mn create-app example.micronaut.micronautguide --build=maven --lang=java
Micronaut AWS Parameter Store
Learn how to use AWS Parameter for Configuration Discovery in a Micronaut application.
Authors: Pavol Gressa, Sergio del Amo
Micronaut Version: 2.5.0
1. Getting Started
In this guide, you are going to use AWS Parameter Store in Micronaut Application to drive configuration.
2. What you will need
To complete this guide, you will need the following:
-
Some time on your hands
-
A decent text editor or IDE
-
JDK 1.8 or greater installed with
JAVA_HOME
configured appropriately
3. Solution
We recommend that you follow the instructions in the next sections and create the app step by step. However, you can go right to the completed example.
-
Download and unzip the source
4. Writing the App
Create an app using the Micronaut Command Line Interface or with Micronaut Launch.
If you don’t specify the --build argument, Gradle is used as a build tool. If you don’t specify the --lang argument, Java is used as a language.
|
The previous command creates a Micronaut app with the default package example.micronaut
in a folder named micronautguide
.
If you are using Java or Kotlin and IntelliJ IDEA, make sure you have enabled annotation processing.
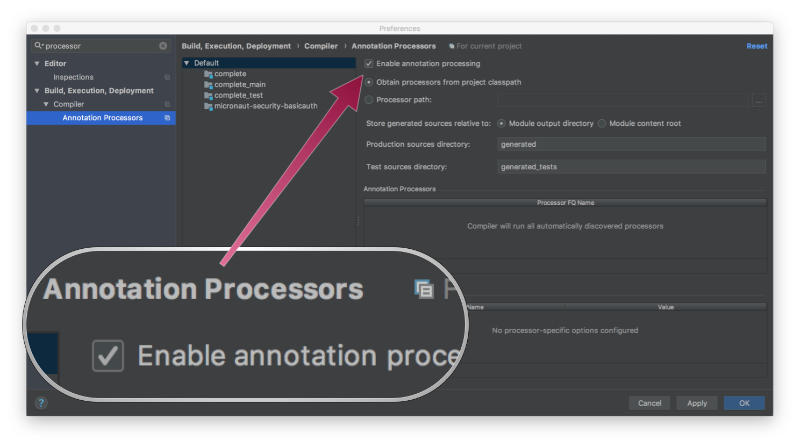
4.1. Controller
First, create an interface to encapsulate the Value-added Tax rate of a country.
package example.micronaut;
import io.micronaut.core.annotation.NonNull;
import java.math.BigDecimal;
public interface Vat {
@NonNull
String getCountry();
@NonNull
BigDecimal getRate();
}
Create a GET endpoint /vat
. It returns the Value-added Tax rate configured in our app.
package example.micronaut;
import io.micronaut.http.MediaType;
import io.micronaut.http.annotation.Controller;
import io.micronaut.http.annotation.Get;
import io.micronaut.http.annotation.Produces;
import java.math.BigDecimal;
@Controller (1)
public class VatController {
private final Vat vat;
public VatController(Vat vat) { (2)
this.vat = vat;
}
@Produces(MediaType.TEXT_PLAIN) (3)
@Get("/vat") (4)
public BigDecimal index() {
return vat.getRate();
}
}
1 | The class is defined as a controller with the @Controller annotation. |
2 | Use constructor injection to inject a bean of type Vat |
3 | Since it does not return JSON, set the media type to text/plain |
4 | The @Get annotation is used to map the index method to all requests that use an HTTP GET to /vat |
4.2. Configuration
package example.micronaut;
import io.micronaut.context.annotation.ConfigurationProperties;
import io.micronaut.core.annotation.NonNull;
import javax.validation.constraints.NotBlank;
import javax.validation.constraints.NotNull;
import java.math.BigDecimal;
@ConfigurationProperties("vat") (1)
public class VatConfiguration implements Vat { (2)
@NonNull
@NotNull
private BigDecimal rate;
@NotBlank
@NotNull
private String country;
@Override
@NonNull
public BigDecimal getRate() {
return rate;
}
public void setRate(@NonNull BigDecimal rate) {
this.rate = rate;
}
@Override
public String getCountry() {
return country;
}
public void setCountry(String country) {
this.country = country;
}
}
1 | The @ConfigurationProperties annotation takes the configuration prefix |
2 | By implementing the interface, we can inject a bean of type Vat in the previous Controller’s constructor. |
4.3. Test
Write a test which verifies a GET /vat
endpoint returns the value set via configuration.
