommand command) { (7)
int numberOfEntitiesUpdated = genreRepository.update(command.getId(), command.getName());
return HttpResponse
.noContent()
.header(HttpHeaders.LOCATION, location(command.getId()).getPath()); (8)
}
@Get(value = "/list{?args*}") (9)
public List<Genre> list(@Valid SortingAndOrderArguments args) {
return genreRepository.findAll(args);
}
@Post (10)
public HttpResponse<Genre> save(@Body @Valid GenreSaveCommand cmd) {
Genre genre = genreRepository.save(cmd.getName());
return HttpResponse
.created(genre)
.headers(headers -> headers.location(location(genre.getId())));
}
@Post("/ex") (11)
public HttpResponse<Genre> saveExceptions(@Body @Valid GenreSaveCommand cmd) {
try {
Genre genre = genreRepository.saveWithException(cmd.getName());
return HttpResponse
.created(genre)
.headers(headers -> headers.location(location(genre.getId())));
} catch(PersistenceException e) {
return HttpResponse.noContent();
}
}
@Delete("/{id}") (12)
public HttpResponse delete(Long id) {
genreRepository.deleteById(id);
return HttpResponse.noContent();
}
protected URI location(Long id) {
return URI.create("/genres/" + id);
}
protected URI location(Genre genre) {
return location(genre.getId());
}
}
1 | It is critical that any blocking I/O operations (such as fetching the data from the database) are offloaded to a separate thread pool that does not block the Event loop. |
2 | The class is defined as a controller with the @Controller annotation mapped to the path /genres . |
3 | Constructor injection. |
4 | Maps a GET request to /genres/{id} which attempts to show a genre. This illustrates the use of a URL path variable. |
5 | Returning null when the genre doesn’t exist makes Micronaut to response with 404 (not found). |
6 | Maps a PUT request to /genres which attempts to update a genre. |
7 | Add @Valid to any method parameter which requires validation. Use a POJO supplied as a JSON payload in the request to populate command. |
8 | It is easy to add custom headers to the response. |
9 | Maps a GET request to /genres which returns a list of genres. This mapping illustrates URL parameters being mapped to a single POJO. |
10 | Maps a POST request to /genres which attempts to save a genre. |
11 | Maps a POST request to /ex which generates an exception. |
12 | Maps a DELETE request to /genres/{id} which attempts to remove a genre. This illustrates the use of a URL path variable. |
4.7. Writing Tests
Create a test which verifies the CRUD operations:
package example.micronaut;
import example.micronaut.domain.Genre;
import io.micronaut.core.type.Argument;
import io.micronaut.http.HttpHeaders;
import io.micronaut.http.HttpRequest;
import io.micronaut.http.HttpResponse;
import io.micronaut.http.HttpStatus;
import io.micronaut.http.client.HttpClient;
import io.micronaut.http.client.annotation.Client;
import io.micronaut.http.client.exceptions.HttpClientResponseException;
import io.micronaut.test.extensions.junit5.annotation.MicronautTest;
import org.junit.jupiter.api.Test;
import javax.inject.Inject;
import java.util.ArrayList;
import java.util.List;
import static org.junit.jupiter.api.Assertions.assertEquals;
import static org.junit.jupiter.api.Assertions.assertNotNull;
import static org.junit.jupiter.api.Assertions.assertThrows;
@MicronautTest (1)
public class GenreControllerTest {
@Inject
@Client("/")
HttpClient client; (2)
@Test
public void supplyAnInvalidOrderTriggersValidationFailure() {
HttpClientResponseException thrown = assertThrows(HttpClientResponseException.class, () -> {
client.toBlocking().exchange(HttpRequest.GET("/genres/list?order=foo"));
});
assertNotNull(thrown.getResponse());
assertEquals(HttpStatus.BAD_REQUEST, thrown.getStatus());
}
@Test
public void testFindNonExistingGenreReturns404() {
HttpClientResponseException thrown = assertThrows(HttpClientResponseException.class, () -> {
client.toBlocking().exchange(HttpRequest.GET("/genres/99"));
});
assertNotNull(thrown.getResponse());
assertEquals(HttpStatus.NOT_FOUND, thrown.getStatus());
}
@Test
public void testGenreCrudOperations() {
List<Long> genreIds = new ArrayList<>();
HttpRequest request = HttpRequest.POST("/genres", new GenreSaveCommand("DevOps")); (3)
HttpResponse response = client.toBlocking().exchange(request);
genreIds.add(entityId(response));
