mn create-app example.micronaut.micronautguide --build=maven --lang=kotlin
Micronaut HTTP Client
Learn how to use Micronaut low-level HTTP Client. Simplify your code with the declarative HTTP client.
Authors: Sergio del Amo, Iván López
Micronaut Version: 2.5.0
1. Getting Started
In this guide we are going to create a Micronaut app written in Kotlin to consume the GitHub API with the Micronaut HTTP Client.
2. What you will need
To complete this guide, you will need the following:
-
Some time on your hands
-
A decent text editor or IDE
-
JDK 1.8 or greater installed with
JAVA_HOME
configured appropriately
3. Solution
We recommend that you follow the instructions in the next sections and create the app step by step. However, you can go right to the completed example.
-
Download and unzip the source
4. Writing the App
Create an app using the Micronaut Command Line Interface or with Micronaut Launch.
If you don’t specify the --build argument, Gradle is used as a build tool. If you don’t specify the --lang argument, Java is used as a language.
|
The previous command creates a Micronaut app with the default package example.micronaut
in a folder named micronautguide
.
If you are using Java or Kotlin and IntelliJ IDEA, make sure you have enabled annotation processing.
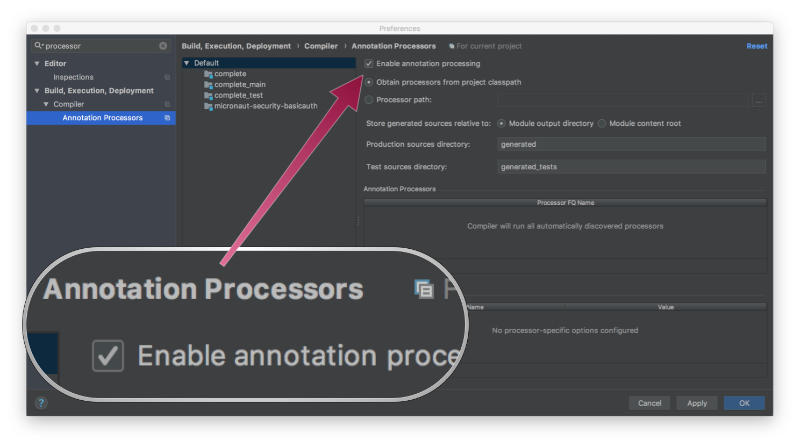
4.1. GitHub API
In this guide, you are going to consume the GitHub API from a Micronaut application.
In particular, we consume the List releases endpoint.
This returns a list of releases, which does not include regular Git tags that have not been associated with a release.
This API resource can be consumed by both authenticated and anonymous clients.
Initially, you will consume it anonymously, later we will discuss authentication.
Modify src/main/resources/application.yml
to create some configuration parameters.
github:
organization: micronaut-projects
repo: micronaut-core
To encapsulate type-safe configuration retrieval, we use a @ConfigurationProperties
object:
package example.micronaut
import io.micronaut.context.annotation.ConfigurationProperties
import io.micronaut.context.annotation.Requires
@ConfigurationProperties(GithubConfiguration.PREFIX)
@Requires(property = GithubConfiguration.PREFIX)
class GithubConfiguration {
var organization: String? = null
var repo: String? = null
var username: String? = null
var token: String? = null
companion object {
const val PREFIX = "github"
const val GITHUB_API_URL = "https://api.github.com"
}
}
In this guide, you are going to fetch Micronaut Core releases.
To consume the GitHub API, you will use Micronaut HTTP Client.
4.2. Low Level Client
Initially, you will create a Bean which uses the low-level Client API.