package example.micronaut;
import io.micronaut.http.client.HttpClient;
import io.micronaut.http.client.annotation.Client;
import io.micronaut.test.extensions.junit5.annotation.MicronautTest;
import org.junit.jupiter.api.Assertions;
import org.junit.jupiter.api.Test;
import io.micronaut.context.annotation.Property;
import javax.inject.Inject;
import java.math.BigDecimal;
@MicronautTest (1)
@Property(name = "vat.country", value = "Switzerland") (2)
@Property(name = "vat.rate", value = "7.7") (2)
public class VatControllerTest {
@Inject
@Client("/")
public HttpClient httpClient;
@Test
void vatExposesTheValueAddedTaxRate() {
Assertions.assertEquals(httpClient.toBlocking().retrieve("/vat", BigDecimal.class), new BigDecimal("7.7"));
}
}
1 | Annotate the class with @MicronautTest so Micronaut will initialize the application context and the embedded server. |
2 | Annotate the class with @Property to supply configuration to the test. |
4.4. Dependency
To use AWS Parameter Store to drive configuration add the following dependency:
<dependency>
<groupId>io.micronaut.aws</groupId>
<artifactId>micronaut-aws-parameter-store</artifactId>
<scope>compile</scope>
</dependency>
4.5. Bootstrap.yml
To enable distributed configuration a src/main/resources/bootstrap.yml configuration file must be created and configured to use Parameter Store:
micronaut:
application:
name: micronautguide
config-client:
enabled: true
aws.client.system-manager.parameterstore.enabled: true
-
micronaut.application.name
name will be part of the parameter name in AWS Parameter Store. -
Set
microanut.config-client.enabled: true
which is used read and resolve configuration from distributed sources. -
With
aws.client.system-manager.parameterstore.enabled
enable distributed configuration with AWS Parameter Store.
4.6. Logging
Add loggers to get a more verbose output:
...
<logger name="io.micronaut.discovery" level="TRACE"/>
<logger name="io.micronaut.aws" level="TRACE"/>
</configuration>
5. Populate Configuration at AWS Parameter Store
You can use the AWS CLI or the AWS Console to populate your configuration.
5.1. Set Parameters via AWS Console
Visit AWS Parameter Store service.
Create two parameters:
Set the environment to use ec2
, the programmatic access and region:
$ export MICRONAUT_ENVIRONMENTS=ec2
$ export AWS_ACCESS_KEY_ID=xxx
$ export AWS_SECRET_ACCESS_KEY=xxx
$ export AWS_REGION=us-east-1
To run the application use the ./mvnw mn:run
command which will start the application on port 8080.
