mn create-app example.micronaut.micronautguide --build=gradle --lang=kotlin
Micronaut Cache
Learn how to use Micronaut’s caching annotations
Authors: Sergio del Amo
Micronaut Version: 2.5.0
1. Getting Started
In this guide, you are going to use Micronaut’s caching annotations to speed up your application.
2. What you will need
To complete this guide, you will need the following:
-
Some time on your hands
-
A decent text editor or IDE
-
JDK 1.8 or greater installed with
JAVA_HOME
configured appropriately
3. Solution
We recommend that you follow the instructions in the next sections and create the app step by step. However, you can go right to the completed example.
-
Download and unzip the source
4. Writing the App
Create an app using the Micronaut Command Line Interface or with Micronaut Launch.
If you don’t specify the --build argument, Gradle is used as a build tool. If you don’t specify the --lang argument, Java is used as a language.
|
The previous command creates a Micronaut app with the default package example.micronaut
in a folder named micronautguide
.
If you are using Java or Kotlin and IntelliJ IDEA, make sure you have enabled annotation processing.
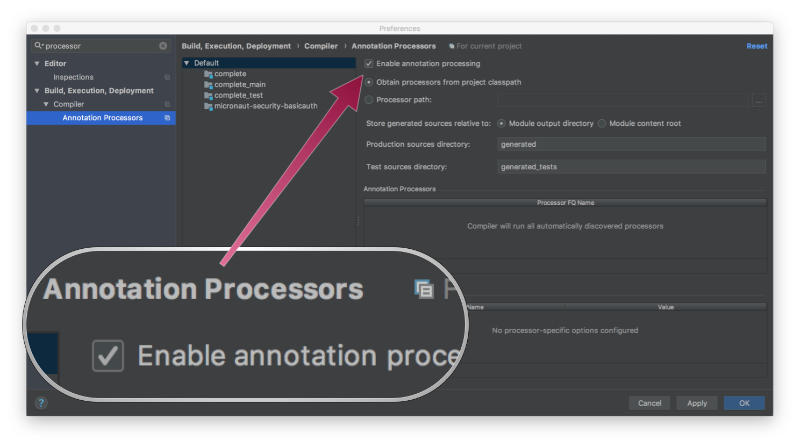
4.1. Configure the Application
In this sample application, we cache news headlines. Add Micronaut Caffeine Cache dependency which adds support for cache using Caffeine.
implementation("io.micronaut.cache:micronaut-cache-caffeine")
Configure your caches in application.yml
:
micronaut:
caches:
headlines: (1)
charset: 'UTF-8'
1 | Configure a cache called headlines . |
Check the properties (maximum-size , expire-after-write and expire-after-access ) to configure the size and expiration of your caches. It is important to keep the caches' size under control.
|
4.2. Micronaut Cache Api
Imagine a service which retrieves headlines for a given month. This operation may be expensive and you may want to cache it.