Create a POJO to parse the JSON response into an object:
package example.micronaut
import io.micronaut.core.annotation.Introspected
@Introspected
data class GithubRelease(val name: String, val url: String)
Create GithubLowLevelClient
:
package example.micronaut
import io.micronaut.core.type.Argument
import io.micronaut.http.HttpHeaders.ACCEPT
import io.micronaut.http.HttpHeaders.USER_AGENT
import io.micronaut.http.HttpRequest
import io.micronaut.http.client.RxHttpClient
import io.micronaut.http.client.annotation.Client
import io.micronaut.http.uri.UriBuilder
import io.reactivex.Maybe
import java.net.URI
import javax.inject.Singleton
@Singleton (1)
class GithubLowLevelClient(@param:Client(GithubConfiguration.GITHUB_API_URL) private val httpClient: RxHttpClient, (2)
configuration: GithubConfiguration) { (3)
private val uri: URI = UriBuilder.of("/repos")
.path(configuration.organization)
.path(configuration.repo)
.path("releases")
.build()
fun fetchReleases(): Maybe<List<GithubRelease>> {
val req: HttpRequest<*> = HttpRequest.GET<Any>(uri) (4)
.header(USER_AGENT, "Micronaut HTTP Client") (5)
.header(ACCEPT, "application/vnd.github.v3+json, application/json") (6)
val flowable = httpClient.retrieve(req, Argument.listOf(GithubRelease::class.java)) (7)
return flowable.firstElement() (8)
}
}
1 | Use javax.inject.Singleton to designate a class as a singleton. |
2 | Inject RxClient via constructor injection. |
3 | Inject the previously defined configuration parameters. |
4 | Creating HTTP Requests is easy thanks to Micronaut’s fluid API. |
5 | GitHub API requires to set the User-Agent header. |
6 | GitHub encourages to explicitly request the version 3 via the Accept header. With @Header , you add the Accept: application/vnd.github.v3+json HTTP header to every request. |
7 | Use retrieve to perform an HTTP request for the given request object and convert the full HTTP response’s body into the specified type. e.g. List<GithubRelease> . |
8 | The retrieve method returns a Flowable which has a firstElement method that returns the first emitted item or nothing |
Instead of retrieve we could have used jsonStream . You can use jsonStream() to stream arrays of type application/json or
JSON streams of type application/x-json-stream . If we use retrieve , such as in the previous code listing, the operation will not block.
However, it will not return until all the data has been received from the server. In the case of a JSON array that would be the whole array.
However, if you are interested in just the first element of the array, jsonStream provides a better alternative since it starts streaming data from the server without needing the whole response.
For example, jsonStream().firstElement() will only parse the first item in a JSON array. Hence it is more efficient.
|
4.3. Declarative Client
It is time to take a look at Micronaut’s support for declarative clients via the Client annotation.
Create GithubApiClient
which clearly illustrates how a declarative Micronaut HTTP Client, which is generated at compile-time, simplifies our code.
package example.micronaut
import io.micronaut.http.HttpHeaders.ACCEPT
import io.micronaut.http.HttpHeaders.USER_AGENT
import io.micronaut.http.annotation.Get
import io.micronaut.http.annotation.Header
import io.micronaut.http.annotation.Headers
import io.micronaut.http.client.annotation.Client
import io.reactivex.Flowable
@Client(GithubConfiguration.GITHUB_API_URL) (1)
@Headers(
Header(name = USER_AGENT, value = "Micronaut HTTP Client"), (2)
Header(name = ACCEPT, value = "application/vnd.github.v3+json, application/json") (3)
)
interface GithubApiClient {
@Get("/repos/\${github.organization}/\${github.repo}/releases") (4)
fun fetchReleases(): Flowable<GithubRelease?>? (5)
}
1 | URL of the remote service |
2 | GitHub API requires to set the User-Agent header. |
3 | GitHub encourages to explicitly request the version 3 via the Accept header. With @Header , you add the Accept: application/vnd.github.v3+json HTTP header to every request. |
4 | You can use configuration parameter interpolation when you define the path of the GET endpoint. |
5 | You can return reactive types, such as an RxJava Flowable . |
4.4. Controller
Create a Controller. It uses both (low-level and declarative clients). It showcases several Micronaut’s capabilities.
-
Micronaut supports any framework that implements Reactive Streams, including RxJava, and Reactor. Thus, you can easily and efficiently compose multiple HTTP client calls without blocking (which will limit the throughput and scalability of your application).