You will get an output such as:
06:41:44.364 [main] INFO i.m.context.env.DefaultEnvironment - Established active environments: [ec2]
06:41:44.377 [main] INFO i.m.context.env.DefaultEnvironment - Established active environments: [ec2]
06:41:44.489 [main] INFO i.m.context.DefaultBeanContext - Reading Startup environment from bootstrap.yml
06:41:46.425 [main] DEBUG i.m.d.c.c.DistributedPropertySourceLocator - Resolving configuration sources from client: compositeConfigurationClient(AWS Parameter Store)
06:41:47.811 [main] TRACE i.m.d.a.p.AWSParameterStoreConfigClient - parameterBasePath=/config/application no parameters found
06:41:47.813 [main] TRACE i.m.d.a.p.AWSParameterStoreConfigClient - Retrieving parameters by path /config/application, pagination requested: false
06:41:47.961 [main] TRACE i.m.d.a.p.AWSParameterStoreConfigClient - parameterBasePath=/config/application no parameters found
06:41:47.961 [main] TRACE i.m.d.a.p.AWSParameterStoreConfigClient - parameterBasePath=/config/application no parameters found
06:41:48.084 [main] TRACE i.m.d.a.p.AWSParameterStoreConfigClient - parameterBasePath=/config/micronautguide no parameters found
06:41:48.085 [main] TRACE i.m.d.a.p.AWSParameterStoreConfigClient - Retrieving parameters by path /config/micronautguide, pagination requested: false
06:41:48.222 [main] TRACE i.m.d.a.p.AWSParameterStoreConfigClient - parameterBasePath=/config/micronautguide no parameters found
06:41:48.224 [main] TRACE i.m.d.a.p.AWSParameterStoreConfigClient - Converted {vat.rate=21, vat.country=Spain}
06:41:48.224 [main] TRACE i.m.d.a.p.AWSParameterStoreConfigClient - param found: parameterBasePath=/config/micronautguide parameter=vat.rate
06:41:48.224 [main] TRACE i.m.d.a.p.AWSParameterStoreConfigClient - param found: parameterBasePath=/config/micronautguide parameter=vat.country
06:41:48.359 [main] TRACE i.m.d.a.p.AWSParameterStoreConfigClient - parameterBasePath=/config/application_ec2 no parameters found
06:41:48.360 [main] TRACE i.m.d.a.p.AWSParameterStoreConfigClient - Retrieving parameters by path /config/application_ec2, pagination requested: false
06:41:48.485 [main] TRACE i.m.d.a.p.AWSParameterStoreConfigClient - parameterBasePath=/config/application_ec2 no parameters found
06:41:48.485 [main] TRACE i.m.d.a.p.AWSParameterStoreConfigClient - parameterBasePath=/config/application_ec2 no parameters found
06:41:48.607 [main] TRACE i.m.d.a.p.AWSParameterStoreConfigClient - parameterBasePath=/config/micronautguide_ec2 no parameters found
06:41:48.607 [main] TRACE i.m.d.a.p.AWSParameterStoreConfigClient - Retrieving parameters by path /config/micronautguide_ec2, pagination requested: false
06:41:48.732 [main] TRACE i.m.d.a.p.AWSParameterStoreConfigClient - parameterBasePath=/config/micronautguide_ec2 no parameters found
06:41:48.733 [main] TRACE i.m.d.a.p.AWSParameterStoreConfigClient - parameterBasePath=/config/micronautguide_ec2 no parameters found
06:41:48.733 [main] TRACE i.m.d.a.p.AWSParameterStoreConfigClient - source=micronautguide got priority=-98
06:41:48.734 [main] INFO i.m.d.c.c.DistributedPropertySourceLocator - Resolved 1 configuration sources from client: compositeConfigurationClient(AWS Parameter Store)
06:41:49.083 [main] INFO io.micronaut.runtime.Micronaut - Startup completed in 4874ms. Server Running: http://localhost:8080
You should be able to execute curl -i localhost:8080/vat
and see 21
.
5.2. Set Parameters via AWS CLI
Install AWS CLI.
Once you configure the CLI a region and user AWS access ID and secret key is configured.
Run the following commands to
$ aws ssm put-parameter --name /config/micronautguide/vat/country --value=Spain --type String
$ aws ssm put-parameter --name /config/micronautguide/vat/rate --value=21.0 --type String
Set the environment to use ec2
.
$ export MICRONAUT_ENVIRONMENTS=ec2
The AWS region and programmatic access set via the CLI will be used.
To run the application use the ./mvnw mn:run
command which will start the application on port 8080.
