Deploy a Micronaut application to AWS Lambda Java 11 Runtime
Learn how to distribute a Micronaut application to AWS Lambda 11 Runtime
Authors: Sergio del Amo
Micronaut Version: 2.0.1
1 Getting Started
Please, read about Micronaut AWS Lambda Support to learn more about different Lambda runtime, Triggers and Handlers and how to integrate with a Micronaut application.
In this guide, we are going to deploy a Micronaut Application to AWS Lambda. If your Lambda integrates with API Gateway via a Lambda Proxy, a Micronaut function of type Application
with the aws-lambda
feature is a good fit. Specially, when you have multiple endpoints which you wish to delegate to a single Lambda.
1.1 What you will need
To complete this guide, you will need the following:
-
Some time on your hands
-
A decent text editor or IDE
-
JDK 1.8 or greater installed with
JAVA_HOME
configured appropriately
You will need also an AWS Account.
1.2 Solution
We recommend you to follow the instructions in the next sections and create the app step by step. However, you can go right to the completed example.
-
Download and unzip the source
or
-
Clone the Git repository:
git clone https://github.com/micronaut-guides/mn-application-aws-lambda-java11.git
Then, cd
into the complete
folder which you will find in the root project of the downloaded/cloned project.
2 Writing the App
Create a micronaut application with the aws-lambda
feature using the CLI:
% mn create-app example.micronaut.complete --features=aws-lambda
or use Micronaut Launch
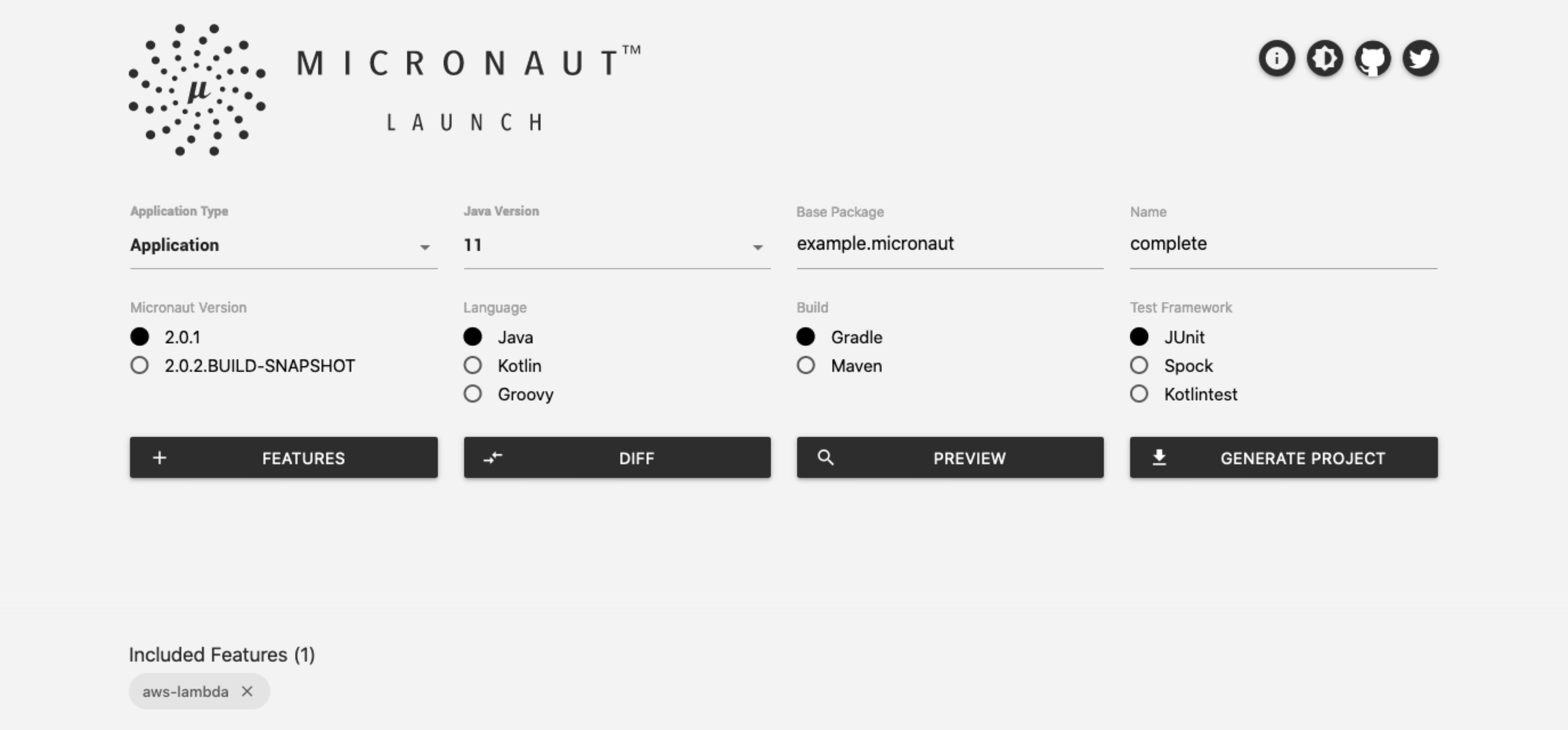
2.1 Code
The generated application contains a BookController
. It responds to POST request to /
.