package example.micronaut
import io.micronaut.cache.annotation.CacheConfig
import io.micronaut.cache.annotation.CacheInvalidate
import io.micronaut.cache.annotation.CachePut
import io.micronaut.cache.annotation.Cacheable
import java.time.Month
import java.util.concurrent.TimeUnit
import javax.inject.Singleton
@Singleton (1)
@CacheConfig("headlines") (2)
open class NewsService {
val headlines = mutableMapOf(
Month.NOVEMBER to listOf("Micronaut Graduates to Trial Level in Thoughtworks technology radar Vol.1",
"Micronaut AOP: Awesome flexibility without the complexity"),
Month.OCTOBER to listOf("Micronaut AOP: Awesome flexibility without the complexity"))
@Cacheable (3)
open fun headlines(month: Month): List<String>? {
return try {
TimeUnit.SECONDS.sleep(3) (4)
headlines[month]
} catch (e: InterruptedException) {
null
}
}
@CachePut(parameters = ["month"]) (5)
open fun addHeadline(month: Month, headline: String): List<String>? {
val l = headlines.getOrDefault(month, emptyList()).toMutableList()
l.add(headline)
headlines[month] = l
return headlines[month]
}
@CacheInvalidate(parameters = ["month"]) (6)
open fun removeHeadline(month: Month, headline: String?) {
val l = headlines.getOrDefault(month, emptyList()).toMutableList()
l.remove(headline)
headlines[month] = l
}
}
1 | To register a Singleton in Micronaut’s application context annotate your class with javax.inject.Singleton . |
2 | Specifies the cache name headlines to store cache operation values in. |
3 | Indicates a method is cacheable. The cache name headlines specified in @CacheConfig is used. Since the method has only one parameter, you don’t need to specify the month parameters attribute of the the annation. |
4 | Emulate an expensive operation by sleeping for several seconds. |
5 | The return value is cached with name headlines for the supplied month . The method invocation is never skipped even if the cache headlines for the supplied month already existed. |
6 | Method invocation causes the invalidation of the cache headlines for the supplied month . |
If you don’t annotate the class with @CacheConfig , specify the cache name in the cache annotations. E.g. @Cacheable(value = "headlines", parameters = {"month"})
|
4.3. Test the Cache
We can verify that the cache works as expected:
package example.micronaut
import io.micronaut.test.extensions.junit5.annotation.MicronautTest
import org.junit.jupiter.api.Assertions
import org.junit.jupiter.api.MethodOrderer.OrderAnnotation
import org.junit.jupiter.api.Order
import org.junit.jupiter.api.Test
import org.junit.jupiter.api.TestMethodOrder
import org.junit.jupiter.api.Timeout
import java.time.Month
import javax.inject.Inject
@TestMethodOrder(OrderAnnotation::class) (1)
@MicronautTest (2)
internal class NewsServiceTest {
@Inject
lateinit var newsService : NewsService (3)
@Timeout(4) (4)
@Test
@Order(1) (5)
fun firstInvocationOfNovemberDoesNotHitCache() {
val headlines = newsService.headlines(Month.NOVEMBER)
Assertions.assertEquals(2, headlines!!.size)
}
@Timeout(1) (4)
@Test
@Order(2) (5)
fun secondInvocationOfNovemberHitsCache() {
val headlines = newsService.headlines(Month.NOVEMBER)
Assertions.assertEquals(2, headlines!!.size)
}
@Timeout(4) (4)
@Test
@Order(3) (5)
fun firstInvocationOfOctoberDoesNotHitCache() {
val headlines = newsService.headlines(Month.OCTOBER)
Assertions.assertEquals(1, headlines!!.size)
}
@Timeout(1) (4)
@Test
@Order(4) (5)
fun secondInvocationOfOctoberHitsCache() {
val headlines = newsService.headlines(Month.OCTOBER)
Assertions.assertEquals(1, headlines!!.size)
}
@Timeout(1) (4)
@Test
@Order(5) (5)
fun addingAHeadlineToNovemberUpdatesCache() {
val headlines = newsService.addHeadline(Month.NOVEMBER, "Micronaut 1.3 Milestone 1 Released")
Assertions.assertEquals(3, headlines!!.size)
}
@Timeout(1) (4)
@Test
@Order(6) (5)
fun novemberCacheWasUpdatedByCachePutAndThusTheValueIsRetrievedFromTheCache() {
val headlines = newsService.headlines(Month.NOVEMBER)
Assertions.assertEquals(3, headlines!!.size)
}
@Timeout(1) (4)
@Test
@Order(7) (5)
fun invalidateNovemberCacheWithCacheInvalidate() {
Assertions.assertDoesNotThrow { newsService.removeHeadline(Month.NOVEMBER, "Micronaut 1.3 Milestone 1 Released") }
