mn create-app example.micronaut.micronautguide --build=gradle --lang=kotlin
Table of Contents
Creating your first Micronaut app
Learn how to create a Hello World Micronaut app with a controller and a functional test.
Authors: Iván López, Sergio del Amo
Micronaut Version: 2.5.0
1. Getting Started
In this guide we are going to create a Micronaut app written in Kotlin.
2. What you will need
To complete this guide, you will need the following:
-
Some time on your hands
-
A decent text editor or IDE
-
JDK 1.8 or greater installed with
JAVA_HOME
configured appropriately
3. Solution
We recommend that you follow the instructions in the next sections and create the app step by step. However, you can go right to the completed example.
-
Download and unzip the source
4. Writing the App
Create an app using the Micronaut Command Line Interface or with Micronaut Launch.
If you don’t specify the --build argument, Gradle is used as a build tool. If you don’t specify the --lang argument, Java is used as a language.
|
The previous command creates a Micronaut app with the default package example.micronaut
in a folder named micronautguide
.
If you are using Java or Kotlin and IntelliJ IDEA, make sure you have enabled annotation processing.
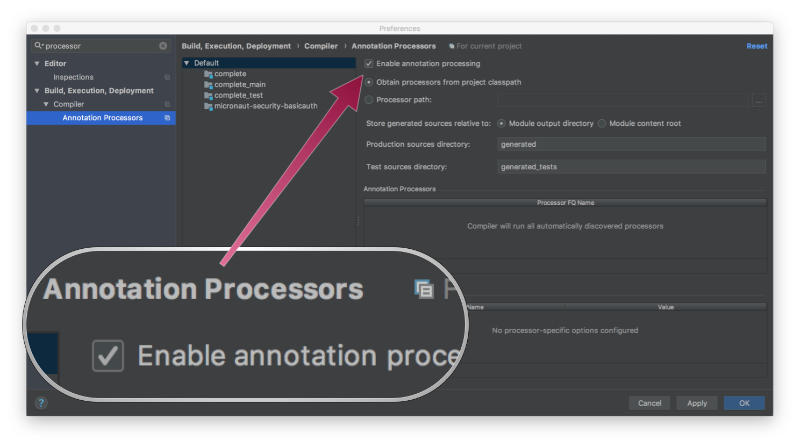
4.1. Application
Application.kt
is used when running the application via Gradle or via deployment. You can also run the main class directly within your IDE if it is configured correctly.
package example.micronaut
import io.micronaut.runtime.Micronaut.*
fun main(args: Array<String>) {
build()
.args(*args)
.packages("example.micronaut")
.start()
}
4.2. Controller
In order to create a microservice that responds with "Hello World" you first need a controller.
Create a Controller:
package example.micronaut
import io.micronaut.http.MediaType
import io.micronaut.http.annotation.Controller
import io.micronaut.http.annotation.Get
import io.micronaut.http.annotation.Produces
@Controller("/hello") (1)
class HelloController {
@Get("/") (2)
@Produces(MediaType.TEXT_PLAIN) (3)
fun index(): String {
return "Hello World" (4)
}
}
1 | The class is defined as a controller with the @Controller annotation mapped to the path /hello |
2 | The @Get annotation is used to map the index method to all requests that use an HTTP GET |
3 | By default a Micronaut’s response uses application/json as Content-Type . We are returning a String not a JSON object. Because of that, we set it to text/plain . |
4 | A String "Hello World" is returned as the result |
4.3. Test
Create a test which verifies that when you do a GET request to /hello
you get Hello World
as a response:
package example.micronaut
import io.micronaut.http.HttpRequest
import io.micronaut.http.client.RxHttpClient
import io.micronaut.http.client.annotation.Client
import io.micronaut.test.extensions.junit5.annotation.MicronautTest
import org.junit.jupiter.api.Assertions
import org.junit.jupiter.api.Test
import javax.inject.Inject
@MicronautTest (1)
class HelloControllerTest {
@Inject
@field:Client("/") (2)
lateinit var client : RxHttpClient
@Test
fun testHello() {
val request: HttpRequest<Any> = HttpRequest.GET("/hello") (3)
val body = client.toBlocking().retrieve(request)
Assertions.assertNotNull(body)
Assertions.assertEquals("Hello World", body)
}
}
1 | Annotate the class with @MicronautTest so Micronaut will initialize the application context and the embedded server. |
2 | Inject the RxHttpClient bean. It is used the execute an HTTP call to the controller. |
3 | Creating HTTP Requests is easy thanks to Micronaut’s fluid API. |
5. Testing the Application
To run the tests:
$ ./gradlew test
$ open build/reports/tests/test/index.html
6. Running the Application
To run the application use the ./gradlew run
command which will start the application on port 8080.
7. Generate a Micronaut app’s Native Image with GraalVM
We are going to use GraalVM, the polyglot embeddable virtual machine, to generate a Native image of our Micronaut application.
Native images compiled with GraalVM ahead-of-time improve the startup time and reduce the memory footprint of JVM-based applications.
Use of GraalVM’s native-image tool is only supported in Java or Kotlin projects. Groovy relies heavily on
reflection which is only partially supported by GraalVM.
|
7.1. Native Image generation
The easiest way to install GraalVM is to use SDKMan.io.
# For Java 8
$ sdk install java 21.1.0.r8-grl
# For Java 11
$ sdk install java 21.1.0.r11-grl
You need to install the native-image
component which is not installed by default.
$ gu install native-image
To generate a native image using Gradle run:
$ ./gradlew nativeImage
The native image will be created in build/native-image/application
and can be run with ./build/native-image/application
It is also possible to customize the name of the native image or pass additional parameters to GraalVM:
nativeImage {
args('--verbose')
imageName('mn-graalvm-application') (1)
}
1 | The native image name will now be mn-graalvm-application |
You can execute the endpoint exposed by the native image:
$ curl localhost:8080/hello
Hello World
8. Next steps
Read more about Micronaut testing.
9. Help with Micronaut
Object Computing, Inc. (OCI) sponsored the creation of this Guide. A variety of consulting and support services are available.