-
Micronaut enables you to consume/produce JSON Streams.
package example.micronaut
import io.micronaut.http.MediaType
import io.micronaut.http.annotation.Controller
import io.micronaut.http.annotation.Get
import io.reactivex.Flowable
import io.reactivex.Maybe
@Controller("/github") (1)
class GithubController(private val githubLowLevelClient: GithubLowLevelClient, (2)
private val githubApiClient: GithubApiClient) {
@Get("/releases-lowlevel") (3)
fun releasesWithLowLevelClient(): Maybe<List<GithubRelease>> { (4)
return githubLowLevelClient.fetchReleases()
}
@Get(uri = "/releases", produces = [MediaType.APPLICATION_JSON_STREAM]) (5)
fun fetchReleases(): Flowable<GithubRelease?>? { (6)
return githubApiClient.fetchReleases()
}
}
1 | The class is defined as a controller with the @Controller annotation mapped to the path /github . |
2 | Inject beans via constructor injection. |
3 | The @Get annotation is used to map the index method to all requests that use an HTTP GET |
4 | The releasesWithLowLevelClient returns a Maybe which may or may not emit an item. If an item is not emitted a 404 is returned. |
5 | In order to do JSON streaming you can declare a controller method that returns a application/x-json-stream of JSON objects. |
6 | You can return reactive types, such as an RxJava Flowable . |
4.5. Tests
Create a test which verifies both clients work as expected and the controller echoes the output of the GitHub API in a Reactive way.
package example.micronaut
import io.micronaut.core.type.Argument
import io.micronaut.http.HttpRequest
import io.micronaut.http.HttpStatus
import io.micronaut.http.client.RxStreamingHttpClient
import io.micronaut.http.client.annotation.Client
import io.micronaut.test.extensions.junit5.annotation.MicronautTest
import org.junit.jupiter.api.Assertions.assertEquals
import org.junit.jupiter.api.Assertions.assertNotNull
import org.junit.jupiter.api.Assertions.assertTrue
import org.junit.jupiter.api.Test
import java.util.stream.StreamSupport
import javax.inject.Inject
@MicronautTest (1)
class GithubControllerTest {
@Inject
@field:Client("/")
lateinit var client: RxStreamingHttpClient (2)
@Test
fun verifyGithubReleasesCanBeFetchedWithLowLevelHttpClient() {
//when:
val request: HttpRequest<Any> = HttpRequest.GET("/github/releases-lowlevel")
val rsp = client.toBlocking().exchange(request, (3)
Argument.listOf(GithubRelease::class.java)) (4)
//then: 'the endpoint can be accessed'
assertEquals(HttpStatus.OK, rsp.status) (5)
assertNotNull(rsp.body()) (6)
//when:
val releases = rsp.body()
//then:
for (name in expectedReleases) {
assertTrue(releases.stream().map(GithubRelease::name).anyMatch { anObject: String? -> name.equals(anObject) })
}
}
@Test
fun verifyGithubReleasesCanBeFetchedWithCompileTimeAutoGeneratedAtClient() {
//when:
val request: HttpRequest<Any> = HttpRequest.GET("/github/releases-lowlevel")
val githubReleaseStream = client.jsonStream(request, GithubRelease::class.java) (7)
val githubReleases = githubReleaseStream.blockingIterable()
//then:
for (name in expectedReleases) {
assertTrue(StreamSupport.stream(githubReleases.spliterator(), false)
.map(GithubRelease::name)
.anyMatch { anObject: String? -> name.equals(anObject) })
}
}
companion object {
private val expectedReleases = listOf("Micronaut 2.5.0", "Micronaut 2.4.4", "Micronaut 2.4.3")
}
}
1 | Annotate the class with @MicronautTest to let Micronaut starts the embedded server and inject the beans. More info: https://micronaut-projects.github.io/micronaut-test/latest/guide/index.html. |
2 | Inject the RxStreamingHttpClient bean in the application context. |
3 | Sometimes, receiving just the object is not enough, and you need information about the response. In this case, instead of retrieve you should use the exchange method. |
4 | Micronaut makes it easy to parse JSON into Java objects. |
5 | Use status to check the HTTP status code. |
6 | Use .body() to retrieve the parsed payload. |
7 | Use the jsonStream method, which returns a Flowable , to consume the endpoint which generates a JSON Stream. |
5. Testing the Application
To run the tests:
$ ./mvnw test
6. HTTP Client Filter
Often, you need to include the same HTTP headers or URL parameters in a set of requests against a third-party API or when
calling another Microservice. To simplify this, Micronaut includes the ability to define HttpClientFilter
classes that
are applied to all matching HTTP clients.