assertEquals(HttpStatus.CREATED, response.getStatus());
request = HttpRequest.POST("/genres", new GenreSaveCommand("Microservices")); (3)
response = client.toBlocking().exchange(request);
assertEquals(HttpStatus.CREATED, response.getStatus());
Long id = entityId(response);
genreIds.add(id);
request = HttpRequest.GET("/genres/" + id);
Genre genre = client.toBlocking().retrieve(request, Genre.class); (4)
assertEquals("Microservices", genre.getName());
request = HttpRequest.PUT("/genres", new GenreUpdateCommand(id, "Micro-services"));
response = client.toBlocking().exchange(request); (5)
assertEquals(HttpStatus.NO_CONTENT, response.getStatus());
request = HttpRequest.GET("/genres/" + id);
genre = client.toBlocking().retrieve(request, Genre.class);
assertEquals("Micro-services", genre.getName());
request = HttpRequest.GET("/genres/list");
List<Genre> genres = client.toBlocking().retrieve(request, Argument.of(List.class, Genre.class));
assertEquals(2, genres.size());
request = HttpRequest.POST("/genres/ex", new GenreSaveCommand("Microservices")); (3)
response = client.toBlocking().exchange(request);
assertEquals(HttpStatus.NO_CONTENT, response.getStatus());
request = HttpRequest.GET("/genres/list");
genres = client.toBlocking().retrieve(request, Argument.of(List.class, Genre.class));
assertEquals(2, genres.size());
request = HttpRequest.GET("/genres/list?max=1");
genres = client.toBlocking().retrieve(request, Argument.of(List.class, Genre.class));
assertEquals(1, genres.size());
assertEquals("DevOps", genres.get(0).getName());
request = HttpRequest.GET("/genres/list?max=1&order=desc&sort=name");
genres = client.toBlocking().retrieve(request, Argument.of(List.class, Genre.class));
assertEquals(1, genres.size());
assertEquals("Micro-services", genres.get(0).getName());
request = HttpRequest.GET("/genres/list?max=1&offset=10");
genres = client.toBlocking().retrieve(request, Argument.of(List.class, Genre.class));
assertEquals(0, genres.size());
// cleanup:
for (Long genreId : genreIds) {
request = HttpRequest.DELETE("/genres/" + genreId);
response = client.toBlocking().exchange(request);
assertEquals(HttpStatus.NO_CONTENT, response.getStatus());
}
}
protected Long entityId(HttpResponse response) {
String path = "/genres/";
String value = response.header(HttpHeaders.LOCATION);
if (value == null) {
return null;
}
int index = value.indexOf(path);
if (index != -1) {
return Long.valueOf(value.substring(index + path.length()));
}
return null;
}
}
1 | Annotate the class with @MicronautTest to let Micronaut starts the embedded server and inject the beans. More info: https://micronaut-projects.github.io/micronaut-test/latest/guide/index.html. |
2 | Inject the HttpClient bean in the application context. |
3 | Creating HTTP Requests is easy thanks to Micronaut’s fluid API. |
4 | If you care just about the object in the response use retrieve . |
5 | Sometimes, receiving just the object is not enough and you need information about the response. In this case, instead of retrieve you should use the exchange method. |
5. Testing the Application
To run the tests:
$ ./mvnw test
6. Running the Application
To run the application use the ./mvnw mn:run
command which will start the application on port 8080.
7. Using Postgresql
When running on production you want to use a real database instead of using H2. Let’s explain how to use Postgres.
After installing Docker, execute the following command to run a postgresql container:
docker run -it --rm \
-p 5432:5432 \
-e POSTGRES_USER=dbuser \
-e POSTGRES_PASSWORD=theSecretPassword \
-e POSTGRES_DB=micronaut \
postgres:11.5-alpine
Add Postgresql driver dependency:
<dependency>
<groupId>org.postgresql</groupId>
<artifactId>postgresql</artifactId>
<scope>runtime</scope>
</dependency>
To use postgresql, setup several environment variables which match those defined in application.yml
:
$ export JDBC_URL=jdbc:postgresql://localhost:5432/micronaut
$ export JDBC_USER=dbuser
$ export JDBC_PASSWORD=theSecretPassword
$ export JDBC_DRIVER=org.postgresql.Driver
Run the app again. If you take a close look to the output, you can see that the app uses postgresql:
..
...
08:40:02.746 [main] INFO org.hibernate.dialect.Dialect - HHH000400: Using dialect: org.hibernate.dialect.PostgreSQL10Dialect
....