package example.micronaut;
import io.micronaut.http.annotation.Body;
import io.micronaut.http.annotation.Controller;
import io.micronaut.http.annotation.Post;
import javax.validation.Valid;
import java.util.UUID;
@Controller (1)
public class BookController {
@Post (2)
public BookSaved save(@Valid @Body Book book) { (3)
BookSaved bookSaved = new BookSaved();
bookSaved.setName(book.getName());
bookSaved.setIsbn(UUID.randomUUID().toString());
return bookSaved;
}
}
1 | The class is defined as a controller with the @Controller annotation mapped to the path / |
2 | The @Post annotation is used to map HTTP request to / to the the save method. |
3 | Add the @Valid annotation to any method parameter’s object which requires validation. |
The controller’s method parameter is a Book
object:
package example.micronaut;
import edu.umd.cs.findbugs.annotations.NonNull;
import io.micronaut.core.annotation.Introspected;
import javax.validation.constraints.NotBlank;
@Introspected (1)
public class Book {
@NotBlank (2)
@NonNull (3)
private String name;
public Book() {
}
@NonNull
public String getName() {
return name;
}
public void setName(@NonNull String name) {
this.name = name;
}
}
1 | Annotate the class with @Introspected to generate the Bean Metainformation at compile time. |
2 | name is required |
3 | Add a nullability annotation to help with Kotlin interoperability and help the IDE. |
It returns a BookSaved
object:
package example.micronaut;
import edu.umd.cs.findbugs.annotations.NonNull;
import io.micronaut.core.annotation.Introspected;
import javax.validation.constraints.NotBlank;
@Introspected (1)
public class BookSaved {
@NotBlank (2)
@NonNull (3)
private String name;
@NotBlank (2)
@NonNull (3)
private String isbn;
public BookSaved() {
}
@NonNull
public String getName() {
return name;
}
public void setName(@NonNull String name) {
this.name = name;
}
@NonNull
public String getIsbn() {
return isbn;
}
public void setIsbn(@NonNull String isbn) {
this.isbn = isbn;
}
}
1 | Annotate the class with @Introspected to generate the Bean Metainformation at compile time. |
2 | name and isbn are required |
3 | Add a nullability annotation to help with Kotlin interoperability and help the IDE. |
The generated tests illustrates how the code works when the lambda gets invoked:
package example.micronaut;
import com.amazonaws.serverless.exceptions.ContainerInitializationException;
import com.amazonaws.serverless.proxy.internal.testutils.AwsProxyRequestBuilder;
import com.amazonaws.serverless.proxy.internal.testutils.MockLambdaContext;
import com.amazonaws.serverless.proxy.model.AwsProxyRequest;
import com.amazonaws.serverless.proxy.model.AwsProxyResponse;
import com.amazonaws.services.lambda.runtime.Context;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.ObjectMapper;
import io.micronaut.http.HttpHeaders;
import io.micronaut.http.HttpMethod;
import io.micronaut.http.HttpStatus;
import io.micronaut.http.MediaType;
import org.junit.jupiter.api.AfterAll;
import org.junit.jupiter.api.Assertions;
import org.junit.jupiter.api.BeforeAll;
import org.junit.jupiter.api.Test;
import io.micronaut.function.aws.proxy.MicronautLambdaHandler;
public class BookControllerTest {
private static MicronautLambdaHandler handler;
private static Context lambdaContext = new MockLambdaContext();
private static ObjectMapper objectMapper;
@BeforeAll
public static void setupSpec() {
try {
handler = new MicronautLambdaHandler(); (1)
objectMapper = handler.getApplicationContext().getBean(ObjectMapper.class);
} catch (ContainerInitializationException e) {
e.printStackTrace();
}
}
@AfterAll
public static void cleanupSpec() {
handler.getApplicationContext().close(); (2)
}
@Test
void testSaveBook() throws JsonProcessingException {
Book book = new Book();
book.setName("Building Microservices");
String json = objectMapper.writeValueAsString(book);
AwsProxyRequest request = new AwsProxyRequestBuilder("/", HttpMethod.POST.toString())
.header(HttpHeaders.CONTENT_TYPE, MediaType.APPLICATION_JSON)
.body(json)
.build();
AwsProxyResponse response = handler.handleRequest(request, lambdaContext); (3)
Assertions.assertEquals(HttpStatus.OK.getCode(), response.getStatusCode());
BookSaved bookSaved = objectMapper.readValue(response.getBody(), BookSaved.class);
Assertions.assertEquals(bookSaved.getName(), book.getName());
Assertions.assertNotNull(bookSaved.getIsbn());
}
}
1 | When you instantiate the Handler, the application context starts. |
2 | Remember to close your application context when you end your test. You can use your handler to obtain it. |
3 | You don’t invoke the controller directly. Instead, your handler receives a AWS Proxy Request event which it is routed transparently to your controller. |
3 Lambda
3.1 Create Function
Create a Lambda Function. As a runtime, select Java 11 (Correto).