}
@Timeout(1) (4)
@Test
@Order(8) (5)
fun octoberCacheIsStillValid() {
val headlines = newsService.headlines(Month.OCTOBER)
Assertions.assertEquals(1, headlines!!.size)
}
@Timeout(4) (4)
@Test
@Order(9) (5)
fun novemberCacheWasInvalidated() {
val headlines = newsService.headlines(Month.NOVEMBER)
Assertions.assertEquals(2, headlines!!.size)
}
}
1 | Used to configure the test method execution order for the annotated test class. |
2 | Annotation used to define a Micronaut test |
3 | Inject NewsService bean. |
4 | Timeout annotation fails a test if its execution exceeds a given duration. It helps us verify that we are leaveraging the cache. |
5 | Used to configure the order in which the test method should executed relative to other tests in the class. |
4.4. Controller
Create a controller which engages the previous service:
package example.micronaut
import io.micronaut.core.annotation.Introspected
import java.time.Month
@Introspected
data class News(val month: Month, val headlines: List<String>)
package example.micronaut
import io.micronaut.http.annotation.Controller
import io.micronaut.http.annotation.Get
import java.time.Month
@Controller
class NewsController(val newsService: NewsService) {
@Get("/{month}")
fun index(month: Month): News {
return News(month, newsService.headlines(month).orEmpty())
}
}
Add a test:
package example.micronaut
import io.micronaut.http.HttpRequest
import io.micronaut.http.client.HttpClient
import io.micronaut.http.client.annotation.Client
import io.micronaut.http.uri.UriBuilder
import io.micronaut.test.extensions.junit5.annotation.MicronautTest
import org.junit.jupiter.api.Assertions
import org.junit.jupiter.api.Test
import org.junit.jupiter.api.Timeout
import java.time.Month
import javax.inject.Inject
@MicronautTest
class NewsControllerTest {
@Inject
@field:Client("/")
lateinit var client: HttpClient
@Timeout(5) (1)
@Test
fun fetchingOctoberHeadlinesUsesCache() {
val request: HttpRequest<Any> = HttpRequest.GET(UriBuilder.of("/").path(Month.OCTOBER.name).build())
var news: News = client.toBlocking().retrieve(request, News::class.java)
val expected = "Micronaut AOP: Awesome flexibility without the complexity"
Assertions.assertEquals(listOf(expected), news.headlines)
news = client.toBlocking().retrieve(request, News::class.java)
Assertions.assertEquals(listOf(expected), news.headlines)
}
}
1 | We call the endpoint twice and verify with the @Timeout annotation that the cache is being used. |
5. Running the Application
To run the application use the ./gradlew run
command which will start the application on port 8080.
6. Generate a Micronaut app’s Native Image with GraalVM
We are going to use GraalVM, the polyglot embeddable virtual machine, to generate a Native image of our Micronaut application.
Native images compiled with GraalVM ahead-of-time improve the startup time and reduce the memory footprint of JVM-based applications.
Use of GraalVM’s native-image tool is only supported in Java or Kotlin projects. Groovy relies heavily on
reflection which is only partially supported by GraalVM.
|
6.1. Native Image generation
The easiest way to install GraalVM is to use SDKMan.io.
# For Java 8
$ sdk install java 21.1.0.r8-grl
# For Java 11
$ sdk install java 21.1.0.r11-grl
You need to install the native-image
component which is not installed by default.
$ gu install native-image
To generate a native image using Gradle run:
$ ./gradlew nativeImage
The native image will be created in build/native-image/application
and can be run with ./build/native-image/application
It is also possible to customize the name of the native image or pass additional parameters to GraalVM:
nativeImage {
args('--verbose')
imageName('mn-graalvm-application') (1)
}
1 | The native image name will now be mn-graalvm-application |
You should be able to execute curl localhost:8080/NOVEMBER
and see results
7. Next steps
Read about Micronaut’s Cache Advice. Moreover, check the Micronaut Cache project for more information.
8. Help with Micronaut
Object Computing, Inc. (OCI) sponsored the creation of this Guide. A variety of consulting and support services are available.