mn create-app example.micronaut.micronautguide --build=maven --lang=java
Creating your first Micronaut Graal application
Learn how to create a Hello World Micronaut GraalVM application.
Authors: Iván López, Sergio del Amo
Micronaut Version: 2.5.0
1. Getting Started
In this guide we are going to create a Micronaut app written in Java.
In this guide we are going to create a Micronaut application with GraalVM support.
2. What you will need
To complete this guide, you will need the following:
-
Some time on your hands
-
A decent text editor or IDE
-
JDK 1.8 or greater installed with
JAVA_HOME
configured appropriately
3. Solution
We recommend that you follow the instructions in the next sections and create the app step by step. However, you can go right to the completed example.
-
Download and unzip the source
4. Writing the App
Create an app using the Micronaut Command Line Interface or with Micronaut Launch.
If you don’t specify the --build argument, Gradle is used as a build tool. If you don’t specify the --lang argument, Java is used as a language.
|
The previous command creates a Micronaut app with the default package example.micronaut
in a folder named micronautguide
.
If you are using Java or Kotlin and IntelliJ IDEA, make sure you have enabled annotation processing.
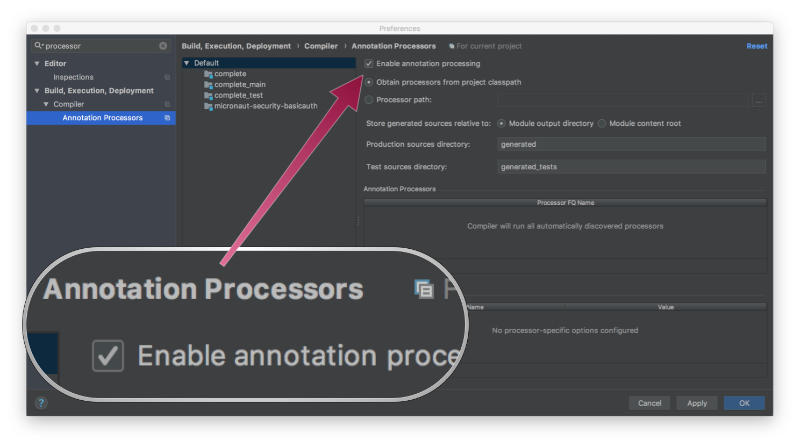
4.1. Service
Create a POJO Conference
:
package example.micronaut;
import io.micronaut.core.annotation.Introspected;
@Introspected (1)
public class Conference {
private String name;
public Conference(String name) {
this.name = name;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
1 | Annotate the class with @Introspected
to generate BeanIntrospection metadata at compilation time. This information is used to the render the POJO as json using Jackson
without using reflection. |
Create a Service:
package example.micronaut;
import javax.inject.Singleton;
import java.util.Arrays;
import java.util.List;
import java.util.Random;
@Singleton (1)
public class ConferenceService {
private static final List<Conference> CONFERENCES = Arrays.asList(
new Conference("Greach"),
new Conference("GR8Conf EU"),
new Conference("Micronaut Summit"),
new Conference("Devoxx Belgium"),
new Conference("Oracle Code One"),
new Conference("CommitConf"),
new Conference("Codemotion Madrid")
);
public Conference randomConf() { (2)
return CONFERENCES.get(new Random().nextInt(CONFERENCES.size()));
}
}
1 | Use javax.inject.Singleton to designate a class a a singleton. |
2 | Return a random conference. |
4.2. Controller
Create a Controller with a method that returns a Conference
. Micronaut will convert it automatically to JSON in the
response:
package example.micronaut;
import io.micronaut.http.annotation.Controller;
import io.micronaut.http.annotation.Get;
@Controller("/conferences") (1)
public class ConferenceController {
private final ConferenceService conferenceService;
public ConferenceController(ConferenceService conferenceService) { (2)
this.conferenceService = conferenceService;
}
@Get("/random") (3)
public Conference randomConf() { (4)
return conferenceService.randomConf();
}
}
1 | The class is defined as a controller with the @Controller annotation mapped to the path /conferences . |
2 | Constructor injection |
3 | The @Get annotation is used to map the index method to all requests that use an HTTP GET |
4 | Return a Conference . |
5. Generate a Micronaut app’s Native Image with GraalVM
We are going to use GraalVM, the polyglot embeddable virtual machine, to generate a Native image of our Micronaut application.
Native images compiled with GraalVM ahead-of-time improve the startup time and reduce the memory footprint of JVM-based applications.
Use of GraalVM’s native-image tool is only supported in Java or Kotlin projects. Groovy relies heavily on
reflection which is only partially supported by GraalVM.
|
5.1. Native Image generation
The easiest way to install GraalVM is to use SDKMan.io.
# For Java 8
$ sdk install java 21.1.0.r8-grl
# For Java 11
$ sdk install java 21.1.0.r11-grl
You need to install the native-image
component which is not installed by default.
$ gu install native-image
To generate a native image using Maven run:
$ ./mvnw package -Dpackaging=native-image
The native image will be created in target/application
and can be run with ./target/application
.
5.2. Creating native image inside Docker
The output following this approach is a docker image that runs the native image of your application. You don’t need to install any additional dependency.
$ ./mvnw package -Dpackaging=docker-native
5.3. Running the native image
Execute the application either by running the executable or starting the docker container.
10:29:46.845 [main] INFO io.micronaut.runtime.Micronaut - Startup completed in 12ms. Server Running: http://localhost:8080
We can see that the application starts in only 12ms.
5.4. Sending a request
Start the application either using Docker or the native executable. You can run a few cURL requests to test the application:
complete $ time curl localhost:8080/conferences/random
{"name":"Greach"}
real 0m0.016s
user 0m0.005s
sys 0m0.004s
complete $ time curl localhost:8080/conferences/random
{"name":"GR8Conf EU"}
real 0m0.014s
user 0m0.005s
sys 0m0.004s
For more information about the new plugins take a look at the Micronaut Gradle plugin and Micronaut Maven Plugin documentation. |
6. Next steps
Read more about GraalVM Support inside Micronaut.
7. Help with Micronaut
Object Computing, Inc. (OCI) sponsored the creation of this Guide. A variety of consulting and support services are available.