Connect to your postgresql database, and you will see both genre
and book
tables.
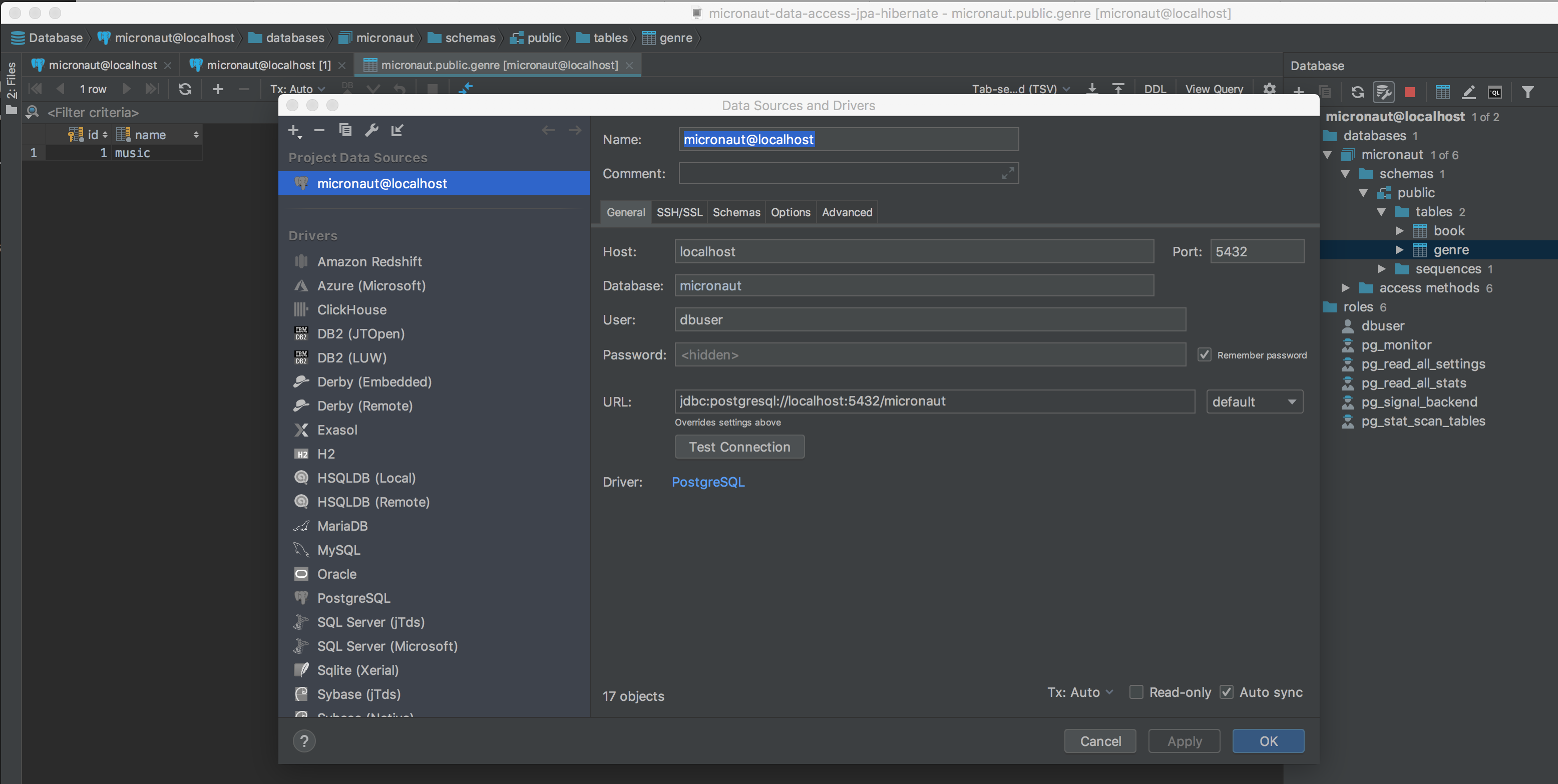
Save one genre, and your genre
table will now contain an entry.
curl -X "POST" "http://localhost:8080/genres" \
-H 'Content-Type: application/json; charset=utf-8' \
-d $'{ "name": "music" }'
8. Next steps
Read more about Configurations for Data Access section in the Micronaut documentation.
9. Help with Micronaut
Object Computing, Inc. (OCI) sponsored the creation of this Guide. A variety of consulting and support services are available.