You will get an output such as:
06:41:44.364 [main] INFO i.m.context.env.DefaultEnvironment - Established active environments: [ec2]
06:41:44.377 [main] INFO i.m.context.env.DefaultEnvironment - Established active environments: [ec2]
06:41:44.489 [main] INFO i.m.context.DefaultBeanContext - Reading Startup environment from bootstrap.yml
06:41:46.425 [main] DEBUG i.m.d.c.c.DistributedPropertySourceLocator - Resolving configuration sources from client: compositeConfigurationClient(AWS Parameter Store)
06:41:47.811 [main] TRACE i.m.d.a.p.AWSParameterStoreConfigClient - parameterBasePath=/config/application no parameters found
06:41:47.813 [main] TRACE i.m.d.a.p.AWSParameterStoreConfigClient - Retrieving parameters by path /config/application, pagination requested: false
06:41:47.961 [main] TRACE i.m.d.a.p.AWSParameterStoreConfigClient - parameterBasePath=/config/application no parameters found
06:41:47.961 [main] TRACE i.m.d.a.p.AWSParameterStoreConfigClient - parameterBasePath=/config/application no parameters found
06:41:48.084 [main] TRACE i.m.d.a.p.AWSParameterStoreConfigClient - parameterBasePath=/config/micronautguide no parameters found
06:41:48.085 [main] TRACE i.m.d.a.p.AWSParameterStoreConfigClient - Retrieving parameters by path /config/micronautguide, pagination requested: false
06:41:48.222 [main] TRACE i.m.d.a.p.AWSParameterStoreConfigClient - parameterBasePath=/config/micronautguide no parameters found
06:41:48.224 [main] TRACE i.m.d.a.p.AWSParameterStoreConfigClient - Converted {vat.rate=21, vat.country=Spain}
06:41:48.224 [main] TRACE i.m.d.a.p.AWSParameterStoreConfigClient - param found: parameterBasePath=/config/micronautguide parameter=vat.rate
06:41:48.224 [main] TRACE i.m.d.a.p.AWSParameterStoreConfigClient - param found: parameterBasePath=/config/micronautguide parameter=vat.country
06:41:48.359 [main] TRACE i.m.d.a.p.AWSParameterStoreConfigClient - parameterBasePath=/config/application_ec2 no parameters found
06:41:48.360 [main] TRACE i.m.d.a.p.AWSParameterStoreConfigClient - Retrieving parameters by path /config/application_ec2, pagination requested: false
06:41:48.485 [main] TRACE i.m.d.a.p.AWSParameterStoreConfigClient - parameterBasePath=/config/application_ec2 no parameters found
06:41:48.485 [main] TRACE i.m.d.a.p.AWSParameterStoreConfigClient - parameterBasePath=/config/application_ec2 no parameters found
06:41:48.607 [main] TRACE i.m.d.a.p.AWSParameterStoreConfigClient - parameterBasePath=/config/micronautguide_ec2 no parameters found
06:41:48.607 [main] TRACE i.m.d.a.p.AWSParameterStoreConfigClient - Retrieving parameters by path /config/micronautguide_ec2, pagination requested: false
06:41:48.732 [main] TRACE i.m.d.a.p.AWSParameterStoreConfigClient - parameterBasePath=/config/micronautguide_ec2 no parameters found
06:41:48.733 [main] TRACE i.m.d.a.p.AWSParameterStoreConfigClient - parameterBasePath=/config/micronautguide_ec2 no parameters found
06:41:48.733 [main] TRACE i.m.d.a.p.AWSParameterStoreConfigClient - source=micronautguide got priority=-98
06:41:48.734 [main] INFO i.m.d.c.c.DistributedPropertySourceLocator - Resolved 1 configuration sources from client: compositeConfigurationClient(AWS Parameter Store)
06:41:49.083 [main] INFO io.micronaut.runtime.Micronaut - Startup completed in 4874ms. Server Running: http://localhost:8080
You should be able to execute curl -i localhost:8080/vat
and see 21
.
5.3. Leverage environments
AWS Parameter Store is specially powerful in combination with Micronaut environments. Imagine we deploy our application also for Switzerland. We can have an environment named ch
and load different configuration based on the environment. Create two parameters:
$ aws ssm put-parameter --name /config/micronautguide_ch/vat/country --value=Switzerland --type String
$ aws ssm put-parameter --name /config/micronautguide_ch/vat/rate --value=7.7 --type String
Set the environment to use ec2
and ch
.
$ export MICRONAUT_ENVIRONMENTS=ec2,ch
Run the app and you should be able to execute curl -i localhost:8080/vat
and see 7.7
.
6. Next steps
Read about Micronaut’s AWS Parameter Store integration.
Read about AWS System Manager Parameter Store
7. Help with Micronaut
Object Computing, Inc. (OCI) sponsored the creation of this Guide. A variety of consulting and support services are available.