For a real world example, let us provide GitHub Authentication via an HttpClientFilter
. Follow the steps in
to create you own Personal Token.
Then you can use those credentials to access the GitHub API
using Basic Auth.
Create a Filter:
package example.micronaut
import io.micronaut.context.annotation.Requires
import io.micronaut.http.HttpResponse
import io.micronaut.http.MutableHttpRequest
import io.micronaut.http.annotation.Filter
import io.micronaut.http.filter.ClientFilterChain
import io.micronaut.http.filter.HttpClientFilter
import org.reactivestreams.Publisher
@Filter("/repos/**") (1)
@Requires(condition = GithubFilterCondition::class) (2)
class GithubFilter(val configuration: GithubConfiguration) : HttpClientFilter { (3)
override fun doFilter(request: MutableHttpRequest<*>, chain: ClientFilterChain): Publisher<out HttpResponse<*>?> {
return chain.proceed(request.basicAuth(configuration.username, configuration.token)) (4)
}
}
1 | Supply the pattern you want to match to the @Filter annotation. |
2 | Micronaut will not load the Bean unless configuration properties are set. |
3 | Constructor injection of the configuration parameters. |
4 | Enhance every request sent to GitHub API providing Basic Authentication. |
6.1. Configuration Parameters
Add your GitHub username
and token
to src/main/resource/application.yml
github:
organization: micronaut-projects
repo: micronaut-core
username: yourgithubusername
token: xxxxxxxxxxxx
Add to src/main/resources/logback.xml
, a logger to see Micronaut’s HTTP client output.
<logger name="io.micronaut.http.client" level="TRACE"/>
If you run again the tests, you will see the that the Filter is invoked and HTTP Basic Auth is used against GitHub API.
13:09:56.662 [default-nioEventLoopGroup-1-4] DEBUG i.m.h.client.netty.DefaultHttpClient - Sending HTTP GET to https://api.github.com/repos/micronaut-projects/micronaut-core/releases
13:09:56.663 [default-nioEventLoopGroup-1-4] TRACE i.m.h.client.netty.DefaultHttpClient - User-Agent: Micronaut HTTP Client
13:09:56.663 [default-nioEventLoopGroup-1-4] TRACE i.m.h.client.netty.DefaultHttpClient - Accept: application/json
13:09:56.663 [default-nioEventLoopGroup-1-4] TRACE i.m.h.client.netty.DefaultHttpClient - Authorization: Basic xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx
13:09:56.664 [default-nioEventLoopGroup-1-4] TRACE i.m.h.client.netty.DefaultHttpClient - host: api.github.com
7. Generate a Micronaut app’s Native Image with GraalVM
We are going to use GraalVM, the polyglot embeddable virtual machine, to generate a Native image of our Micronaut application.
Native images compiled with GraalVM ahead-of-time improve the startup time and reduce the memory footprint of JVM-based applications.
Use of GraalVM’s native-image tool is only supported in Java or Kotlin projects. Groovy relies heavily on
reflection which is only partially supported by GraalVM.
|
7.1. Native Image generation
The easiest way to install GraalVM is to use SDKMan.io.
# For Java 8
$ sdk install java 21.1.0.r8-grl
# For Java 11
$ sdk install java 21.1.0.r11-grl
You need to install the native-image
component which is not installed by default.
$ gu install native-image
To generate a native image using Maven run:
$ ./mvnw package -Dpackaging=native-image
The native image will be created in target/application
and can be run with ./target/application
.
8. Next steps
Visit Micronaut HTTP Client documentation to learn more.
9. Help with Micronaut
Object Computing, Inc. (OCI) sponsored the creation of this Guide. A variety of consulting and support services are available.