3.2 Upload Code
Generate a FAT JAR of your app with ./gradlew shadowJar
and upload it.
3.3 Handler
As Handler, set:
io.micronaut.function.aws.proxy.MicronautLambdaHandler
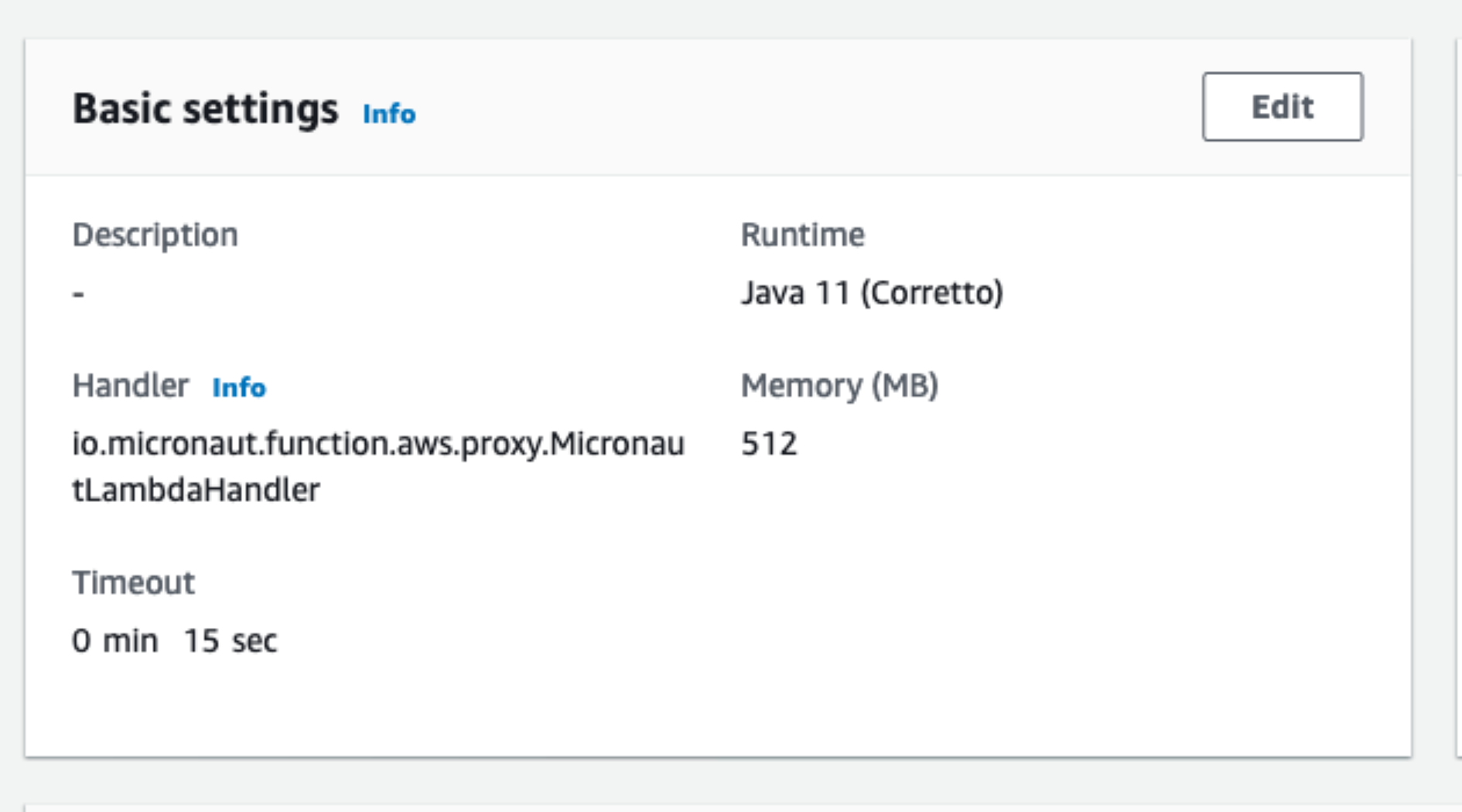
3.4 Test
You can test it easily. As Event Template
use apigateway-aws-proxy
to get you started:
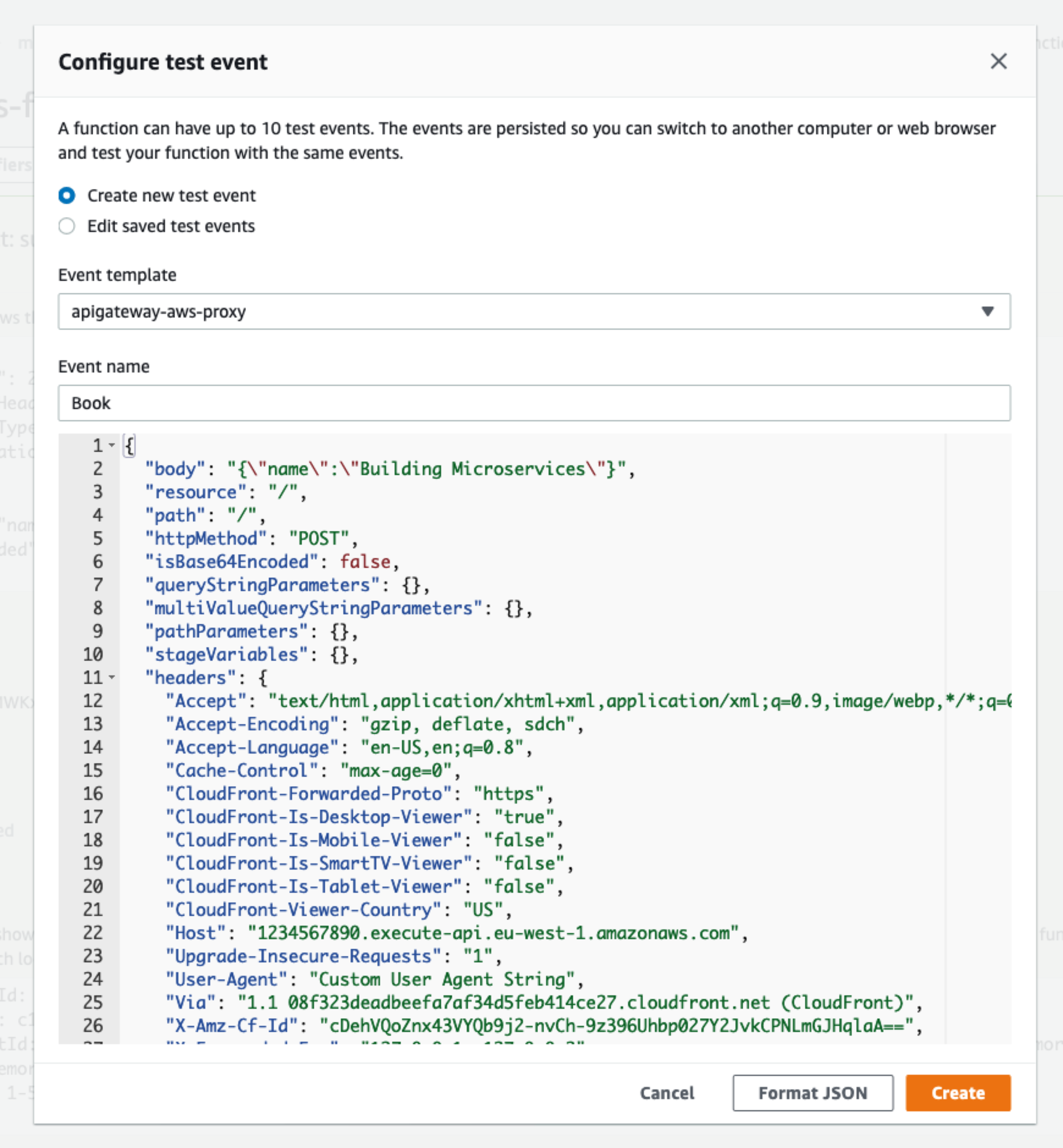
You should see a 200 response:
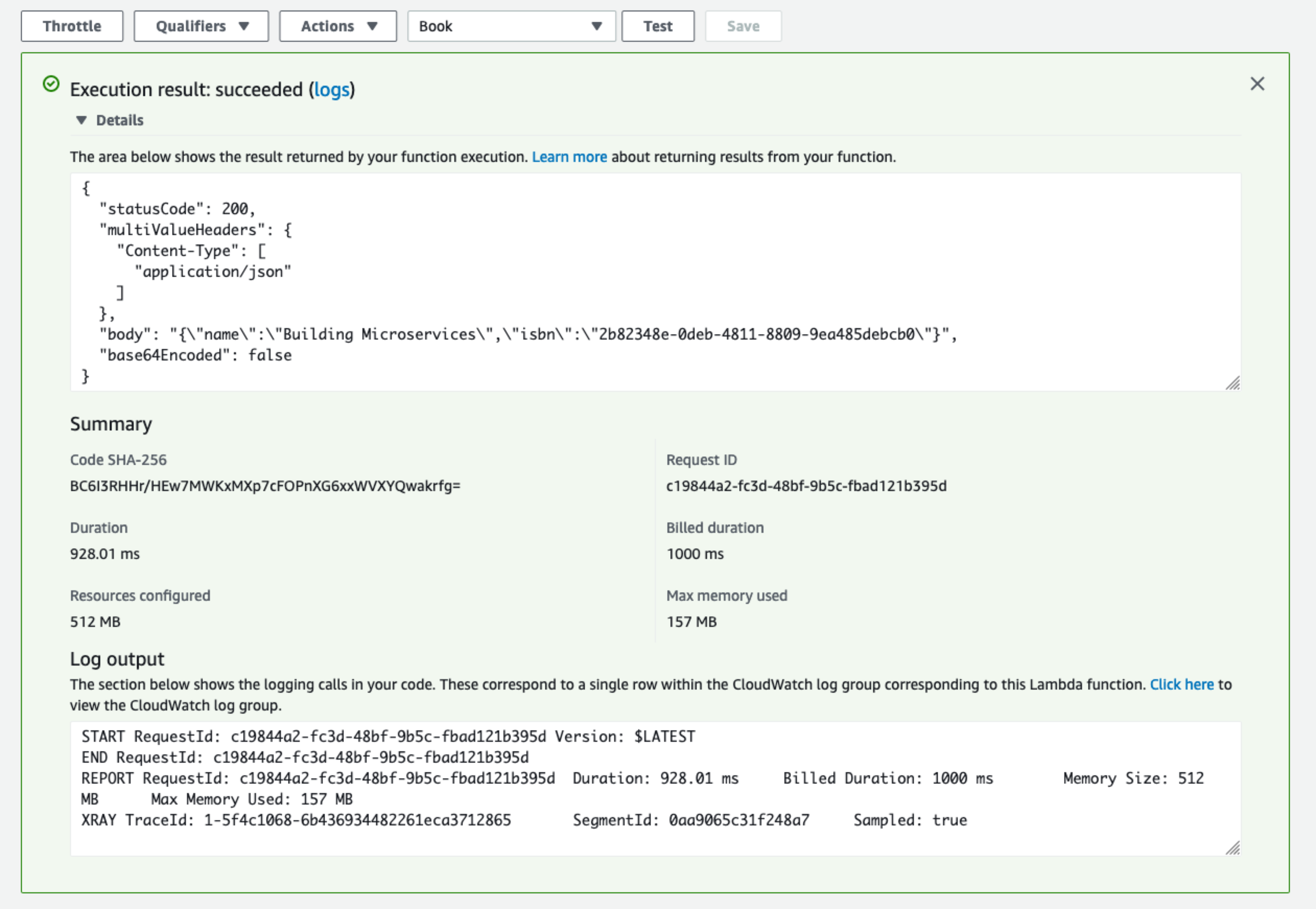
4 Next Steps
Read more about Micronaut AWS Lambda Support